Answered step by step
Verified Expert Solution
Question
1 Approved Answer
TABLE OF CONTENTS 1. Introduction 2. Download Code and Setup 3. Problem 1: Battery Meter Simulation o 3.1. Overview o 3.2. batt update.c: Updating
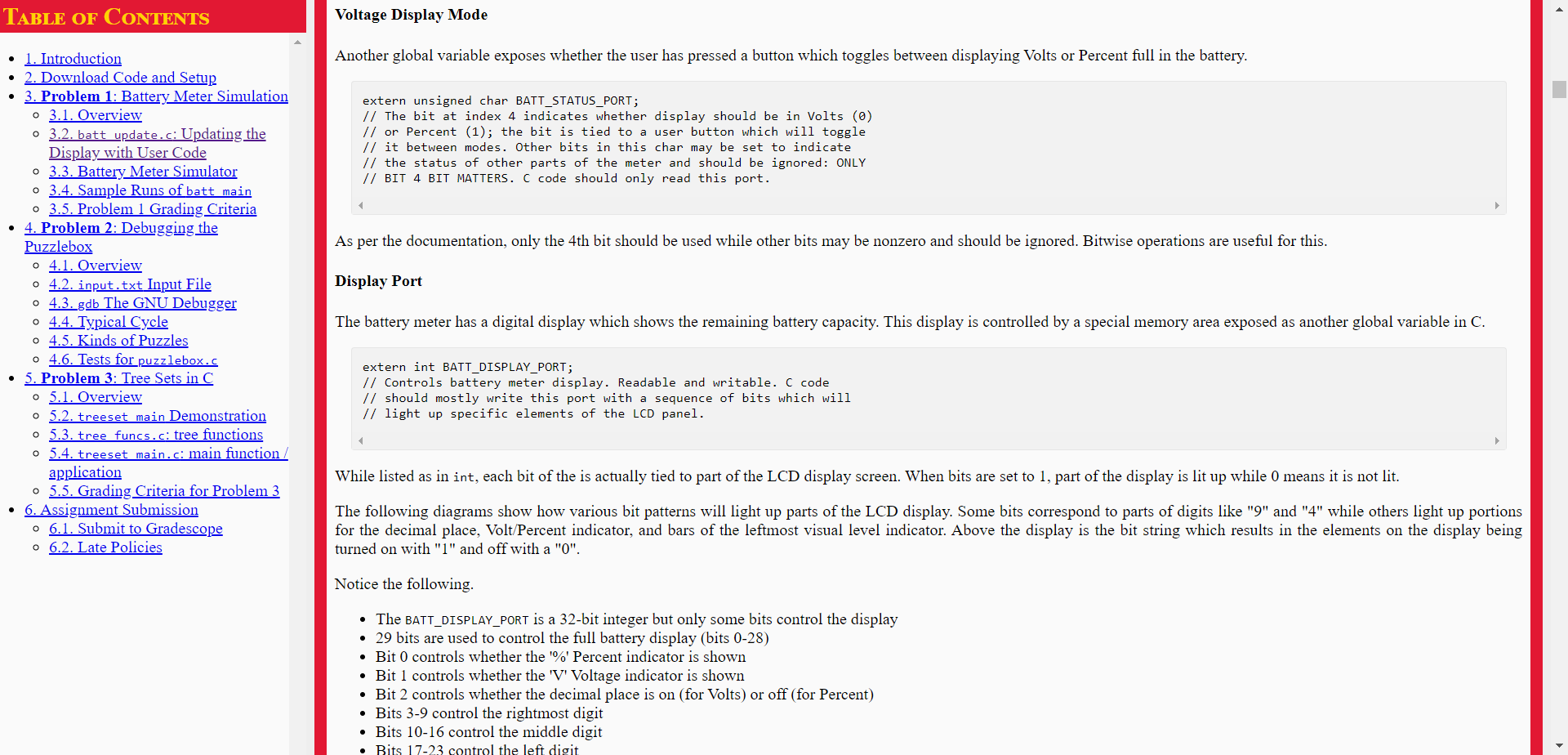
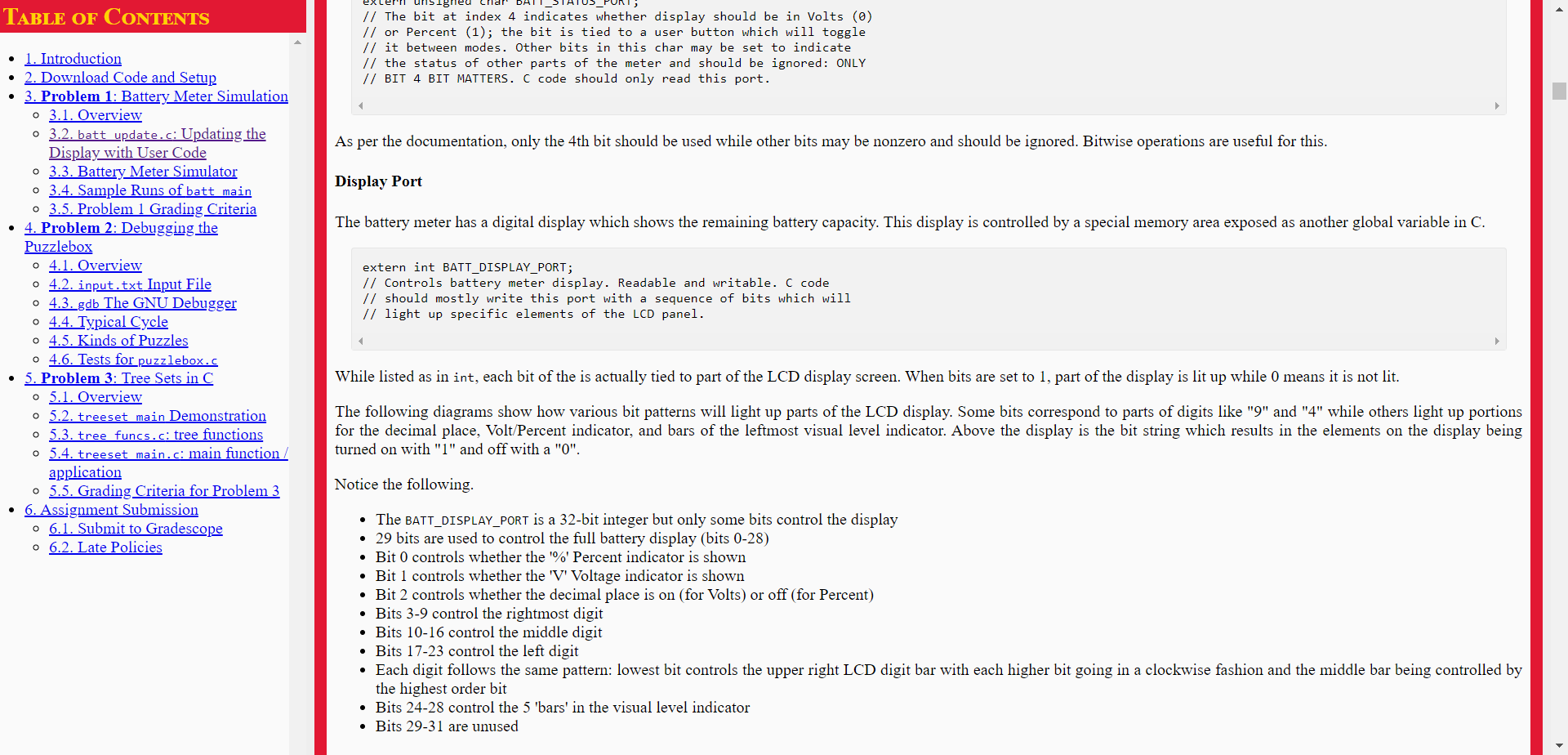

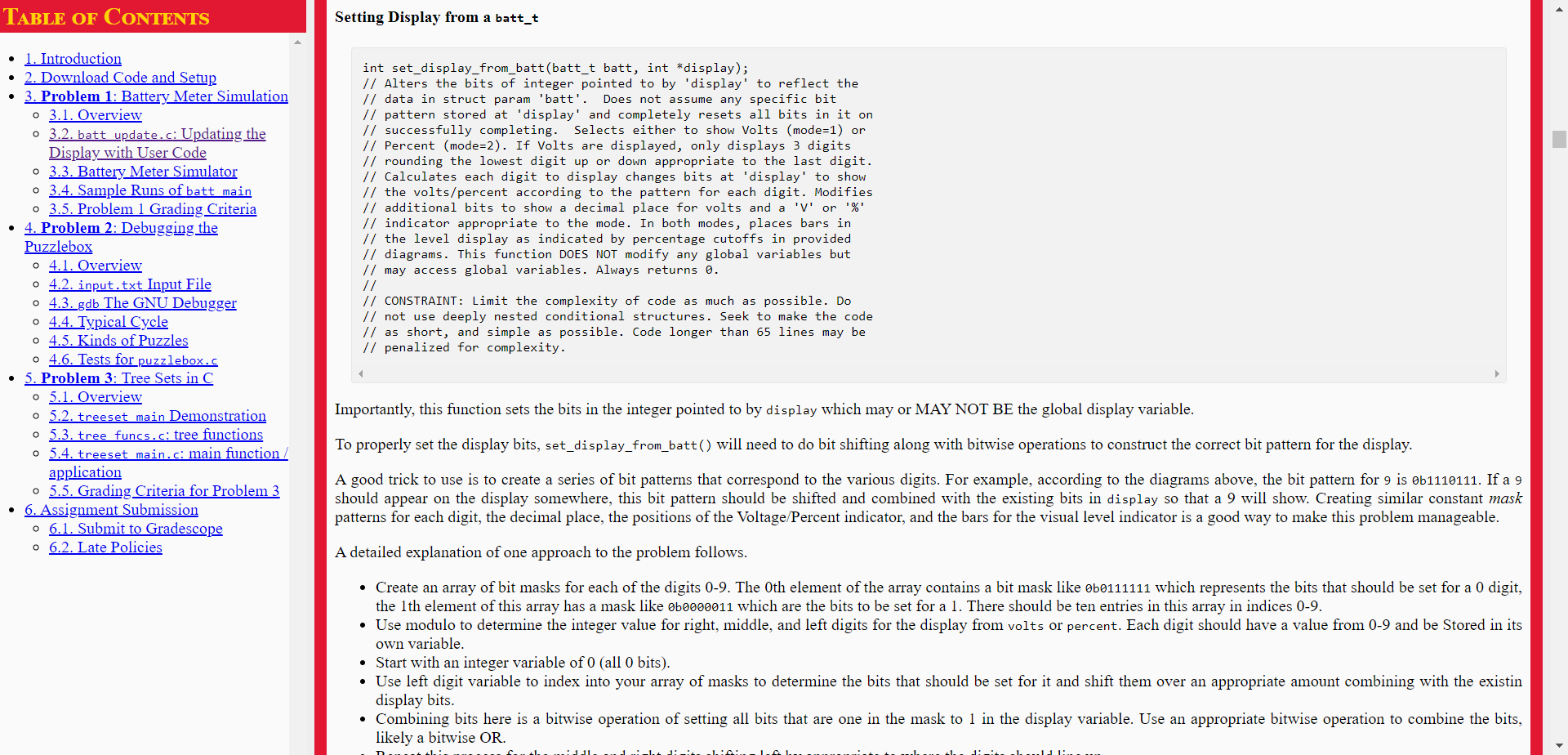
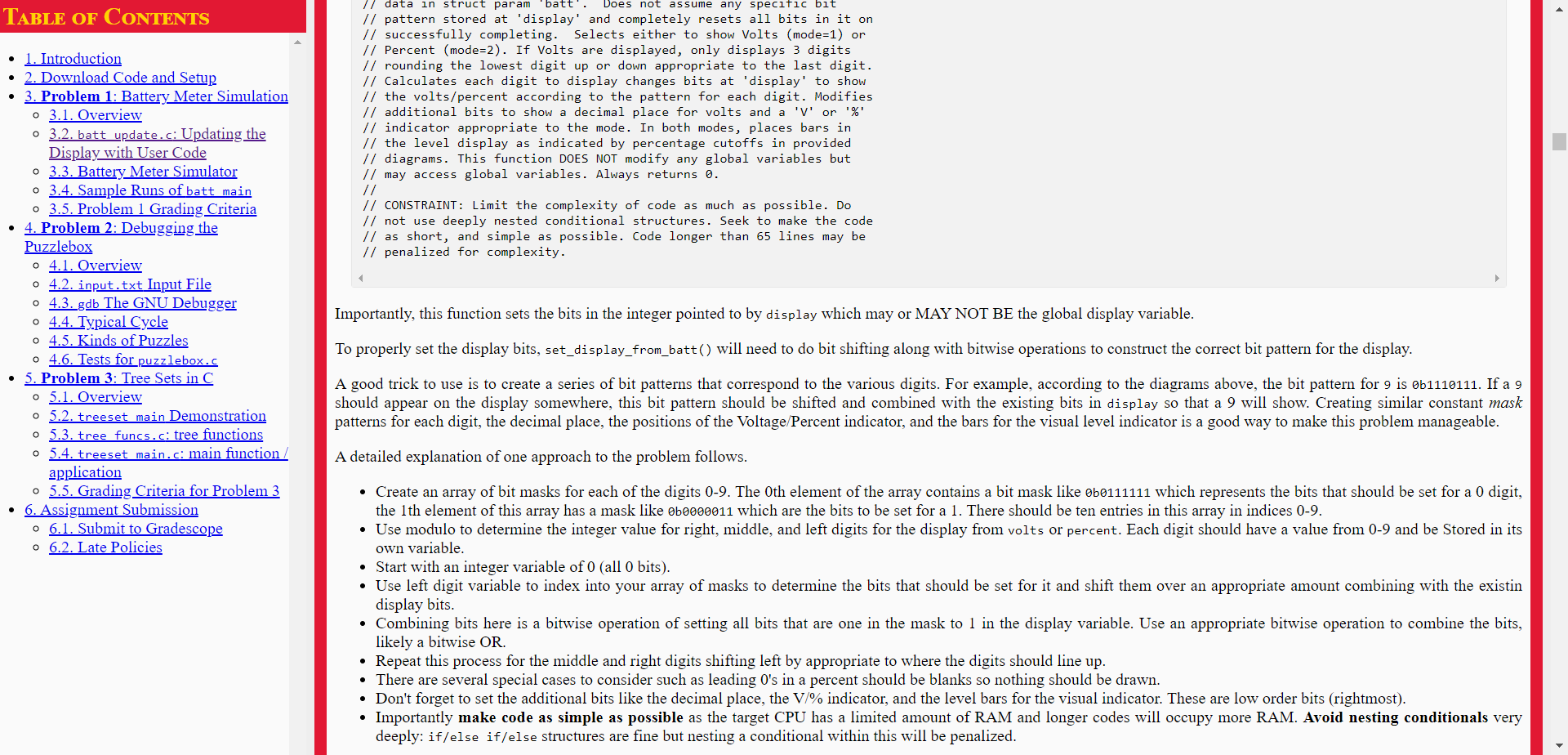
TABLE OF CONTENTS 1. Introduction 2. Download Code and Setup 3. Problem 1: Battery Meter Simulation o 3.1. Overview o 3.2. batt update.c: Updating the Display with User Code o 3.3. Battery Meter Simulator o 3.4. Sample Runs of batt main 3.5. Problem 1 Grading Criteria 4. Problem 2: Debugging the Puzzlebox o 4.1. Overview o 4.2. input.txt Input File o 4.3. gdb The GNU Debugger O 4.4. Typical Cycle o 4.5. Kinds of Puzzles o 4.6. Tests for puzzlebox.c 5. Problem 3: Tree Sets in C o 5.1. Overview o 5.2. treeset main Demonstration o 5.3. tree funcs.c: tree functions o 5.4. treeset main.c: main function / application o 5.5. Grading Criteria for Problem 3 6. Assignment Submission o 6.1. Submit to Gradescope o 6.2. Late Policies Voltage Display Mode Another global variable exposes whether the user has pressed a button which toggles between displaying Volts or Percent full in the battery. extern unsigned char BATT_STATUS_PORT; // The bit at index 4 indicates whether display should be in Volts (0) // or Percent (1); the bit is tied to a user button which will toggle // it between modes. Other bits in this char may be set to indicate // the status of other parts of the meter and should be ignored: ONLY // BIT 4 BIT MATTERS. C code should only read this port. As per the documentation, only the 4th bit should be used while other bits may be nonzero and should be ignored. Bitwise operations are useful for this. Display Port The battery meter has a digital display which shows the remaining battery capacity. This display is controlled by a special memory area exposed as another global variable in C. extern int BATT_DISPLAY_PORT; // Controls battery meter display. Readable and writable. C code // should mostly write this port with a