TASKS The task is to Write academic integrity comment in pizza_shop.py (Modify the first comment in the file) Write academic integrity comment in test_driver.py (Modify
TASKS
The task is to Write academic integrity comment in "pizza_shop.py" (Modify the first comment in the file)
Write academic integrity comment in "test_driver.py" (Modify the first comment in the file)
Initialize git repository (Make commits after every major change (minimum 10))
Write ingredient class(es)
Write a unittest or a pytest for PizzaBase class (see Unit Test section below)
Write pizza class(es)
Write pizza shop class(es)
Test thoroughly
Ensure output matches sample output (see Sample Output section below)
Remove all "TODO" comments in your code.
REQUIREMENTS
Do the following throughout the project while writing each method of every class:
Make a git commit after every major change (minimum 10). Ensure they have a descriptive commit message.
Test each method while writing each method. Comment out your tests after you know they are working.
Write doc strings to describe each class and each method (see Doc Strings section below).
Write inline comments to describe important sections of code.
Ensure you follow "Best Practice" (see Best Practice section below).
You must update your UML diagram to reflect any changes made to your design. Ensure your system meets the following requirements:
You must demonstrate multiple examples of inheritance, aggregation, composition, and association.
You must demonstrate polymorphism by calling overridden methods, or by checking the types of objects.
You must demonstrate good encapsulation by making variables private or protected and providing getter and/or setter methods to them.
You must demonstrate how to raise and handle exceptions.
DOCSTRINGS
Please provide a descriptive docstring on the first line of each of your classes and methods.
Docstrings are written in '''triple quotes''' on the first line inside a class or method, such as:
class Food:
'''Superclass for other types of Foods in the PizzaShop. '''
def __init__(self, name, price):
'''Initialises name and price. Called by subclasses using super().
'''
pass #TODO initialise name and price
Document your code as you go, not all at the end. Write a brief docstring about the one thing that the method is supposed to do before you write the code. Refer to that docstring to remind yourself of the problem you need to solve or the code you need to write. This helps maintain focus when implementing the method and should stop you from making it do more than the one thing that the docstring says it should do.
BEST PRACTICE
Ensure you do the following or you may lose marks, especially for not implementing relationships correctly:
Avoid global variables. Ensure most of your code is written within methods within classes. Write code in the main function() to construct your object(s) and start the program e.g.:
def main():
# TODO Write your main program code here...
print("~~ Welcome to the Pizza Shop ~~")
print()
shop = PizzaShop() # Construct object
shop.command_prompt() # Call method to start the program
# Write the rest of your code inside classes...
Use self correctly e.g.:
class Food:
def __init__(self, name, price):
self.set_price(price) # GOOD! Self as calling object.
Food.set_name(self, name) # BAD! Self as argument. Do not do this.
Avoid class-variables (you will not need them). Use instance-variables instead e.g.:
class Food():
name = "tomato" # BAD! This class-variable is unnecessary. Do not do this.
def __init__(self, name):
self.__name = name # GOOD! Instance-variables belong to self
Avoid circular imports. If this file imports that file and that file imports this file, we have a circular import. Python cannot resolve circular imports. Avoid this by writing classes in one file.
FILES
You have been provided with a folder called files containing an empty folder called receipts and two other files: menu.txt and ingredients.txt.
