Question
Store a table (rows and columns) of data (a DataFrame) and perform some basic statistical operations and sub-setting on the data. Users can load a
Store a table (rows and columns) of data (a DataFrame) and perform some basic statistical operations and sub-setting on the data. Users can load a DataFrame using a CSV (comma-separated values) file and also save a manipulated (subset) DataFrame in a new CSV.
DataFrame - Has a collection of column headers, a collection of data types for each column (int double char String), and a collection of data rows (max size 25,000 rows). You will need to use polymorphism to be able to store data of different types in the same collection of rows. Any errors in DataFrame methods should throw an exception, and when caught the details of the exception should be written to a log.txt file. Other than the method to write out the active DataFrame to a CSV file (using the name of the DataFrame as the name of the CSV file), the DataFrame class should not do any file reading or writing. Instead the DataFrameMenu should handle all other file reading/writing.
DataFrameMenu - The user interface (void main) to allow a user to create and analyze DataFrames (up to 10 loaded at a time). Any exceptions thrown from a DataFrame should be handled and logged in a log.txt file. The active DataFrame is the one that commands execute on. This functionality is required:
- import a valid CSV, create a DataFrame, and make it the active DataFrame
- change the active DataFrame to one loaded already
- average a column in the active DataFrame, only works on numeric columns, given a column name for numeric data, return the average of the values in the column (if any error in method, return Double.NaN)
- find the minimum of a column in the active DataFrame, only works on numeric columns, given a column name for numeric data, return the minimum of the values in the column (if any error in method, return Double.POSITIVE_INFINITY)
- find the maximum of a column in the active DataFrame, only works on numeric columns, given a column name for numeric data, return the maximum of the values in the column (if any error in method, return Double.NEGATIVE_INFINITY)
- frequency table (5 equally sized intervals) of a column in the active DataFrame, only works on numeric columns (if any error in method, throw an exception)
- subset a DataFrame - create a new DataFrame (and make it the new active one) from the current active DataFrame by selecting rows where "columnData operator value" boolean statement is true for the data in a column. The allowed operators are < == > != (if any error in method, throw an exception). Name the new DataFrame using the current DataFrame name along with the boolean statement.
- export the active DataFrame to a CSV file named with the same name as the DataFrame. Include column names and data types in the first two rows.
- quit
Here are two sample CSV files you can test with. Note the first row are the column names and the 2nd row are the datatypes.
- youth fitness data -PEdata-multiYear.csv
- bikeshare data -Divvy_Trips_July2013.csv
Each non "void main" class needs its own individual test program. Each non "void main" class needs its own individual exception class and should throw exceptions for any invalid action. Other classes need to catch these exceptions where appropriate and write out details to log.txt.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
Answer Below is a basic outline of the classes with minimal implementation Youll need to fill in the details and add error handling logging and other ...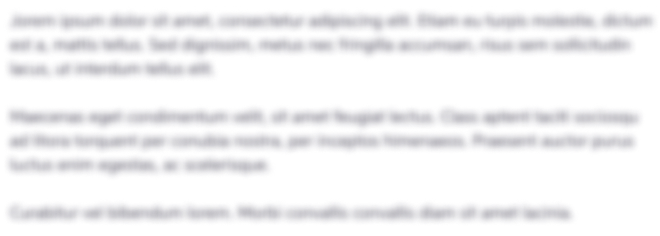
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started