sequence of bits which will // light up specific elements of the LCD panel. While listed as in int, each bit of the is actually tied to part of the LCD display screen. When bits are set to 1, part of the display is lit up while 0 means it is not lit. The following diagrams show how various bit patterns will light up parts of the LCD display. Some bits correspond to parts of digits like "9" and "4" while others light up portions for the decimal place, Volt/Percent indicator, and bars of the leftmost visual level indicator. Above the display is the bit string which results in the elements on the display being turned on with "1" and off with a "0". Notice the following. The BATT_DISPLAY_PORT is a 32-bit integer but only some bits control the display 29 bits are used to control the full battery display (bits 0-28) Bit 0 controls whether the '%' Percent indicator is shown Bit 1 controls whether the 'V' Voltage indicator is shown Bit 2 controls whether the decimal place is on (for Volts) or off (for Percent) Bits 3-9 control the rightmost digit Bits 10-16 control the middle digit Bits 17-23 control the left digit TABLE OF CONTENTS 1. Introduction 2. Download Code and Setup 3. Problem 1: Battery Meter Simulation o 3.1. Overview o 3.2. batt update.c: Updating the Display with User Code o 3.3. Battery Meter Simulator o 3.4. Sample Runs of batt main 3.5. Problem 1 Grading Criteria 4. Problem 2: Debugging the Puzzlebox o 4.1. Overview o 4.2. input.txt Input File o 4.3. gdb The GNU Debugger O 4.4. Typical Cycle o 4.5. Kinds of Puzzles o 4.6. Tests for puzzlebox.c 5. Problem 3: Tree Sets in C o 5.1. Overview o 5.2. treeset main Demonstration o 5.3. tree funcs.c: tree functions o 5.4. treeset main.c: main function / application o 5.5. Grading Criteria for Problem 3 6. Assignment Submission o 6.1. Submit to Gradescope o 6.2. Late Policies sign US_PORT; // The bit at index 4 indicates whether display should be in Volts (0) // or Percent (1); the bit is tied to a user button which will toggle // it between modes. Other bits in this char may be set to indicate // the status of other parts of the meter and should