Question
std::vector (available via #include ) is a template class and a C++ construct. Vector is a dynamic array that grows and shrinks dynamically as needed:
std::vector (available via #include
http://www.cplusplus.com/reference/vector/vector/ (Links to an external site.)Links to an external site.
To overcome integral size limitation (INT_MAX, DOUBLE_MAX, etc) we want to devise a programmatic scheme to store a number that can be VERY LARGE. To do that we have a positive number (N >= 0) whose digits are stored in a vector. For instance 123 is stored as {1, 2, 3} in the vector. 54321 is stored as {5, 4, 3, 2, 1} in the vector.
Write the following function that simulates ++N by taking in its vector representation as the function parameter. The function returns the result of ++N in its vector form:
vectorplusPlusN(vector v)
Examples:
input: {1,2}
output: {1,3}
input: {0}
output: {1}
input: {1}
output: {2}
input: {9}
output: {1,0}
input: {9,9,9,9,9,9,9,9,9,9,9,9,9,9,9,9,9,9,9,9,9,9,9,9,9,9,9,9}
output: {1,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0}
input: {9,2,3,9}
output: {9,2,4,0}
input: {9,9,9,9}
output: {1,0,0,0,0}
input: {2,9,9}
output: {3,0,0}
Constraints / Assumptions:
This problem tests your understanding of how templated dynamic array (i.e., vector) works in C++
Your inputs come straight from main(...) NOT cin, getline(...), etc., inside the above given function you have to write
N >= 0; the input vector
N can be very large, exceeding all integral limits in C++
You may use any vector member functions (http://www.cplusplus.com/reference/vector/vector/ (Links to an external site.)Links to an external site.) for this problem
You may create your local copy of vector
Your main() function isn't graded; only the above referenced function is.
Failure to follow the same exact function signature receives -1 logic point deduction
You may have your test code in main(). The following is just an example using v = {9, 9}:
int main() { vectorv {9, 9}; vector retVal = plusPlusN(v); // retVal = {1, 0, 0} // insert you own assert or other test code here return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
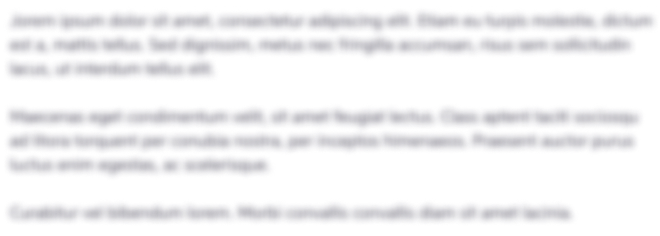
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started