ingredients.txt
The ingredients file contains information which will be used to construct the various pizza ingredients. Each line represents the name and price for one ingredient. For Food items, the format is "name $price". PizzaBases are prepended with the string "base:" such as "base: deep pan $8". In this case, name is "deep pan" and price is 8.0. The file may contain the following:
base: deep pan $8
base: thin crust $8
base: cheese crust $12
tomato sauce $0
bbq sauce $0
sweet chilli sauce $0.5
chedder cheese $1
mozzarella cheese $1
fetta cheese $1
ham $2
salami $3
chicken $4
prawns $5
pineapple $2
onion $1
capsicum $1
jalapenos $2.5
olives $2
mushrooms $2.5
spinach $3
anchovies $2.5
menu.txt
The menu file contains the recipe for making all the pizzas that the pizza shop offers. Each line is in the format "pizza name $price ingredient name 1, ingredient name 2, ...". The file may contain the following:
Margarita Pizza $12 tomato sauce, mozzarella cheese, chedder cheese
Hawaiian Pizza $12 tomato sauce, mozzarella cheese, ham, pineapple
Meat Lovers Pizza $12 bbq sauce, mozzarella cheese, ham, salami, onion
Supreme Pizza $12 tomato sauce, mozzarella cheese, ham, mushrooms, capsicum, salami, olives, pineapple
Vegetarian Pizza $12 tomato sauce, fetta cheese, capsicum, pineapple, mushrooms, spinach, olives
BBQ Chicken Pizza $14 bbq sauce, mozzarella cheese, chicken, onion
Spicy Seafood Pizza $14 sweet chilli sauce, mozzarella cheese, prawns, anchovies, jalapenos, capsicum, onion
Chicken and Prawn Pizza $16 sweet chilli sauce, fetta cheese, chicken, prawns, spinach
Your PizzaShop class will need to implement a method called load_ingredients() that reads the ingredients.txt file and, for each line, extracts the name and price. If it starts with the word "base: ", it will construct a PizzaBase, otherwise it will construct a Food with the extracted information. The Food will then be added to the PizzaShop's ingredients.
The PizzaShop class must implement a method called loadMenu() that reads the menu file and constructs a Pizza using information extracted from each line. To construct a Pizza you will need the name, price, and a list of toppings, a size, and a pizza base. You can assume that all Pizzas in the file are on a "large", "thin crust", PizzaBase. Each topping should be accessed from the list created using the loadIngredients() method. These toppings should be added to a new list and used to construct the Pizza. The Pizza will then be added to the PizzaShop's PizzaMenu.
Receipts
The receipts folder will contain files representing orders made to the Pizza Shop. Each file name will be the name of the person who purchased the pizza. Whenever a person orders a pizza, their receipt will be appended to the file with their name. If a user named Jane ordered some pizzas, the receipts folder may contain a file named "Jane.txt" containing the following:
Spicy Seafood Pizza, small cheese crust $12.12:
sweet chilli sauce, mozzarella cheese, prawns, anchovies, jalapenos, capsicum
Meat Lovers Pizza, large thin crust $15.50:
bbq sauce, mozzarella cheese, ham, salami, onion, anchovies, fetta cheese
Enjoy your meal Jane! :)
Notice that there may be multiple orders in the same file. These orders may be made at different times.
CLASSES
The following is a guide for how to write Food and its subclasses. You may follow your own UML design if you believe it will work, but please also meet the minimum requirements described in this section. Please update your UML diagram to reflect your code.
All variables must be hidden, and appropriate accessor methods must be provided for each.
Food
Food has a name and price. Food could represent pizzas, pizza toppings, or pizza bases or any other menu item. The class must have an appropriate __init__ method, instance variables for name and price, and appropriate getter and setter methods to access them.
The class must provide the following methods which will be overridden in each subclass:
clone(other)
Returns a new copy of this object with no memory references in common with the calling object.
equals(other)
Returns True if the calling object is considered equal to the other argument. To be considered equal, self and other must be the same type and contain the same values in each of their variables. If they are not equal, the method returns False.
At minimum, it must also have methods to do the following:
Get the price as a string in the format $1.00 i.e., starting with a dollar sign and formatted to 2 decimal places.
PizzaBase
PizzaBase represents the round bread that the pizza toppings will be added to. It should be derived from Food. It must have appropriate instance variables for the diameter measured in inches. The class must override the following methods:
clone(other)
Returns a new copy of this object with no memory references in common with the calling object.
equals(other)
Returns True if the calling object is considered equal to the other argument. To be considered equal, self and other must be the same type and contain the same values in each of their variables. If they are not equal, the method returns False.
At minimum, it must also have methods to do the following:
Calculate the cost per square inch. For example, if a large, cheese-crust pizza base costs $12, it's cost per square inch is calculated with the expression: $12 / (diameter/2) *2 .
Set the diameter and scale the cost of the PizzaBase by the cost per square inch multiplied by the newly calculated square inches.
A method that takes a string, either "small", "medium", or "large", and sets the diameter to 10, 12, or 14 respectively. Then scale the cost of the PizzaBase by the cost per square inch multiplied by the newly calculated square inches. This should raise an exception with an appropriate error message, if the string is invalid.
Note: You will need to write a PyTest or Unittest for PizzaBase in the file test_driver.py. You should do that now before you move on. Please see the Unit Test section below for instructions.