be ignored: ONLY // BIT 4 BIT MATTERS. C code should only read this port. As per the documentation, only the 4th bit should be used while other bits may be nonzero and should be ignored. Bitwise operations are useful for this. Display Port The battery meter has a digital display which shows the remaining battery capacity. This display is controlled by a special memory area exposed as another global variable in C. extern int BATT_DISPLAY_PORT; // Controls battery meter display. Readable and writable. C code // should mostly write this port with a sequence of bits which will // light up specific elements of the LCD panel. While listed as in int, each bit of the is actually tied to part of the LCD display screen. When bits are set to 1, part of the display is lit up while 0 means it is not lit. The following diagrams show how various bit patterns will light up parts of the LCD display. Some bits correspond to parts of digits like "9" and "4" while others light up portions for the decimal place, Volt/Percent indicator, and bars of the leftmost visual level indicator. Above the display is the bit string which results in the elements on the display being turned on with "1" and off with a "0". Notice the following. The BATT_DISPLAY_PORT is a 32-bit integer but only some bits control the display 29 bits are used to control the full battery display (bits 0-28) Bit 0 controls whether the '%' Percent indicator is shown Bit 1 controls whether the 'V' Voltage indicator is shown Bit 2 controls whether the decimal place is on (for Volts) or off (for Percent) Bits 3-9 control the rightmost digit Bits 10-16 control the middle digit Bits 17-23 control the left digit Each digit follows the same pattern: lowest bit controls the upper right LCD digit bar with each higher bit going in a clockwise fashion and the middle bar being controlled by the highest order bit Bits 24-28 control the 5 'bars' in the visual level indicator Bits 29-31 are unused This function works with the struct batt_t defined in batt.h which has the following layout. // Breaks battery stats down into constituent parts typedef struct{ short