Question
Suppose that we want to help physicians to diagnose illnesses. A physician observes a patient's symptoms and considers the illnesses that could be associated with
Suppose that we want to help physicians to diagnose illnesses. A physician observes a patient's symptoms and considers the illnesses that could be associated with those symptoms. Design and implement a class PhysiciansHelper that provide a list of those illnesses.
PhysiciansHelper should contain a dictionary of illnesses and symptoms. A method should read a text file of illnesses and their symptoms into the dictionary. See format in sample data file.
PhysiciansHelper should read a list of symptoms for a patient. A method should read a list of symptoms and store into a list. Then, it finds a list illnesses that are associated with those symptoms. See format in sample run output.
Note: I have modified specification and requirements of this project
Project requirements:
? You will need to complete two methods in PhysiciansHelper class:
public void processDatafile();
public void processSymptoms();
Refer to PhysiciansHelper for detailed info.
? You must use the predefined private Map> symptomChecker to store symptoms to illnesses mapping info. See format in sample run output.
? All string data are not case sensitive, i.e. should work for either uppercase or lowercase letters.
? In processSymptoms() methods, you must
o list possible symptoms in sorted order.
o read user input symptoms in single line
o check for invalid symptoms and eliminate duplicated symptoms
o display result in format as shown in sample output (must count number of matched symptoms)
? Use Java API Map and List in your project
https://docs.oracle.com/javase/8/docs/api/java/util/Map.html
https://docs.oracle.com/javase/8/docs/api/java/util/List.html
Here are some methods that you may need:
Map: get(), put(), containsKey(), keySet(), entrySet()
List: add(), contains(), size()
Compile program: javac PhysiciansHelper.java
Sample Run: Note: I expect similar output from your program
$ cat sample_data.txt // contents of sample data file
cold: runny nose, cough, sore throat
flu: runny nose, cough, sore throat, muscle aches, fever
mumps: high fever, rash, swelling
whooping cough: cough, rash, high fever
acid reflux: chest pain, burping
chickenpox: fever, blister
measles : runny nose, cough, fever, rash
norovirus : nausea, diarrhea, vomiting
ague: sweatiness, achy, high fever
$ javac PhysiciansHelper.java
$ java PhysiciansHelper
Enter data filename: sample_data.txt
============================================
symptomChecker map: // display contents of map
achy=[ague]
blister=[chickenpox]
burping=[acid reflux]
chest pain=[acid reflux]
cough=[cold, flu, whooping cough, measles]
diarrhea=[norovirus]
fever=[flu, chickenpox, measles]
high fever=[mumps, whooping cough, ague]
muscle aches=[flu]
nausea=[norovirus]
rash=[mumps, whooping cough, measles]
runny nose=[cold, flu, measles]
sore throat=[cold, flu]
sweatiness=[ague]
swelling=[mumps]
vomiting=[norovirus]
============================================
The possible symptoms are: // display symptoms in sorted order
achy
blister
burping
chest pain
cough
diarrhea
fever
high fever
muscle aches
nausea
rash
runny nose
sore throat
sweatiness
swelling
vomiting
============================================
Enter symptoms: cough,rash,cough,fever,blisterx,cough,runny NOSE
=>duplicate symptom:cough
=>invalid symptom:blisterx
=>duplicate symptom:cough
============================================
PatientSymptoms list: [cough, rash, fever, runny nose]
Possible illnesses that match any symptom are:
==> Disease(s) match 1 symptom(s)
chickenpox
mumps
==> Disease(s) match 2 symptom(s)
whooping cough
cold
==> Disease(s) match 3 symptom(s)
flu
==> Disease(s) match 4 symptom(s)
measles
||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
sample_data.txt
cold: runny nose, cough, sore throat
flu: runny nose, cough, sore throat, muscle aches, fever
mumps: high fever, rash, swelling
whooping cough: cough, rash, high fever
acid reflux: chest pain, burping
chickenpox: fever, blister
measles : runny nose, cough, fever, rash
norovirus : nausea, diarrhoea, vomiting
ague: sweatiness, achy, high fever
||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
PhysiciansHelper.java
import java.util.*;
import java.io.*;
public class PhysiciansHelper
{
// symptom to illnesses map
private Map> symptomChecker;
/* Constructor symptomChecker map using TreeMap */
public PhysiciansHelper()
{
// use TreeMap, i.e. sorted order keys
symptomChecker = new TreeMap>();
} // end default constructor
/* Reads a text file of illnesses and their symptoms.