Pizza
Pizza contains a pizza base and toppings. It should be derived from Food. It must have appropriate instance variables for the original base, original toppings, current base, and current toppings.
The class must override the following methods:
clone(other) Returns a new copy of this object with no memory references in common with the calling object. Note: You will need to be able to clone a pizza from a menu item e.g., Hawaiian Pizza, Meat Lover's Pizza, etc.
equals(other) Returns True if the calling object is considered equal to the other argument. To be considered equal, self and other must be the same type and contain the same values in each of their variables. If they are not equal, the method returns False.
At minimum, it must also have methods to do the following:
Add a topping to the current toppings.
Remove a topping from the current toppings.
The pizza's get_price() method must add the price for any food that is in toppings that was not in original toppings and also add the difference in price between the pizza base and original base.
Pizza Shop
The pizza shop allows us to open and operate our restaurant. It will allow the user to order and customize pizzas using a text-based interface and save receipts for those orders to a file. The user may order any number of pizzas before exiting the program. The receipt for each order will be written to a file named with the user's name e.g., "mary.txt". You must have the ability to display the receipt before making your order.
It must have instance variables for menu pizzas and pizza toppings.
At minimum, it must have methods to do the following:
Read the ingredients from file and use the information to construct a list of Food objects and a list of PizzaBase objects.
Read the menu from file and use the information to construct a list of template pizzas objects.
Choose and clone a Pizza from the pizza menu. You must display the menu in the following format: Menu:
Margarita Pizza, large thin crust $12.00:
tomato sauce, mozzarella cheese, chedder cheese
Hawaiian Pizza, large thin crust $12.00:
tomato sauce, mozzarella cheese, ham, pineapple
Meat Lovers Pizza, large thin crust $12.00:
bbq sauce, mozzarella cheese, ham, salami, onion
What pizza would you like: Margarita Pizza
Your pizza: Margarita Pizza, large thin crust $12.00:
tomato sauce, mozzarella cheese, chedder cheese
Change the Pizza Base on the selected Pizza.
You must display the menu in the following format: Bases:
deep pan
thin crust
cheese crust
What base would you like: cheese crust
Your pizza: Margarita Pizza, medium cheese crust $12.82:
tomato sauce, mozzarella cheese, chedder cheese
Change the size of the Pizza/Pizza Base. You must display the menu in the following format:
What size pizza would you like (small/medium/large): small
Your pizza: Margarita Pizza, small thin crust $8.08:
tomato sauce, mozzarella cheese, chedder cheese
Add a topping from the list of available extra toppings. You must display the menu in the following format: Toppings:
tomato sauce $0.00
bbq sauce $0.00
sweet chilli sauce $0.50
chedder cheese $1.00
mozzarella cheese $1.00
fetta cheese $1.00
ham $2.00
What topping would you like to add: ham
Your pizza: Margarita Pizza, medium cheese crust $14.82:
tomato sauce, mozzarella cheese, chedder cheese, ham
Remove a topping from the Pizza. You must display the menu in the following format:
Toppings: tomato sauce
mozzarella cheese
chedder cheese
ham $2.00
pineapple $2.00
What topping would you like to remove: pineapple
Your pizza: Margarita Pizza, medium cheese crust $14.82:
tomato sauce, mozzarella cheese, chedder cheese, ham
Display the orders so far, for example:
So far you have ordered...
Spicy Seafood Pizza, small cheese crust $12.12:
sweet chilli sauce, mozzarella cheese, prawns, anchovies, jalapenos, capsicum
Meat Lovers Pizza, large thin crust $15.50:
bbq sauce, mozzarella cheese, ham, salami, onion, anchovies, fetta cheese
Save a receipt for the ordered Pizzas, for example:
Spicy Seafood Pizza, small cheese crust $12.12:
sweet chilli sauce, mozzarella cheese, prawns, anchovies, jalapenos, capsicum, onion
Meat Lovers Pizza, large thin crust $17.50:
bbq sauce, mozzarella cheese, ham, salami, onion, anchovies, fetta cheese, olives
Enjoy your meal Jane! :)
A command prompt that allows the user to navigate the Pizza Shop interface.
