Question
Take the linked list program (provided) and write a linked list implementation of the stack data structure. You can refer to the web but you
Take the linked list program (provided) and write a linked list implementation of the stack data structure. You can refer to the web but you must use the supplied code as a starting point and evolve it to work as a stack. Test the code and present your changes and the testing.(Please provide a single code for this in your answer. So that I can simply run in my compiler and see results. Someone provided answer for this question I posted on yesterday. But, it showing errors. So,please provide whole answer)
linked list programm:
A// declaring functions prototypes
#include
#include
using namespace std;
struct node {
int data;
struct node *next;
};
struct node *start = NULL;
struct node *create_ll(struct node *);
struct node *display(struct node *);
struct node *remove_beg(struct node *);
struct node *remove_list(struct node *);
struct node *remove_end(struct node *);
struct node *sort_list(struct node *);
struct node *remove_sorted(struct node *);
struct node *insert_sorted(struct node *);
int main (){
int option;
do {
cout << " Main Menu: ";
cout << " 1: Create a List";
cout << " 2: Display a List";
cout << " 3: Remove from the beginning of the List";
cout << " 4: Remove the whole list";
cout << " 5: Remove from the end of the list";
cout << " 6: Sort List";
cout << " 7: Remove from a Sorted List";
cout << " 8: Insert into a Sorted List";
cout << " 9: Exit";
cout << " Enter your option : ";
cin >> option;
switch (option) {
case 1:
start = create_ll(start);
cout << " LINKED LIST CREATED";
break;
case 2:
start = display(start);
break;
case 3:
start = remove_beg(start);
break;
case 4:
start = remove_list(start);
break;
case 5:
start = remove_end(start);
break;
case 6:
start = sort_list(start);
break;
case 7:
start = remove_sorted(start);
break;
case 8:
start = insert_sorted(start);
break;
}
}while (option != 9);
return 0;
}
struct node *create_ll(struct node *start) {
struct node *new_node;
int num;
cout << " Enter -1 to end";
cout << " Enter the data : ";
cin >> num;
while (num != -1) {
new_node = (node *) operator new (sizeof (node));
new_node->data=num;
if (start==NULL) {
new_node->next = NULL;
start = new_node;
} else {
new_node->next = start;
start = new_node;
}
cout << " Enter the data : ";
cin >> num;
}
return start;
}
struct node *display(struct node *start) {
struct node *ptr;
ptr = start;
cout << " ";
while (ptr != NULL) {
cout << ptr->data << " ";
ptr = ptr->next;
}
return start;
}
struct node *remove_beg(struct node *start) {
struct node *ptr;
if (start != NULL) {
ptr = start;
start = start->next;
delete ptr;
}
return start;
}
struct node *remove_list (struct node *start) {
struct node *ptr;
if (start != NULL ) {
ptr=start;
while(ptr != NULL) {
cout << " " << ptr->data << " is removed ";
start = remove_beg(ptr);
ptr = start;
}
}
return start;
}
struct node *remove_end(struct node *start){
struct node *ptr, *preptr;
if (start != NULL) {
ptr=start;
preptr=NULL;
while (ptr->next != NULL) {
preptr = ptr;
ptr = ptr->next;
}
if (preptr != NULL) {
preptr->next = NULL;
delete ptr;
} else {
start = remove_beg(ptr);
}
}
return start;
}
struct node *sort_list (struct node *start) {
