Question
TASKS (PLEASE CODE IN JAVA) ALL CODE IS GIVEN ONLY NEEDED CHANGES TO HOMEOWNERS AND POLICY EXCEPTION CLASS 1.Create the PolicyExceptionclass according to its UML
TASKS (PLEASE CODE IN JAVA) ALL CODE IS GIVEN ONLY NEEDED CHANGES TO HOMEOWNERS AND POLICY EXCEPTION CLASS
1.Create the PolicyExceptionclass according to its UML Class Diagram. a.There will be two constructors b.There will not be a null constructor. 2.Modify HomeOwnersclasses so that they meet the revised requirements as shown in the UML class diagrams. a.Add the required validation code as explained in the UML diagrams. NOTE:for some validations, a specific method of validating input is required. b.Throw a PolicyExceptionexception when an object cannot be successfully instantiated. c.Exceptions thrown in set methods should be caught in constructors and then chained. The new, chained exception should includeall the values submitted to the constructor for instantiate of the object. The chained exception will be thrown as a PolicyExceptiontype. d.Modify the toStringof both HomeOwnersclasses to: a.Use the superclass toStringmethod to provide basic policy information. b.Use a StringBuilderobject to build the output.
HomeOwners 1.Modify the subclass HomeOwners according to the UML requirements and instructions as provided. 2.Property type must be digit between 1 and 5, inclusive. If the offered value is out of range, assign the default value of 1 to the instance variable. Continue with instantiation of the object. 3.The insured value of a structure must be greater than 0, but not more than 1,000,000. If the offered value is out of range, the object cannot be instantiated. 4.The insured value of the contents must be greater than 0, but not more than 1,000,000. . If the offered value is out of range, the object cannot be instantiated. 5.The deductible must be between 1% and 10%, inclusive. . If the offered value is out of range, the object cannot be instantiated. 6.If a validation fails, a PolicyException will be thrown. The message used to instantiate the exception object must include all values offered to the constructor. 7.The toStringmethod will be updated to meet the following requirements: a.For the first portion of the output, call the superclass toStringmethod. b.Use a StringBuilderobject to build the output
PolicyException Write code for the subclass PolicyExceptionaccording to the UML requirements as provided.
NOTES on ExceptionsExceptions thrown in the class code (HomeOwners) should be chained and passed up the call stack to the test harness. Completeand meaningful messages should be provided for any/every error. Except for the requirement to include all values offered at the constructor level, the form and wording of exception messages is up to the team.
PROVIDED CODE: AUTO CLASS public class Auto extends Policy { private double exposureRate; // private String makeModel; // Make and model of auto private int modelYear; // Model year of auto private String vin; // Vehicle ID number private static int limits[] = new int[3]; // Array of int values representing coverage limits private int deduct; // Policy deductible, stated in dollars public Auto() { }//END Auto null constructor public Auto(String own, String insd, String nbr, double prem, String model, int year, String id, int[] lims, int ded) { super(own, insd, nbr, prem); setMakeModel(model); setModelYear(year); setVin(id); setLimits(lims);//set array variable setDeduct(ded); setExposureRate(); }//END Auto full constructor /* * SET METHODS */ public final void setMakeModel(String model) { makeModel = model; }//END setMakeModel public final void setModelYear(int year) { modelYear = year; }//END setModelYear public final void setVin(String id) { vin = id; }//END setVin public final void setLimits(int[] lims) { limits = lims; } public final void setDeduct(int ded) { deduct = ded; }//END deduct public final void setExposureRate() { exposureRate = .0051; }//END setExposureRate /* * GET METHODS */ public final String getMakeModel() { return makeModel; }//END getMakeModel public final int getModelYear() { return modelYear; }//END modelYear public final String getVin() { return vin; }//END getVin public final int[] getLimits() { return limits; } public final int getDeduct() { return deduct; }//END getDeduct public final double getExposureRate() { return exposureRate; } /* * OTHER METHODS */ public String produceLimits() { return String.format("Collission: $%,.2f, Comprehensive: $%,.2f, UIM: $%,.2f", limits[0]*1000, limits[1]*1000, limits[2]*1000); }//END produceLimits public double calcExposure() { return (limits[0] + limits[1] + limits[2]) * 1000; }//END calcExposure public double calcCurrentValue() { return getPolPrem() - (calcExposure() * getExposureRate()); }//END calcCurrentValue public String toString()
{ return String.format("%n %s" + "This Auto policy insures a %d %s, VIN %s, with limits of %s and a deductible of $%,.2f.", super.toString(), getModelYear(), getMakeModel(), getVin(), produceLimits(), getDeduct()); }//END toString }//END Auto class
