Answered step by step
Verified Expert Solution
Question
1 Approved Answer
SymbolTable.java - package edu.ser 2 2 2 . m 0 3 _ 0 2 ; / * * * Symbol table interface. * * @author
SymbolTable.java
package edu.serm;
Symbol table interface.
@author Sedgewick, Acuna
@param search key
@param return type
public interface SymbolTable
put keyvalue pair into the table
void putKey key, Value val;
get value paired with key. returns null if key does not exist.
Value getKey key;
remove key and its value from table
void deleteKey key;
is there a value paired with key?
boolean containsKey key;
is the table empty?
boolean isEmpty;
number of keyvalue pairs
int size;
all keys in the table
Iterable keys;
OrderedSymbolTable.java
package edu.serm;
Ordered symbol table interface.
@author Sedgewick, Acuna
@param search key
@param return type
public interface OrderedSymbolTable extends SymbolTable
Returns the minimum key.
@throws NoSuchElementException if the ST is empty
@return minimum key.
Key min;
Returns the maximum key.
@throws NoSuchElementException if the ST is empty
@return maximum key.
Key max;
Returns largest key less than or equal to key. If no such key exists,
returns null.
@throws NoSuchElementException if the ST is empty
@param key target floor
@return closest key
Key floorKey key;
Returns smallest key greater than or equal to key. If no such key exists,
returns null.
@throws NoSuchElementException if the ST is empty
@param key target floor
@return closest key
Key ceilingKey key;
Returns number of keys less than key.
@param key target key
@return rank
int rankKey key;
Returns key of rank k
@param k target rank
@return key
Key selectint k;
Delete smallest key.
@throws NoSuchElementException if the ST is empty
void deleteMin;
Deletes largest key.
@throws NoSuchElementException if the ST is empty
void deleteMax;
Returns the number of keys in lohi
@param lo begining of range
@param hi end of range
@return number of keys
int sizeKey lo Key hi;
Returns all keys in lohi in sorted order.
@param lo begining of range
@param hi end of range
@return Iterable container of keys.
Iterable keysKey lo Key hi;
BSTjava
package edu.serm;
BST defines an interface to a BST implementation of OrderedSymbolTable that
offers methods
specific to trees.
@author Acuna
@version
@param contained key type
@param contained value type
public interface BST extends OrderedSymbolTable
Puts a key value pair into the tree. If key already exists, then only
updates value.
@param key key to add
@param val value for key
void putFastKey key, Value val;
Returns the value paired with a key in the tree. Returns null if key does
not exist.
@param key key to find
@return value of key
Value getFastKey key;
Returns a string representation of the tree. The ordering is a level
traversal of the tree,
and each node's value is separated by a space. If there is no valid subtree
rooted at the
given key, returns "empty".
@return the level order string representation of the tree
public String displayLevelKey key;
public void balance;
Returns the root node of the BST
@return the root node
public Node getRoot;
Node.java
package edu.serm;
A class to represent a node in a binary search tree.
@author Sedgewick, Acuna
@version
@param contained key type
@param contained value type
public class Node
public final Key key;
public Value val;
public Node left, right;
public int N;
public NodeKey key, Value val, int N
this.key key; this.val val; this.N N;
CompletedBST.java
package edu.serm;
A binary search tree based implementation of a symbol table.
Completion time: your completion time
@author your name Sedgewick, Acuna
@version version
import java.util.Collections;
import java.util.LinkedList;
import java.util.NoSuchElementException;
import java.util.Queue;
public class CompletedBST, Value implements BST
private Node root;
@Override
public int size
return sizeroot;
private int sizeNode x
if x null
return ;
else
return xN;
@Override
public Value getKey key
Node iter root;
whileiter null
int cmp key.compareToiterkey;
if cmp
iter iter.left;
else if cmp
iter iter.right;
else
return iter.val;
return null;
private Value getNode x Key key
etc the whole compltedbst.java is not fitting here help!!

Step by Step Solution
There are 3 Steps involved in it
Step: 1
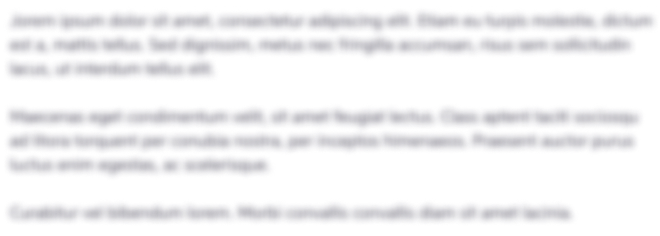
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started