struct node *ptr1, *ptr2;
int temp;
if (start != NULL) {
ptr1 = start;
while (ptr1->next != NULL) {
ptr2 = ptr1->next;
while(ptr2 != NULL) {
if (ptr1->data > ptr2->data){
temp = ptr1->data;
ptr1->data = ptr2->data;
ptr2->data = temp;
}
ptr2=ptr2->next;
}
ptr1=ptr1->next;
}
}
return start;
}
struct node *remove_sorted(struct node *start) {
struct node *ptr, *preptr;
int val;
bool matched;
cout << " Enter the value of the node which has to be deleted : ";
cin >> val;
if (start != NULL) {
ptr = start;
preptr=NULL;
while (ptr->data != val && ptr->next != NULL) {
preptr=ptr;
ptr = ptr->next;
}
if (ptr->data == val) {
if (preptr != NULL){
preptr->next = ptr->next;
delete ptr;
} else {
start = remove_beg(ptr);
}
}else {
cout << " no match ";
}
}
return start;
}
struct node *insert_sorted(struct node *start){
struct node *new_node, *ptr, *preptr;
int num;
cout << " Enter the data : ";
cin >> num;
new_node = (node *) operator new (sizeof (node));
new_node->data = num;
if (start != NULL ) {
ptr= start;
while (ptr->data < num) {
preptr = ptr;
ptr = ptr->next;
if (ptr == NULL) {
break;
}
}
if (ptr == NULL) {
preptr->next = new_node;
new_node->next = NULL;
} else if (ptr == start) {
new_node->next = start;
start = new_node;
} else {
new_node->next = ptr;
preptr->next = new_node;
}
} else {
start = new_node;
start->next=NULL;
}
return start;
}
Am providing stack programme also, but you can write your own stack programm. I just adding it.
# include
#define MAX 10 // added
int st[MAX], top= -1;
void push (int st[], int val);
int pop(int st[]);
int peek(int st[]);
void display(int st[]);
int main()
{
int val,option;
//clrscr(); was commented out
do
{
printf(" **MAIN MENU**");
printf(" 1.PUSH");
printf(" 2.POP");
printf(" 3.PEEK");
printf(" 4.DISPLAY");
printf(" 5.Exit Program");
printf(" *************");
printf(" Enter your option: "); // removed typo 'f'
scanf("%d",&option);
switch(option)
{
case 1:
printf(" Enter the number to be pushed on to the stack:");
scanf("%d",&val);
push(st, val);
break;
case 2:
val = pop(st);
printf(" The value deleted from the stack is : %d", val);
break;
case 3:
val = peek(st);
break;
case 4:
display(st);
break;
case 5: // A good bye message was added
printf(" Good Bye");
break;
default: // added this because all switches
printf(" Invalid choice! "); // needed a default if a choice
break; // was invalid
}
}while(option != 5);
//getch(); // dev specific it is used to halt system till user input
return 0;
}
void push( int st[], int val) // in to int
{
if(top == MAX-1)
{
printf(" STACK OVERFLOW");
}
else
{
top++;
st[top] = val;
}
}
int pop(int st[])
{
int val;
if(top == -1)
{
printf(" STACK UNDERFLOW");
return -1;
}
else
{
val = st[top]; // added ; to the end
top--;
return val;
}
}
void display(int st[])
{
int i;
if(top == -1)
printf(" STACK IS EMPTY");
else
{
for(i=top;i>=0;i--)
printf(" %d",st[i]);
}
}
int peek(int st[]) // peep to peek
{
if(top == NULL) // lowercased TOP to top
{
printf(" STACK IS EMPTY");
return -1;
}
else {
printf(" %d",st[top]);
return(st[top]);
}
}
Please provide a final programm based on the linked list provided
Step by Step Solution
There are 3 Steps involved in it
Step: 1
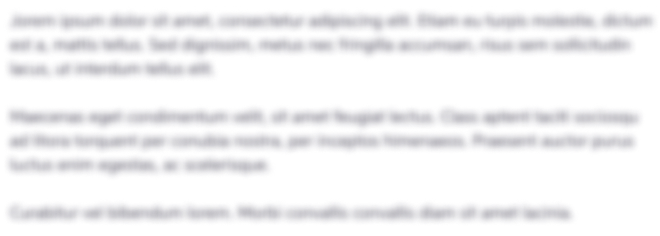
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started