COMMAND PROMPT
The Command Prompt will output "Welcome to the Pizza Shop". It will then ask for the user's name and validate this to ensure it contains at least 1 word. It will then ask the user:
1. Order Pizza
2. Display orders
3. Exit
How may I help you:
While the user has not entered "3", take keyboard input for the user's choice. If the user enters invalid input, output "Please select either 1, 2, or 3". If the user enters "1", call the method to select a Pizza from the pizza menu. If the user enters an invalid pizza name, output "We do not make that kind of pizza" and return to the main menu. If the user successfully chooses a Pizza, output the submenu:
1. Change Size
2. Change Pizza Base
3. Add Topping
4. Remove Topping
5. Order
6. Cancel
What would you like to do:
While the user has not entered "5" or "6", output "Your pizza: pizza" where pizza is your selected Pizza and take keyboard input for the users choice. Call the PizzaShop method that is appropriate to the user's choice. If the user has not entered a valid choice, output "Please only enter choices 1 - 6." If the user enters "5", add the pizza to the orders and return to the main menu. If the user enters "5" or "6" return to the main menu.
At the main menu, if the user enters "2", display the Pizzas that the user has ordered so far. If the user enters "3", save the orders to a receipt file, output "Have a good day! :)" and end the program.
Your program should never crash. Ensure you handle any exceptions appropriately.
UNIT TEST
You must write a unittest or a pytest for the PizzaBase class within the "test_driver.py" file. You must write tests for PizzaBase methods. Each test must use one set of safe arguments and one set of dangerous arguments. Ensure the methods work as intended in all cases. The methods that you must test are:
clone
equals
calculate_square_inches
set_diameter
set_size_string
For both set_diameter and set_size_string, ensure the price is recalculated correctly.
SAMPLE OUTPUT
Please ensure your program matches the following output as closely as possible.
~~ Welcome to the Pizza Shop ~~
What is your name:
Please enter your name:
Please enter your name: Michael
1. Order Pizza
2. Display orders
3. Exit
How may I help you: 4
Please select either 1, 2, or 3
1. Order Pizza
2. Display orders
3. Exit
How may I help you: 1
Menu:
Margarita Pizza, large thin crust $12.00:
tomato sauce, mozzarella cheese, chedder cheese
Hawaiian Pizza, large thin crust $12.00:
tomato sauce, mozzarella cheese, ham, pineapple
Meat Lovers Pizza, large thin crust $12.00:
bbq sauce, mozzarella cheese, ham, salami, onion
Supreme Pizza, large thin crust $12.00:
tomato sauce, mozzarella cheese, ham, mushrooms, capsicum, salami, olives, pineapple
Vegetarian Pizza, large thin crust $12.00:
tomato sauce, fetta cheese, capsicum, pineapple, mushrooms, spinach, olives
BBQ Chicken Pizza, large thin crust $14.00:
bbq sauce, mozzarella cheese, chicken, onion
Spicy Seafood Pizza, large thin crust $14.00:
sweet chilli sauce, mozzarella cheese, prawns, anchovies, jalapenos, capsicum, onion
Chicken and Prawn Pizza, large thin crust $16.00:
sweet chilli sauce, fetta cheese, chicken, prawns, spinach
What pizza would you like: none
We do not make that kind of pizza
1. Order Pizza
2. Display orders
3. Exit
How may I help you: 1
Menu:
Margarita Pizza, large thin crust $12.00:
tomato sauce, mozzarella cheese, chedder cheese
Hawaiian Pizza, large thin crust $12.00:
tomato sauce, mozzarella cheese, ham, pineapple
Meat Lovers Pizza, large thin crust $12.00:
bbq sauce, mozzarella cheese, ham, salami, onion
Supreme Pizza, large thin crust $12.00:
tomato sauce, mozzarella cheese, ham, mushrooms, capsicum, salami, olives, pineapple
Vegetarian Pizza, large thin crust $12.00:
tomato sauce, fetta cheese, capsicum, pineapple, mushrooms, spinach, olives
BBQ Chicken Pizza, large thin crust $14.00:
bbq sauce, mozzarella cheese, chicken, onion
Spicy Seafood Pizza, large thin crust $14.00:
sweet chilli sauce, mozzarella cheese, prawns, anchovies, jalapenos, capsicum, onion
Chicken and Prawn Pizza, large thin crust $16.00:
sweet chilli sauce, fetta cheese, chicken, prawns, spinach
What pizza would you like: Margarita Pizza
Your pizza: Margarita Pizza, large thin crust $12.00:
tomato sauce, mozzarella cheese, chedder cheese
1. Change Size
2. Change Pizza Base
3. Add Topping
4. Remove Topping
5. Order
6. Cancel
What would you like to do: 7
Please only enter choices 1 - 6.