HOMEOWNERS CLASS public class HomeOwners extends Policy { private double exposureRate; // current exposureRate for HomeOwners Policy private String propAddress; // legal address of insured property private int propType; // numeric code describing property type private int structure; // structure coverage limit, stated in thousands of dollars private int contents; // contents limits, stated in thousands of dollars private double deduct; // policy deductible as a percentage of total insured value private boolean umbrella; // boolean indicating if policy is tied to umbrella coverage public HomeOwners() { super(); }// end null constructor public HomeOwners( String own, // owed to super class String insd, // owed to super class String nbr, // owed to super class double prem, // owed to super class String addr, // subclass homewoners variable int type, // subclass homewoners variable int struct, // subclass homewoners variable int goods, // subclass homewoners variable double ded, // subclass homewoners variable boolean umbr ) // subclass homewoners variable { super( own, insd, nbr, prem); setExposureRate(); setPropAddress(addr); setPropType(type); setStructure(struct); setContents(goods); setDeduct(ded); setUmbrella(umbr); }//end full constructor /* * SET METHODS */ public final void setPropAddress( String addr ) { propAddress = addr; }// end setPropAddress public final void setPropType( int type ) { propType = type; }// end setProType public final void setStructure( int struct ) { structure = struct; }// end setStructure public final void setContents( int goods ) { contents = goods; }// end setContent public final void setDeduct( double ded ) { deduct = ded; }// end setDeduct public final void setUmbrella( boolean umbr ) { umbrella = umbr; }// end setUmbrella public final void setExposureRate() { exposureRate = .0025; }//END exposureRate /* * GET METHODS */ public final String getPropAddress() { return propAddress; }// end getPropAddress public final int getPropType() { return propType; }//end getProType public final int getStructure() { return structure * 1000; }//end getStructure public final int getContents() { return contents * 1000; }//end getContent public final double getDeduct() { return deduct; }//end getDeduct public final boolean getUmbrella() { return umbrella; }//end getUmbrella public final double getExposureRate() { return exposureRate; }//END getExposureRate public final double getDeductInDollars() { return ((getStructure() + getContents()) * getDeduct()); }//End getDeductInDollars /* * OTHER METHODS */ public double calcExposure() { return (getStructure() + getContents()); }//End calExposure public double calcCurrentValue() { return getPolPrem() -(calcExposure() * getExposureRate()); }//End calcCurrentValue public String toString() { String testUmbrella; //Stores a string related to boolean umbrella if(getUmbrella()) { testUmbrella = "This policy is part of an Umbrella contract"; } else { testUmbrella = "This policy is not part of an Umbrella contract"; } return String.format("%n " + super.toString() + "This Homewners policy insures a type %d home at %s. " + "The structure is insured for $%,.2f; contents for $%,.2f. " + "The dudctible is $%,.2f %s", getPropType(), getPropAddress(), (double)getStructure(), (double)getContents(), getDeductInDollars(), testUmbrella); }//END toString }//End Class
KEYBOARD INPUT /** * This is essentially a 'helper' class holding methods for retrieving keyboard input * * The methods assume a Scanner object named input has been associated with the keyboard. * * Each static method requires as input a String value to be used as a prompt to the user. * * Each static method returns a different primitive data type to the caller. */
import java.util.Scanner;
public class KeyboardInput { public static Scanner input = new Scanner( System.in ); /******************************************************************************************************************* * Methods for collecting user input from keyboard *******************************************************************************************************************/ /******************************************************************************** * This method returns a String * 1. Ask the user for the necessary input * 2. Return that input to the calling method ********************************************************************************/ public static String acceptString( String prompt ) { System.out.printf( "%n%s%n", prompt); return input.nextLine(); } // end acceptString /******************************************************************************** * This method returns an int * 1. Ask the user for the necessary input * 2. Clear the 'hanging' line feed from the keyboard * 3. Return the int value to the calling method ********************************************************************************/ public static int acceptInteger( String prompt ) { System.out.printf( "%n%s%n", prompt ); int aNumber = input.nextInt(); input.nextLine(); return aNumber; } // end acceptInteger /******************************************************************************** * This method returns a double * 1. Ask the user for the necessary input * 2. Clear the 'hanging' line feed from the keyboard * 3. Return the double value to the calling method *******************************************************************************/ public static double acceptDouble( String prompt ) { System.out.printf( "%n%s%n", prompt); double aNumber = input.nextDouble(); input.nextLine(); return aNumber; } // end acceptDouble
