Answered step by step
Verified Expert Solution
Question
1 Approved Answer
struct HuffNode { short value; / / ASCII value of the node, or - 1 if not a leaf node unsigned int freq; / /
struct HuffNode short value;ASCII value of the node, or if not a leaf node unsigned int freq;Number of times this symbol occurs in the file HuffNode left;Pointer to the left child HuffNode right;Pointer to the right child HuffNode parent;Pointer to the parent HuffNodeshort value, unsigned int freq, HuffNodeleft nullptr, HuffNoderight nullptr, HuffNodeparent nullptr : valuevalue freqfreq leftleft rightright parentparent HuffNodeconst HuffNode& hnode : valuehnode.value freqhnode.freq lefthnode.left righthnode.right parenthnode.parent struct Huff compare bool operatorconst HuffNodea const HuffNodeb return afreq bfreq; ; Data Members std::string mFilename ; Stores the name of the file could be input or output, depending on the method unsigned int mFrequencyTable; Array of unsigned values to store how often any ASCII symbol appears in an uncompressed file std::vector mLeafList; std::vector that stores the address of all leaf nodes HuffNode mRoot reinterpretcast; Pointer to the top of the tree std::vector mEncodingTable; Array of vectors containing bools. Each index of this will store the bitcode for an individual ASCII value Methods The HuffNode struct is already implemented. You will use the constructor when creating Nodes in several places. The HuffCompare struct is also fully implemented, and will be used in the GenerateTree method. Huffman Constructor Huffmanconst std::string& filename Assign the appropriate data member the value from the parameter Zero out the entire frequency table Set the root to a value that indicates the tree is currently empty GenerateFrequencyTable void GenerateFrequencyTable Open the file in binary mode, using a std::ifstream Read the file one byte at a time, and increment the corresponding index o The indices of the frequency table line up with the ASCII values Close the file when complete GenerateLeafList void GenerateLeafList Iterate through the frequency table and dynamically create a leaf node for each non frequency Add each node to the mLeafList vector GenerateTree void GenerateTree Create the priorityqueue o This will be storing HuffNode in a vector, and uses HuffCompare for the comparator Populate the priorityqueue with the data in the leaf list Generate the tree with the following algorithm o While the queue has more than node Store the top two nodes into some temporary pointers and pop them Create a new parent node with first node as the left child, and second node as the right child Set the parents value to and the frequency to the sum of its two childrens frequencies Set the first and second nodes parent to the newly created node Insert the new node into the queue Set the root data member o There is only one node in the queue ClearTree void ClearTree Perform a postorder traversal to delete all of the nodes Same as Clear from the BST Destructor ~Huffman Clean up all of the dynamically allocated memory Theres a method to help with this GenerateEncodingTable void GenerateEncodingTable Go through all of the leaf nodes and generate the bit codes This is done by traversing up the tree from each leaf node with a temporary pointer, and storing the direction in the corresponding vector o Each index of the encoding table aligns to an ASCII value As you move up push a to the vector if you passed through a left child connection, and a if you passed through a right connection Once you hit the root node, reverse the values in the vector Compress void Compressconst charoutputFile In this method, mFileName is the file to compress, and the parameter is the name of the file to write to Create the frequency table, leaf list, tree, and encoding table by calling the existing methods in this order Create a BitOfstream and supply it the Huffman header Open the input file in binary mode with a std::ifstream Compress the file o For each byte in the original file, write out the corresponding bitcode from the encoding table Close both streams Decompress void Decompressconst charoutputFile In this method, mFileName is the file to decompress, and the parameter is the name of the file to write to Create a BitIfstream and read the frequency table Create the leaf list and tree by calling the existing methods in this order Create a std::ofstream for output in binary mode Create a bool to use for traversing down the tree, and an unsigned char for writing to the file Create a HuffNode pointer for use in traversing the tree start at the top Go through the compressed file one bit at a time, moving the temporary pointer down the tree o When a leaf node is reached, write the value to the uncompressed file, and go back to the root This will need to be done a number of times equal to the total frequency of the original file Close both streams
struct HuffNode
short value;ASCII value of the node, or if not a leaf node
unsigned int freq;Number of times this symbol occurs in the file
HuffNode left;Pointer to the left child
HuffNode right;Pointer to the right child
HuffNode parent;Pointer to the parent
HuffNodeshort value, unsigned int freq, HuffNodeleft nullptr, HuffNoderight nullptr, HuffNodeparent nullptr
: valuevalue freqfreq leftleft rightright parentparent
HuffNodeconst HuffNode& hnode
: valuehnode.value freqhnode.freq lefthnode.left righthnode.right parenthnode.parent
struct Huff compare
bool operatorconst HuffNodea const HuffNodeb
return afreq bfreq;
;
Data Members
std::string mFilename ; Stores the name of the file could be input or output, depending on
the method
unsigned int mFrequencyTable; Array of unsigned values to store how often any ASCII symbol
appears in an uncompressed file
std::vector mLeafList; std::vector that stores the address of all leaf nodes
HuffNode mRoot reinterpretcast; Pointer to the top of the tree
std::vector mEncodingTable; Array of vectors containing bools.