Your pizza: Margarita Pizza, large thin crust $12.00:
tomato sauce, mozzarella cheese, chedder cheese
1. Change Size
2. Change Pizza Base
3. Add Topping
4. Remove Topping
5. Order
6. Cancel
What would you like to do:1
What size pizza would you like (small/medium/large): small
Your pizza: Margarita Pizza, small thin crust $8.08:
tomato sauce, mozzarella cheese, chedder cheese
1. Change Size
2. Change Pizza Base
3. Add Topping
4. Remove Topping
5. Order
6. Cancel
What would you like to do: 1
What size pizza would you like (small/medium/large): medium
Your pizza: Margarita Pizza, medium thin crust $9.88:
tomato sauce, mozzarella cheese, chedder cheese
1. Change Size
2. Change Pizza Base
3. Add Topping
4. Remove Topping
5. Order
6. Cancel
What would you like to do: 1
What size pizza would you like (small/medium/large): large
Your pizza: Margarita Pizza, large thin crust $12.00:
tomato sauce, mozzarella cheese, chedder cheese
1. Change Size
2. Change Pizza Base
3. Add Topping
4. Remove Topping
5. Order
6. Cancel
What would you like to do: 1
What size pizza would you like (small/medium/large): none
Must be one of small, medium, or large
What size pizza would you like (small/medium/large): s
Must be one of small, medium, or large
What size pizza would you like (small/medium/large): medium
Your pizza: Margarita Pizza, medium thin crust $9.88:
tomato sauce, mozzarella cheese, chedder cheese
1. Change Size
2. Change Pizza Base
3. Add Topping
4. Remove Topping
5. Order
6. Cancel
What would you like to do: 2
Bases:
deep pan
thin crust
cheese crust
What base would you like: none
none is not a pizza base
Your pizza: Margarita Pizza, medium thin crust $9.88:
tomato sauce, mozzarella cheese, chedder cheese
1. Change Size
2. Change Pizza Base
3. Add Topping
4. Remove Topping
5. Order
6. Cancel
What would you like to do: 2
Bases:
deep pan
thin crust
cheese crust
What base would you like: cheese crust
Your pizza: Margarita Pizza, medium cheese crust $12.82:
tomato sauce, mozzarella cheese, chedder cheese
1. Change Size
2. Change Pizza Base
3. Add Topping
4. Remove Topping
5. Order
6. Cancel
What would you like to do: 3
Toppings:
tomato sauce $0.00
bbq sauce $0.00
sweet chilli sauce $0.50
chedder cheese $1.00
mozzarella cheese $1.00
fetta cheese $1.00
ham $2.00
salami $3.00
chicken $4.00
prawns $5.00
pineapple $2.00
onion $1.00
capsicum $1.00
jalapenos $2.50
olives $2.00
mushrooms $2.50
spinach $3.00
anchovies $2.50
What topping would you like to add: none
Could not find none
Your pizza: Margarita Pizza, medium cheese crust $12.82:
tomato sauce, mozzarella cheese, chedder cheese
1. Change Size
2. Change Pizza Base
3. Add Topping
4. Remove Topping
5. Order
6. Cancel
What would you like to do: 3
Toppings:
tomato sauce $0.00
bbq sauce $0.00
sweet chilli sauce $0.50
chedder cheese $1.00
mozzarella cheese $1.00
fetta cheese $1.00
ham $2.00
salami $3.00
chicken $4.00
prawns $5.00
pineapple $2.00
onion $1.00
capsicum $1.00
jalapenos $2.50
olives $2.00
mushrooms $2.50
spinach $3.00
anchovies $2.50
What topping would you like to add: ham
Your pizza: Margarita Pizza, medium cheese crust $14.82:
tomato sauce, mozzarella cheese, chedder cheese, ham
1. Change Size
2. Change Pizza Base
3. Add Topping
4. Remove Topping
5. Order