mlvolts; char percent; char mode; } batt_t; // voltage read from port, units of 0.0005 Volts (milli Volts) // percent full converted from voltage // 1 for percent, 2 for volts, set based on bit 4 of BATT_STATUS_PORT TABLE OF CONTENTS 1. Introduction 2. Download Code and Setup 3. Problem 1: Battery Meter Simulation o 3.1. Overview o 3.2. batt update.c: Updating the Display with User Code o 3.3. Battery Meter Simulator o 3.4. Sample Runs of batt main 3.5. Problem 1 Grading Criteria 4. Problem 2: Debugging the Puzzlebox o 4.1. Overview o 4.2. input.txt Input File o 4.3. gdb The GNU Debugger O 4.4. Typical Cycle o 4.5. Kinds of Puzzles o 4.6. Tests for puzzlebox.c 5. Problem 3: Tree Sets in C o 5.1. Overview o 5.2. treeset main Demonstration o 5.3. tree funcs.c: tree functions o 5.4. treeset main.c: main function / application o 5.5. Grading Criteria for Problem 3 6. Assignment Submission o 6.1. Submit to Gradescope o 6.2. Late Policies Setting Display from a batt_t int set_display_from_batt (batt_t batt, int *display); // Alters the bits of integer pointed to by 'display' to reflect the // data in struct param 'batt'. Does not assume any specific bit // pattern stored at 'display' and completely resets all bits in it on // successfully completing. Selects either to show Volts (mode=1) or // Percent (mode=2). If Volts are displayed, only displays 3 digits // rounding the lowest digit up or down appropriate to the last digit. // Calculates each digit to display changes bits at 'display' to show // the volts/percent according to the pattern for each digit. Modifies // additional bits to show a decimal place for volts and a 'V' or '%' // indicator appropriate to the mode. In both modes, places bars in // the level display as indicated by percentage cutoffs in provided // diagrams. This function DOES NOT modify any global variables but // may access global variables. Always returns 0. // // CONSTRAINT: Limit the complexity of code as much as possible. Do // not use deeply nested conditional structures. Seek to make the code // as short, and simple as possible. Code longer than 65 lines may be // penalized for