Step 1: Getting Started At the beginning of each programming assignment you must have a comment block with the following information: Step 2: Declare the
Step 1: Getting Started
At the beginning of each programming assignment you must have a comment block with the following information:
Step 2: Declare the class Lab9 with the main method
In this lab we only need one class Lab9 with the main method inside.
1. /*------------------------------------------------------------- |
2. // AUTHOR: your name. |
3. // FILENAME: title of the source file. |
4. // SPECIFICATION: your own description of the program. |
5. // FOR: CSE 110- Lab #9 |
6. // TIME SPENT: how long it took you to complete the assignment. |
7. //----------------------------------------------------------- */ |
1. /*------------------------------------------------------------------------- |
2. // AUTHOR: your name. |
3. // FILENAME: title of the source file. |
4. // SPECIFICATION: your own description of the program. |
5. // FOR: CSE 110- Lab #9 |
6. // TIME SPENT: how long it took you to complete the assignment. |
7. //-----------------------------------------------------------*/ |
8. |
9. |
10. import java.util.Scanner; 11. |
12. public class Lab9 { |
13. public static void main(String[] args) { 14. 15. 16. 17. 18. 19. 20. 21. 22. 23. 24. 25. } // Declaring variables // ... // Request array size from the user // ... // Declare the array // ... // Fill in the array // ... // Construct a for loop to do summation // ... // Display the result |
Step 3: Declare some variables
To get input from the user, we need some variables to hold the data. Please declare four variables as follows, you can choose the variable names you like:
- arraySize: int
- currentElement: double
- sumOfElements: double
- scan: Scanner
Step 4: Request array size from the user
Please use the Scanner object you defined to request an integer from the user. Store the result in the variable arraySize. To do so, you will need a println to prompt the user and scan.nextInt() method to parse an integer.
Step 5: Declare the array
In Java, an array is a collection of variables. It reserves a sequence of memory space and collects data of the same type. For example, an integer array of size 5 can hold 5 integers in one place. In some cases, it we would like to keep relevant data together instead of using multiple sparse variables.
With the array size determined, now we can declare an array of double values.
1. // Request array size from user |
2. 3. // Print this message "How many elements in the array?" |
4. // --> |
5. |
6. // Request an integer from the user using the Scanner object |
7. // and store the inputted value in the integer declared above. |
8. // --> |
1. // Declare the array |
2. |
3. |
4. // Declare a new array of size equal to the size requested |
5. // --> |
6. |
7. // For reference, the following is an EXAMPLE declaration of an |
// integer array of a fixed size. DO NOT USE THIS ARRAY. // // int[] integerArray = new int[25]; |
The above example shows you how to declare an integer array. Your array must be double array.
Step 6: Fill in the array
An array element can be accessed by using array index, which is the equivalent idea to the String index. To assign a value to our array, we may do
doubleArray[0] = 10.0; It means we put the value 10.0 to the first element of doubleArray;
To initialize all elements in an array, we can utilize for loop and make the loop variable iterate through available indices.
Please write a for loop with a variable goes from 0 to arraySize 1. In each round, you need to use Scanner object to get a new value for the array element.
1. //for(int i = 0; i < |
2. |
3. // Display the message: "Please enter the next value." |
4. // --> |
5. |
6. // Request the next element (double) from the user using |
7. // the Scanner object. |
8. // --> |
9. |
10. // Store this element at the ith position of the array |
11. // --> |
12. //} |
Step 7: Display and sum the elements in the array
To display and sum elements in an array, again we will use a for loop. Please write a for loop which can iterate all elements in your array and find the sum.
1. // Display and sum the array's elements |
2. |
3. // Construct a for loop that runs backwards through the array, |
4. // starting at the last element and ending at the first. |
5. |
6. // for (/* Loop logic */) { |
7. |
8. // Inside this for loop, print the ith element of the array |
9. // and a tab, with NO newline characters. |
10. // --> |
11. |
12. // If this element is the 8th one on its line, print a |
13. // newline character to advance to the next line. |
14. // Also inside this for loop, add the value of the ith |
15. // element to the current value of the double for the sum |
16. // of elements. |
17. // --> |
18. |
19. //} |
Step 8: Display the result
Finally, after the last for loop, you need to print the sum of all elements in the array. Please check Sample Output below for details.
1. //Display the sum |
2. |
3. // Print the following message to the user, preceded by a |
4. // newline character. |
5. // "The sum of the array's elements is: |
6. // --> |
Sample Output
Below is an example of what your output should roughly look like when this lab is completed. Text in RED represents user input.
How many elements in the array?
10
Please enter the next value:
0
Please enter the next value:
1
Please enter the next value:
2
Please enter the next value:
3
Please enter the next value:
4
Please enter the next value:
5
Please enter the next value:
6
Please enter the next value:
7
Please enter the next value:
8
Please enter the next value:
9
0.0 1.0 2.0 3.0 4.0 5.0 6.0 7.0 8.0 9.0 The sum of the array's elements is: 45.0
Step by Step Solution
There are 3 Steps involved in it
Step: 1
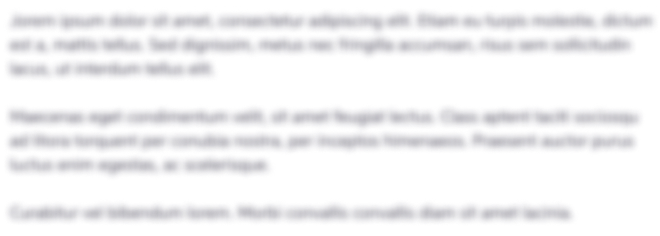
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started