Each line in the file has the form
Illness: Symptom, Symptom, Symptom, ...
Store data into symptomChecker map */
public void processDatafile()
{
// Step 1: read in a data filename from keybaord
// create a scanner for the file
// Step 2: process data lines in file scanner
// 2.1 for each line, split the line into a disease and symptoms
// make sure to trim() spaces and use toLowercase()
// 2.2 for symptoms, split into individual symptom
// create a scanner for symptoms
// useDelimeter(",") to split into individual symptoms
// make sure to trim() spaces and use toLowercase()
// for each symptom
// if it is already in the map, insert disease into related list
// if it is not already in the map, create a new list with the disease
// Step 3: display symptomChecker map
// implement here.....
} // end processDatafile
/* Read patient's symptoms in a line and adds them to the list.
Input format: Symptom, Symptom, Symptom,...
Shows diseases that match a given number of the symptoms. */
public void processSymptoms()
{
// Step 1: get all possible symptoms from assciatedIllnesses map
// and display them
// Step 2: process patient symptoms, add to patientSymptoms list
// read patient's symptoms in a line, separated by ','
// create a scanner for symptoms
// UseDelimeter(",") to split into individual symptoms
// make sure to trim() spaces and use toLowercase()
// display invalid/duplicate symptoms
// add valid symptoms to patientSymptoms list
// Step 3: display patientSymptoms list
// Step 4: process illnesses to frequency count
// create a map of disease name and frequency count
// for each symptom in patientSymptoms list
// get list of illnesses from symptomChecker map
// for each illness in the list
// if it is already in the map, increase counter by 1
// if it is not already in the map, create a new counter 1
// ** need to keep track of maximum counter numbers
// Step 5: display result
// for count i = 1 to maximum counter number
// display illness that has count i
// implement here.....
} // end processSymptoms
public static void main(String[] args)
{
PhysiciansHelper lookup = new PhysiciansHelper();
lookup.processDatafile();
lookup.processSymptoms();
} // end main
} // end PhysiciansHelper
Step by Step Solution
3.27 Rating (156 Votes )
There are 3 Steps involved in it
Step: 1
Solution Code code starts here import javautil import javaio public class PhysiciansHelper symptom to illnesses map private Map symptomChecker Constructor symptomChecker map using TreeMap public Physi...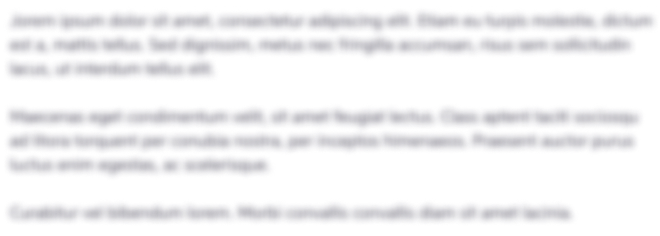
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Document Format ( 2 attachments)
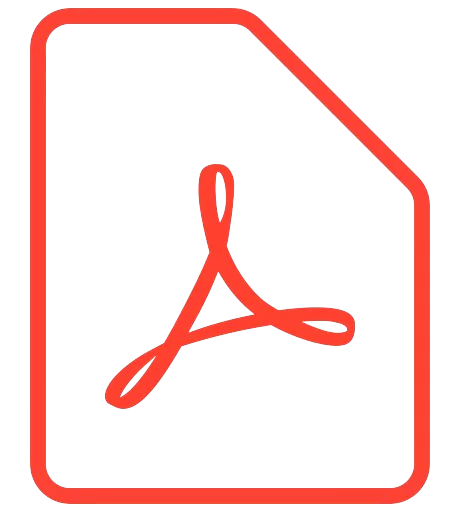
635d8150361f4_176250.pdf
180 KBs PDF File
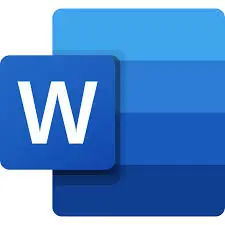
635d8150361f4_176250.docx
120 KBs Word File
Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started