Each index of this will store the bitcode for an individual ASCII value
Methods
The HuffNode struct is already implemented. You will use the constructor when creating
Nodes in several places. The HuffCompare struct is also fully implemented, and will be used in the GenerateTree method.
Huffman Constructor
Huffmanconst std::string& filename
Assign the appropriate data member the value from the parameter
Zero out the entire frequency table
Set the root to a value that indicates the tree is currently empty
GenerateFrequencyTable
void GenerateFrequencyTable
Open the file in binary mode, using a std::ifstream
Read the file one byte at a time, and increment the corresponding index
o The indices of the frequency table line up with the ASCII values
Close the file when complete
GenerateLeafList
void GenerateLeafList
Iterate through the frequency table and dynamically create a leaf node for each non
frequency
Add each node to the mLeafList vector
GenerateTree
void GenerateTree
Create the priorityqueue
o This will be storing HuffNode in a vector, and uses HuffCompare for the
comparator
Populate the priorityqueue with the data in the leaf list
Generate the tree with the following algorithm
o While the queue has more than node
Store the top two nodes into some temporary pointers and pop them
Create a new parent node with first node as the left child, and second
node as the right child
Set the parents value to and the frequency to the sum of its two
childrens frequencies
Set the first and second nodes parent to the newly created node
Insert the new node into the queue
Set the root data member
o There is only one node in the queue
ClearTree
void ClearTree
Perform a postorder traversal to delete all of the nodes
Same as Clear from the BST
Destructor
~Huffman
Clean up all of the dynamically allocated memory Theres a method to help with this
GenerateEncodingTable
void GenerateEncodingTable
Go through all of the leaf nodes and generate the bit codes
This is done by traversing up the tree from each leaf node with a temporary pointer, and storing the direction in the corresponding vector
o Each index of the encoding table aligns to an ASCII value
As you move up push a to the vector if you passed through a left child connection, and a if you passed through a right connection
Once you hit the root node, reverse the values in the vector
Compress
void Compressconst charoutputFile
In this method, mFileName is the file to compress, and the parameter is the name of the file to
write to
Create the frequency table, leaf list, tree, and encoding table by calling the existing
methods in this order
Create a BitOfstream and supply it the Huffman header
Open the input file in binary mode with a std::ifstream
Compress the file
o For each byte in the original file, write out the corresponding bitcode from the
encoding table
Close both streams
Decompress
void Decompressconst charoutputFile
In this method, mFileName is the file to decompress, and the parameter is the name of the file
to write to
Create a BitIfstream and read the frequency table
Create the leaf list and tree by calling the existing methods in this order
Create a std::ofstream for output in binary mode
Create a bool to use for traversing down the tree, and an unsigned char for writing to the file
Create a HuffNode pointer for use in traversing the tree start at the top
Go through the compressed file one bit at a time, moving the temporary pointer down the tree
o When a leaf node is reached, write the value to the uncompressed file, and go
back to the root
This will need to be done a number of times equal to the total frequency
of the original file
Close both streams
Step by Step Solution
There are 3 Steps involved in it
Step: 1
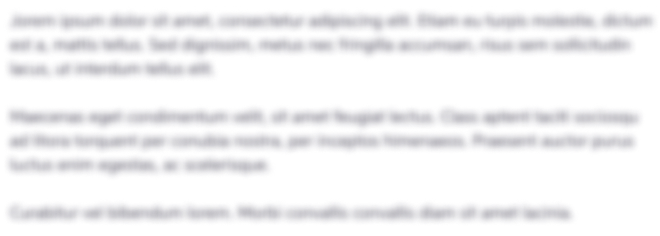
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started