complexity. Importantly, this function sets the bits in the integer pointed to by display which may or MAY NOT BE the global display variable. To properly set the display bits, set_display_from_batt () will need to do bit shifting along with bitwise operations to construct the correct bit pattern for the display. A good trick to use is to create a series of bit patterns that correspond to the various digits. For example, according to the diagrams above, the bit pattern for 9 is ob1110111. If a 9 should appear on the display somewhere, this bit pattern should be shifted and combined with the existing bits in display so that a 9 will show. Creating similar constant mask patterns for each digit, the decimal place, the positions of the Voltage/Percent indicator, and the bars for the visual level indicator is a good way to make this problem manageable. A detailed explanation of one approach to the problem follows. Create an array of bit masks for each of the digits 0-9. The 0th element of the array contains a bit mask like ob0111111 which represents the bits that should be set for a 0 digit, the 1th element of this array has a mask like ob0000011 which are the bits to be set for a 1. There should be ten entries in this array in indices 0-9. Use modulo to determine the integer value for right, middle, and left digits for the display from volts or percent. Each digit should have a value from 0-9 and be Stored in its own variable. Start with an integer variable of 0 (all 0 bits). Use left digit variable to index into your array of masks to determine the bits that should be set for it and shift them over an appropriate amount combining with the existin display bits. Combining bits here is a bitwise operation of setting all bits that are one in the mask to 1 in the display variable. Use an appropriate bitwise operation to combine the bits, likely a bitwise OR. TABLE OF CONTENTS 1. Introduction 2. Download Code and Setup 3. Problem 1: Battery Meter Simulation o 3.1. Overview o 3.2. batt update.c: Updating the Display with User Code o 3.3. Battery Meter Simulator o 3.4. Sample Runs of batt main 3.5. Problem 