/******************************************************************************** * This method returns a float * 1. Ask the user for the necessary input * 2. Clear the 'hanging' line feed from the keyboard * 3. Return the float value to the calling method *******************************************************************************/ public static float acceptFloat( String prompt ) { System.out.printf( "%n%s%n", prompt); float aNumber = input.nextFloat(); input.nextLine(); return aNumber; } // end acceptDouble /******************************************************************************** * This method returns a boolean * 1. Ask the user for the necessary input * 2. Return the boolean value to the calling method *******************************************************************************/ public static boolean acceptBoolean( String prompt ) { System.out.printf( "%n%s%n", prompt); boolean aSwitch = input.nextBoolean(); return aSwitch; } // end acceptDouble }
POLICY CLASS /* * This class will describe a policy object representingan owner's policy */ public abstract class Policy implements BookValue { /* * INSTANCE VARIABLES */ private String owner; // Policy owner's full, legal private String insured; // Full, legal name of named insured on policy private String polNbr; // Unique identifer for specific policy private double polPrem; // Annual premium amount for policy
/* * CONSTRUCTORS */
public Policy() { // NO code required here.. } // End null consturctor public Policy(String own, String insd, String nbr, double prem) {
setOwner(own); setInsured(insd); setPolNbr(nbr); setPolPrem(prem); } // end full Contrutor
/* * Set Methods */
public final void setOwner(String own) { owner = own; } // End setOwner
public final void setInsured(String insd) { insured = insd; } // End setInsured
public final void setPolNbr(String nbr) { polNbr = nbr; } // End setPolNbr
public final void setPolPrem(double prem) { polPrem = prem; } // End setPolPrem
/* * GET METHODS */
public final String getOwner() { return owner; } // End getOwner
public final String getInsured() { return insured; } // End getInsured
public final String getPolNbr() { return polNbr; } // End getpolNbr
public final double getPolPrem() { return polPrem; } // End getPolPrem
/* * OTHER METHODS */
public String identifyContract() { return getPolNbr(); }
public String toString() { // Return a verbose description of the object, displaying all instance values
return String.format("%s owns Policy %s, insuring %s, with a premnium of $%,.2f.%n", getOwner(), getPolNbr(), getInsured(), getPolPrem());
} // End toString
} // End Policy
TEST_XCPTNHANDALING /** * Test Harness for PA03 * * DO NOT CHANGE CODE IN THIS TEST HARNESS! * * This test harness, with the 'helper' class KeyboardInput, will allow for continuous data entry from the keyboard. * * You will want to fully test each validation you've written. Only one validation error per object entry can be * caught and reported. This means you'll need to do A LOT OF TESTING to test all of your validations. * * (c) 2018 Terri Davis */
//import KeyboardInput // The import is not necessary, but it's good to remember we're using this!
public class Test_XcptnHandling { // This object ( myPolicy) provides a 'holding space' for a reference to an object in the InsurancePolicy hierarchy. // NOTE: We are NOT INSTANTIATING an InsurancePolicy object here, just setting up a reference. private static Policy myPolicy; /************************************************************************************************************* * The main method will consist of a continuous input loop based on the boolean variable goAgain. * Use a while loop (while goAgain = true) to contol processing: * Ask user which type of policy to create * If Auto * call method to create Auto object * ElseIf HomeOwners * call method to create HomeOwners object * Else * Tell user valid options * Ask user whether to continue *************************************************************************************************************/ public static void main(String[] args) { boolean goAgain = true; // Set the initial value of the loop control variable to continue the loop. while ( goAgain ) // Initiate an input loop based on the control variable { try // The try block is placed INSIDE the loop to allow continous input { /* * The following statement calls the acceptString method of KeyboardInput. The charAt method of the returned * String object is then called to retrieve the first character of the String. That char is then converted * to upper case and the resulting value is stored in the variable polType */ char polType = Character.toUpperCase( KeyboardInput.acceptString( "Build an Auto policy (A) or a HomeOwners policy (H)? " ).charAt(0) ); /* * Test variable polType. If the value is 'A', go on to collect data to create an Auto object. * If the value is 'H', collect data to create a HomeOwners object. * If the value is neither, let the user know what the acceptable values are. */ if( polType == 'A' ) { buildAutoPolicy( ); // Call method