Question
Taylor series is one of well-known methods to compute mathematical functions such as 1/x, log(x), exp and etc. In this homework, you are provided with
Taylor series is one of well-known methods to compute mathematical functions such as 1/x, log(x), exp and etc. In this homework, you are provided with the Verilog code of a sequential circuit that computes an approximation of reciprocal of x (i.e. 1/x) using the first n term of its Taylor expansion. For a fractional x, the following calculates 1/x:
1 (x 1) + (x 1)2 - (x 1 )3 +
= 1 + (1 x) + (1 x)2 + (1 x)3 +
Shown below is the block diagram of the module approximating 1/x. The module takes an 8- bit fractional fixed-point value on x (0 FILES: 1) DATAPATH.V `timescale 1 ns/ 100 ps module Datapath ( clk, rst, InBus, rst_xreg, load_xu, load_xl, rst_counter, load_iteration, dec_iteration, rst_temp, init_temp, load_temp, rst_resultreg, init_resultreg, load_resultreg, result, counter_zero); input clk, rst; input [3:0] InBus; input rst_xreg, load_xu, load_xl, rst_counter, load_iteration, dec_iteration, rst_temp, init_temp, load_temp, rst_resultreg, init_resultreg, load_resultreg; //come from controller part output [15:0] result; output counter_zero; //go to controller part reg [7:0] out_xreg; reg [3:0] counter; reg [7:0] out_tempreg; reg [15:0] out_resultreg; wire [15:0] mul_bus_temp; wire [7:0] complement_xreg; wire [7:0] mul_bus; wire [15:0] add_bus; //x register: storing input always @( posedge clk ) begin if( rst_xreg ) out_xreg <= 0; else if( load_xl ) out_xreg[3:0] <= InBus; else if( load_xu ) out_xreg[7:4] <= InBus; end //Counter: counting the number of iterations always @(posedge clk ) begin if( rst_counter ) counter <= 0; else if ( load_iteration ) counter <= InBus; else if( dec_iteration ) counter <= counter - 1; end assign counter_zero = (counter == 4'b0000) ? 1'b1 : 1'b0; //Complement assign complement_xreg = ~out_xreg; //Temp register: storing one of terms in Tailor series always @( posedge clk ) begin if( rst_temp ) out_tempreg <= 0; else if( init_temp ) out_tempreg <= 8'b11111111; else if( load_temp ) out_tempreg <= mul_bus; end //Result register: storing partial of result always @( posedge clk ) begin if( rst_resultreg ) out_resultreg <= 0; else if( init_resultreg ) out_resultreg <= 16'b0000000100000000; else if( load_resultreg ) out_resultreg <= add_bus; end //Multiplier //array_mult mult1 (complement_xreg, out_tempreg, mul_bus); assign mul_bus_temp = complement_xreg * out_tempreg; assign mul_bus = mul_bus_temp [15:8]; //Adder assign add_bus = out_resultreg + { 8'b00000000, out_tempreg }; assign result = out_resultreg; endmodule 2) CONTROLLER.V `timescale 1 ns/ 100 ps module Controller ( clk, rst, start, counter_zero, ready, rst_xreg, load_xu, load_xl, rst_counter, load_iteration, dec_iteration, rst_temp, init_temp, load_temp, rst_resultreg, init_resultreg, load_resultreg); input clk, rst, start; input counter_zero; //come from datapath part output ready; output rst_xreg, load_xu, load_xl, rst_counter, load_iteration, dec_iteration, rst_temp, init_temp, load_temp, rst_resultreg, init_resultreg, load_resultreg; //go to datapath part reg ready; reg rst_xreg, load_xu, load_xl, rst_counter, load_iteration, dec_iteration, rst_temp, init_temp, load_temp, rst_resultreg, init_resultreg, load_resultreg; reg [2:0] p_state, n_state; parameter [2:0] IDLE = 3'b000, RECEIVE_ITERATION = 3'b001, RECEIVE_XL = 3'b010, RECEIVE_XU = 3'b011, WAIT_ON_START = 3'b100, CALC_TERM = 3'b101, ADD_NEW_TERM = 3'b110; always @( p_state, start, counter_zero ) begin n_state = IDLE; ready = 1'b0; rst_xreg = 1'b0; load_xu = 1'b0; load_xl = 1'b0; rst_counter = 1'b0; load_iteration = 1'b0; dec_iteration = 1'b0; rst_temp = 1'b0; init_temp = 1'b0; load_temp = 1'b0; rst_resultreg = 1'b0; init_resultreg = 1'b0; load_resultreg = 1'b0; case ( p_state ) IDLE : begin ready = 1'b1; rst_xreg = 1'b1; rst_counter = 1'b1; rst_temp = 1'b1; rst_resultreg = 1'b1; if( start ) n_state = RECEIVE_ITERATION; else n_state = IDLE; end RECEIVE_ITERATION: begin load_iteration = 1'b1; init_temp = 1'b1; init_resultreg = 1'b1; n_state = RECEIVE_XL; end RECEIVE_XL: begin load_xl = 1'b1; n_state = RECEIVE_XU; end RECEIVE_XU: begin load_xu = 1'b1; n_state = WAIT_ON_START; end WAIT_ON_START: begin if( start == 1'b1 ) n_state = WAIT_ON_START; else n_state = CALC_TERM; end CALC_TERM: begin load_temp = 1'b1; dec_iteration = 1'b1; n_state = ADD_NEW_TERM; end ADD_NEW_TERM: begin load_resultreg = 1'b1; if( counter_zero ) n_state = IDLE; else n_state = CALC_TERM; end endcase end always @(posedge clk, posedge rst) begin if( rst ) p_state <= IDLE; else p_state <= n_state; end endmodule 3) ONE_OVER_X.V `timescale 1 ns/ 100 ps module one_over_x ( clk, rst, start, InBus, result, ready); input clk, rst, start; input [3:0] InBus; output [15:0] result; output ready; wire load_xu, load_xl, load_iteration, dec_iteration, init_temp, load_temp, init_resultreg, load_resultreg, counter_zero; Datapath DP ( clk, rst, InBus, rst_xreg, load_xu, load_xl, rst_counter, load_iteration, dec_iteration, rst_temp, init_temp, load_temp, rst_resultreg, init_resultreg, load_resultreg, result, counter_zero ); Controller CU ( clk, rst, start, counter_zero, ready, rst_xreg, load_xu, load_xl, rst_counter, load_iteration, dec_iteration, rst_temp, init_temp, load_temp, rst_resultreg, init_resultreg, load_resultreg ); endmodule
Step by Step Solution
There are 3 Steps involved in it
Step: 1
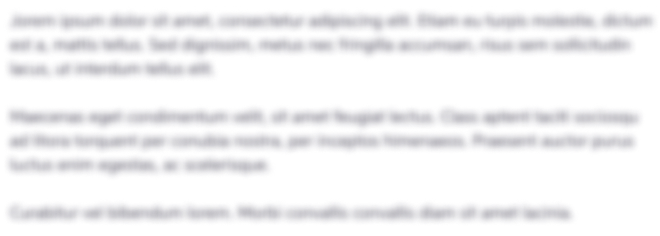
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started