Question
Super Bowl The following data for super bowl games is stored in a file named superbowls.txt I 1/15/1967 Green Bay Packers 35-10 Kansas City Chiefs
Super Bowl
The following data for super bowl games is stored in a file named superbowls.txt
I
1/15/1967
Green Bay Packers
35-10
Kansas City Chiefs
Los Angeles Memorial Coliseum
II
1/14/1968
Green Bay Packers
33-14
Oakland Raiders
Miami Orange Bowl
III
1/12/1969
New York Jets
16-7
Baltimore Colts
Miami Orange Bowl
Write a program that will process this file, reading the file name from the user, and then creating a linked list with records containing the following information, ordered by game number.
struct GameDate
{
int month;
int day;
int year;
}
struct SuperBowlNode { char game_number[NUMBER_SIZE];
char win_team[TEAM_NAME_SIZE];
int win_score;
char lose_team[TEAM_NAME_SIZE];
int lose_score;
char location[CITY_NAME_SIZE];
GameDate date;
SuperBowlNode * next;
};
After processing the file, your program should display a menu that allows the user to choose one of the following options:
- Print Complete Game List in Reverse
- Print Games for a Team
- Print Total Points Scored by a Team
- Exit
Each option is explained below:
- Print Complete Game List: Displays information for all games in a table with the most recent game first. You MUST use a RECURSIVE function for this option.
- Print Games for a Team: Reads a team name from the user and displays information for all games that that team played in, ordered by year (game number).
- Print Total Points Scored by a Team: Reads a team name from the user and displays the sum of the points scored by that team in all super bowl games that the team played in.
- Exit: Exits the program
Your program must continue to display the menu and process selections as stated above until the user chooses to exit the program.
Write a class named GameList that contains a linked list of the SuperBowlNode structures given above. Your class should include a constructor and destructor along with methods to read a file, append a node, display the list in reverse (recursively), display games for a team, and display total points scored for a team.
Store your class declarations and member functions in separate files named with the class name and the appropriate extension (.h or .cpp).
Put your main program in a separate file named sbowl_program.cpp.
I have the .h file completed. I am not sure how to make the readfile function. Any help is much appreciated.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
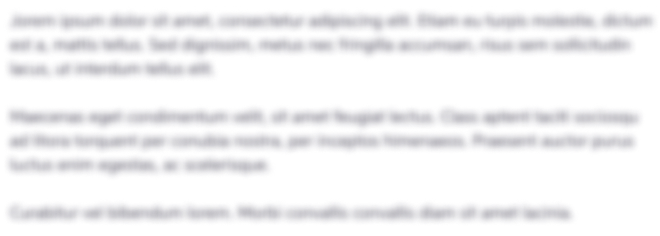
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started