1 Grading Criteria 4. Problem 2: Debugging the Puzzlebox o 4.1. Overview o 4.2. input.txt Input File o 4.3. gdb The GNU Debugger O 4.4. Typical Cycle o 4.5. Kinds of Puzzles o 4.6. Tests for puzzlebox.c 5. Problem 3: Tree Sets in C o 5.1. Overview o 5.2. treeset main Demonstration o 5.3. tree funcs.c: tree functions o 5.4. treeset main.c: main function / application o 5.5. Grading Criteria for Problem 3 6. Assignment Submission o 6.1. Submit to Gradescope o 6.2. Late Policies // data in struct param 'batt'. Does not assume any specific bit // pattern stored at 'display' and completely resets all bits in it on // successfully completing. Selects either to show Volts (mode=1) or // Percent (mode=2). If Volts are displayed, only displays 3 digits // rounding the lowest digit up or down appropriate to the last digit. // Calculates each digit to display changes bits at 'display' to show // the volts/percent according to the pattern for each digit. Modifies // additional bits to show a decimal place for volts and a 'V' or '%' // indicator appropriate to the mode. In both modes, places bars in // the level display as indicated by percentage cutoffs in provided // diagrams. This function DOES NOT modify any global variables but // may access global variables. Always returns 0. // // CONSTRAINT: Limit the complexity of code as much as possible. Do // not use deeply nested conditional structures. Seek to make the code // as short, and simple as possible. Code longer than 65 lines may be // penalized for complexity. Importantly, this function sets the bits in the integer pointed to by display which may or MAY NOT BE the global display variable. To properly set the display bits, set_display_from_batt () will need to do bit shifting along with bitwise operations to construct the correct bit pattern for the display. A good trick to use is to create a series of bit patterns that correspond to the various digits. For example, according to the diagrams above, the bit pattern for 9 is ob1110111. If a 9 should appear on the display somewhere, this bit pattern should be shifted and combined with the existing bits