Question
Step 1: Provide a circular array implementation of a queue (implements ItemQueue.java). Your implementation must accommodate reallocation of the array as needed. Define your array
Step 1:
Provide a circular array implementation of a queue (implements ItemQueue.java). Your implementation must accommodate reallocation of the array as needed. Define your array to initially be a size of 4.
Make your queue implementation is thread safe.
Step 2:
Write a program to thoroughly test all of the methods of your ItemQueue class. Once you are confident is it working correctly, proceed to part III. Make sure to test the resizing of the array thoroughly test your implementation. Be sure to test the reallocation with tail < head and when tail > head.
ItemQueue.java:
/**
* An abstract data type for a Queue. Specifies the 5
* main methods of a queue
*
* @author
*/
public interface ItemQueue
/**
* Enqueue an object to the end of the queue
* @param item the object to be enqueued
*/
public void enqueueItem(E item);
/**
* Dequeue an object from the front of the queue
* @return the item removed from the front of the queue
* @throws NoSuchElementException if the queue is empty
*/
public E dequeueItem();
/**
* Examine the object at the head of the queue, but do
* not remove it.
* @return the item at the head of the queue
* @throws NoSuchElementException if the queue is empty
*/
public E peekItem();
/**
* Test if the queue is empty
* @return true if the queue is empty, otherwise false
*/
public boolean isEmpty();
/**
* Get the number of items in the queue
*
* @return the number of items in the queue
*/
public int noItems();
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
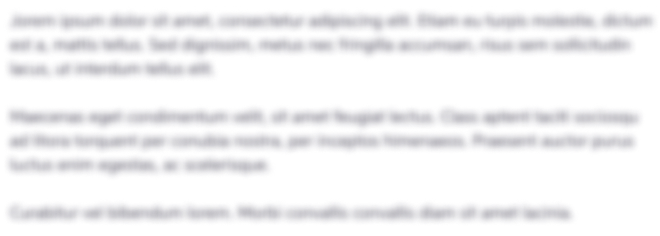
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started