6. Cancel
What would you like to do: 3
Toppings:
tomato sauce $0.00
bbq sauce $0.00
sweet chilli sauce $0.50
chedder cheese $1.00
mozzarella cheese $1.00
fetta cheese $1.00
ham $2.00
salami $3.00
chicken $4.00
prawns $5.00
pineapple $2.00
onion $1.00
capsicum $1.00
jalapenos $2.50
olives $2.00
mushrooms $2.50
spinach $3.00
anchovies $2.50
What topping would you like to add: pineapple
Your pizza: Margarita Pizza, medium cheese crust $16.82:
tomato sauce, mozzarella cheese, chedder cheese, ham, pineapple
1. Change Size
2. Change Pizza Base
3. Add Topping
4. Remove Topping
5. Order
6. Cancel
What would you like to do: 4
Toppings:
tomato sauce
mozzarella cheese
chedder cheese
ham $2.00
pineapple $2.00
What topping would you like to remove: none
Could not find none
Your pizza: Margarita Pizza, medium cheese crust $16.82:
tomato sauce, mozzarella cheese, chedder cheese, ham, pineapple
1. Change Size
2. Change Pizza Base
3. Add Topping
4. Remove Topping
5. Order
6. Cancel
What would you like to do: 4
Toppings:
tomato sauce
mozzarella cheese
chedder cheese
ham $2.00
pineapple $2.00
What topping would you like to remove: pineapple
Your pizza: Margarita Pizza, medium cheese crust $14.82:
tomato sauce, mozzarella cheese, chedder cheese, ham
1. Change Size
2. Change Pizza Base
3. Add Topping
4. Remove Topping
5. Order
6. Cancel
What would you like to do: 4
Toppings:
tomato sauce'
mozzarella cheese
chedder cheese
ham $2.00
What topping would you like to remove: chedder cheese
Your pizza: Margarita Pizza, medium cheese crust $14.82:
tomato sauce, mozzarella cheese, ham
1. Change Size
2. Change Pizza Base
3. Add Topping
4. Remove Topping
5. Order
6. Cancel
What would you like to do: 5
1. Order Pizza
2. Display orders
3. Exit
How may I help you: 1
Menu:
Margarita Pizza, large thin crust $12.00:
tomato sauce, mozzarella cheese, chedder cheese
Hawaiian Pizza, large thin crust $12.00:
tomato sauce, mozzarella cheese, ham, pineapple
Meat Lovers Pizza, large thin crust $12.00:
bbq sauce, mozzarella cheese, ham, salami, onion
Supreme Pizza, large thin crust $12.00:
tomato sauce, mozzarella cheese, ham, mushrooms, capsicum, salami, olives, pineapple
Vegetarian Pizza, large thin crust $12.00:
tomato sauce, fetta cheese, capsicum, pineapple, mushrooms, spinach, olives
BBQ Chicken Pizza, large thin crust $14.00:
bbq sauce, mozzarella cheese, chicken, onion
Spicy Seafood Pizza, large thin crust $14.00:
sweet chilli sauce, mozzarella cheese, prawns, anchovies, jalapenos, capsicum, onion
Chicken and Prawn Pizza, large thin crust $16.00:
sweet chilli sauce, fetta cheese, chicken, prawns, spinach
What pizza would you like: Spicy Seafood Pizza
Your pizza: Spicy Seafood Pizza, large thin crust $14.00:
sweet chilli sauce, mozzarella cheese, prawns, anchovies, jalapenos, capsicum, onion
1. Change Size
2. Change Pizza Base
3. Add Topping
4. Remove Topping
5. Order
6. Cancel
What would you like to do: 6
1. Order Pizza
2. Display orders
3. Exit
How may I help you: 3
Have a good day! :)
Step by Step Solution
There are 3 Steps involved in it
Step: 1
The academic integrity comment should be added at the beginning of the pizzashoppy and ...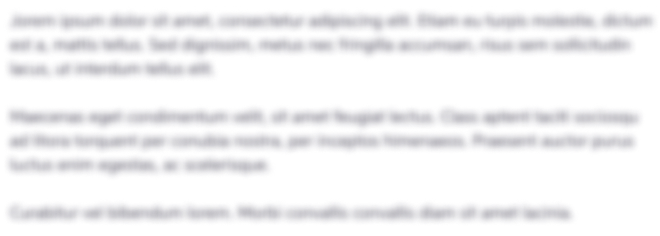
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started