in display so that a 9 will show. Creating similar constant mask patterns for each digit, the decimal place, the positions of the Voltage/Percent indicator, and the bars for the visual level indicator is a good way to make this problem manageable. A detailed explanation of one approach to the problem follows. Create an array of bit masks for each of the digits 0-9. The 0th element of the array contains a bit mask like ob0111111 which represents the bits that should be set for a 0 digit, the 1th element of this array has a mask like ob0000011 which are the bits to be set for a 1. There should be ten entries in this array in indices 0-9. Use modulo to determine the integer value for right, middle, and left digits for the display from volts or percent. Each digit should have a value from 0-9 and be stored in its own variable. Start with an integer variable of 0 (all 0 bits). Use left digit variable to index into your array of masks to determine the bits that should be set for it and shift them over an appropriate amount combining with the existin display bits. Combining bits here is a bitwise operation of setting all bits that are one in the mask to 1 in the display variable. Use an appropriate bitwise operation to combine the bits, likely a bitwise OR. Repeat this process for the middle and right digits shifting left by appropriate to where the digits should line up. There are several special cases to consider such as leading O's in a percent should be blanks so nothing should be drawn. Don't forget to set the additional bits like the decimal place, the V/% indicator, and the level bars for the visual indicator. These are low order bits (rightmost). Importantly make code as simple as possible as the target CPU has a limited amount of RAM and longer codes will occupy more RAM. Avoid nesting conditionals very deeply: if/else if/else structures are fine but nesting a conditional within this will be penalized.
Step by Step Solution
★★★★★
3.32 Rating (146 Votes )
There are 3 Steps involved in it
Step: 1
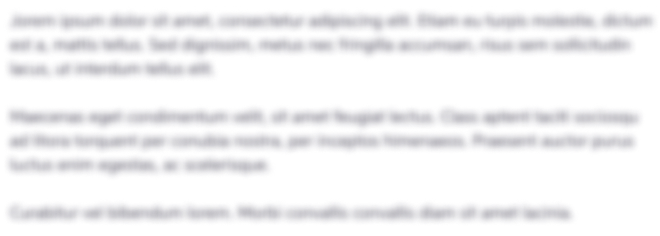
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started