to collect data and instantiate an Auto object } else if( polType == 'H' ) { buildHomeOwnersPolicy( ); // Call method to collect data/instantiate a HomeOwners object } else { // Remind user of the acceptable responses to the question about policy type... System.out.printf( "%n%s%n", "You must select Auto (A) or HomeOwners (H)." ); } } // end try block catch( PolicyException buildError ) { // Catch any validation exception that may have been thrown and display to the console. System.out.printf( "%n%s%n", buildError.getMessage( ) ); } // end catch block // Aks user if they wish to continue the loop -- the loop does not end if a PolicyException was thrown. goAgain = KeyboardInput.acceptBoolean( "Do you wish to enter another? true or false " ); } // end while loop System.out.printf( "%nThanks for playing!%n" ); } // end main method /************************************************************************************************************* * Use methods of KeyboardInput to deliver prompts and accept input from user. * Collect values for all non-static instance variables fo class Auto. * Instantiate an Auto object using those values; assign the object reference to myPolicy * Call the toString method of myPolicy to output information about the instantiated object * * IF a PolicyException is thrown, it is NOT CAUGHT AT THIS LEVEL; it is 'passed through' to the main method * and will be caught & handled there. *************************************************************************************************************/ private static void buildAutoPolicy( ) throws PolicyException { // Variables to hold values required for the superclass level (InsurancePolicy) String own, insd, pol; double prem; // Variables to hold values required for Auto subclass String mkMod, vin; int year, coll, comp, uim, ded; int[] limits = new int[3]; // Gather superclass level values own = KeyboardInput.acceptString( "Policy Owner: " ); insd = KeyboardInput.acceptString( "Insured: " ); pol = KeyboardInput.acceptString( "Policy Number (2 letters, 5 digits): " ); prem = KeyboardInput.acceptDouble( "Annual premium (no dollar sign): " ); // Gather Auto subclass level values mkMod = KeyboardInput.acceptString( "Make & Model (i.e., Ford Fusion): " ); year = KeyboardInput.acceptInteger( "Year of car (four digits): " ); vin = KeyboardInput.acceptString( "VIN (17 characters): " ); coll = KeyboardInput.acceptInteger( "Collision limit (no dollar sign): " ); comp = KeyboardInput.acceptInteger( "Compehensive limit (no dollar sign): " ); uim = KeyboardInput.acceptInteger( "Uinsured Motorist limit (no dollar sign): " ); ded = KeyboardInput.acceptInteger( "Deductible (no dollar sign): " ); // load limits array limits[0] = coll; limits[1] = comp; limits[2] = uim; // Instantiate object; set myPolicy to refer to new object myPolicy = new Auto( own, insd, pol, prem, mkMod, year, vin, limits, ded ); System.out.printf( "%n%s%n", myPolicy.toString( ) ); } // end buildAutoPolicy /************************************************************************************************************* * Use methods of KeyboardInput to deliver prompts and accept input from user. * Collect values for all non-static instance variables fo class HomeOwners. * Instantiate an HomeOwners object using those values; assign the object reference to myPolicy * Call the toString method of myPolicy to output information about the instantiated object * * IF a PolicyException is thrown, it is NOT CAUGHT AT THIS LEVEL; it is 'passed through' to the main method * and will be caught & handled there. *************************************************************************************************************/ private static void buildHomeOwnersPolicy( ) throws PolicyException { // Variables to hold values required for the superclass level (InsurancePolicy) String own, insd, pol; double prem; // Variables to hold values required for HomeOwners subclass String address; int type, struct, cont; double deduct; boolean umb;
// Gather superclass level values own = KeyboardInput.acceptString( "Policy Owner: " ); insd = KeyboardInput.acceptString( "Insured: " ); pol = KeyboardInput.acceptString( "Policy Number (2 letters, 5 digits): " ); prem = KeyboardInput.acceptDouble( "Annual premium (no dollar sign): " ); // Gather HomeOwners subclass level values address = KeyboardInput.acceptString( "Property Address: " ); type = KeyboardInput.acceptInteger( "Property Type Code (1 - 5): " ); struct = KeyboardInput.acceptInteger( "Insured value of structure: " ); cont = KeyboardInput.acceptInteger( "Insured value of contents: " ); deduct = KeyboardInput.acceptDouble( "Deductible as a Percentage of value (i.e., .05 for 5%): " ); umb = KeyboardInput.acceptBoolean( "Is this part of an Umbrella contract (true or false)?" ); // Instantiate object; set myPolicy to refer to new object myPolicy = new HomeOwners( own, insd, pol, prem, address, type, struct, cont, deduct, umb ); System.out.printf( "%n%s%n", myPolicy.toString( ) ); } // end buildHomeOwnersPolicy } // end Test_XcptnHandling test harness
Step by Step Solution
There are 3 Steps involved in it
Step: 1
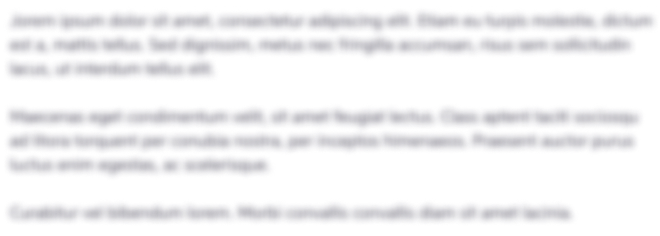
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started