Question
StringArray Ehnancements Modify the StringArray class that was created in class to include three additional functions: insert(index, s) This function should expand the size of
StringArray Ehnancements
Modify the StringArray class that was created in class to include three additional functions:
insert(index, s) This function should expand the size of the array by one, inserting the given string at the given index position. All prior values from position index through the end of the array are to be shifted up to the next position.
insert(index, n_elements) This function should insert n_elements empty strings beginning at position index. The size of the array increases by n_elements.
remove(index, n_elements) This function should remove n_elements elements from the array beginning at position index. Elements beyond those removed are to be shifted down accordingly. The size of the array decreases by n_elements.
Note that you will need to ensure that there is sufficient capacity for the insert functions. There is no need to consider capacity for the remove function.
The supplied stringarray.cpp file is the source code that was developed during class. The supplied stringarray.h file is modified from the class version which includes comments for all public member functions, and also includes prototypes for the functions to be added.
A main function is provided that generates 15 random strings and inserts them into a StringArray object. Add code to the main function that demonstrates your three added functions work as expected.
stringarray.cpp
#include "stringarray.h" | |
using namespace std; | |
StringArray::StringArray () { | |
array_capacity = 10; | |
array_size = 0; | |
p_array = new string[array_capacity]; | |
} | |
void StringArray::append(const string& s) { | |
if (array_size == array_capacity) | |
expand_capacity(); | |
p_array[array_size++] = s; | |
} | |
void StringArray::expand_capacity(int new_capacity) { | |
if (new_capacity == 0) | |
new_capacity = array_capacity + array_capacity / 2; | |
if (new_capacity <= array_capacity) | |
return; | |
// Allocate a new array | |
string *p = new string[new_capacity]; | |
// Copy old array to new array | |
for (int i = 0; i < array_capacity; i++) | |
p[i] = p_array[i]; | |
// Deallocate old array (return to system free memory) | |
delete [] p_array; | |
// Set up pointer to new array, and set capacity | |
p_array = p; | |
array_capacity = new_capacity; | |
} | |
string StringArray::get(int index) const { | |
if (index < 0 || index >= array_size) | |
throw "Index out of range"; | |
return p_array[index]; | |
} | |
void StringArray::resize(int new_size) { | |
if (new_size < 0) | |
throw "Invalid array resize"; | |
expand_capacity(new_size); | |
array_size = new_size; | |
} | |
void StringArray::set(int index, const string& s) { | |
if (index < 0 || index >= array_size) | |
throw "Index out of range"; | |
p_array[index] = s; | |
} |
stringarray.h
#pragma once | |
#include | |
/** | |
* A Dynamic Array of String objects | |
*/ | |
class StringArray { | |
private: | |
std::string *p_array; | |
int array_capacity; | |
int array_size; | |
void expand_capacity(int new_capacity=0); | |
public: | |
/** | |
* Default Constructor | |
* | |
* Creates an empty array of string objects. | |
*/ | |
StringArray(); | |
/** | |
* Construct StringArray of given size | |
* | |
* Creates an array of string objects of a pre-defined size. Each element | |
* created is an empty string. | |
*/ | |
StringArray(int initial_size) : StringArray() { resize(initial_size); } | |
/** | |
* Destructor, frees memory used by the array | |
*/ | |
virtual ~StringArray() { delete [] p_array; } | |
/** | |
* Add an element to the end of the array | |
* | |
* Expands the size of the array by one and sets the element to the | |
* value of the given string. | |
* | |
* @param s String to be added | |
*/ | |
void append(const std::string& s); | |
/** | |
* Retrieve value of string by its index | |
* @param index Index of the string to be returned | |
* @return Value at the given index | |
*/ | |
std::string get(int index) const; | |
void insert(int index, const std::string& s); | |
void insert(int index, int n_elements=1); | |
void remove(int index, int n_elements=1); | |
/** | |
* Change the size of the array | |
* | |
* Changes the size of the array. If new size is larger than the current | |
* size, value in the newly created elements is unpredictable. If new size | |
* is smaller, element values beyond the new size are discarded. | |
* | |
* @param new_size Desired size of the array | |
*/ | |
void resize(int new_size); | |
/** | |
* Set value at given index | |
* @param index Index of the element to be set | |
* @param s New value to be assigned at the given index | |
*/ | |
void set(int index, const std::string& s); | |
/** | |
* Get size of the array | |
* @return Number of elements in the array | |
*/ | |
int size() const { return array_size; } | |
}; |
Step by Step Solution
There are 3 Steps involved in it
Step: 1
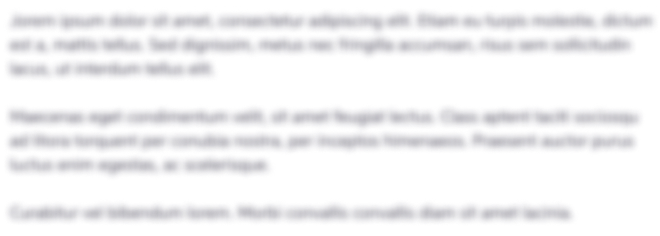
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started