Question
template class Sentinel{ struct Node{ T data_; Node* next_; Node* prev_; Node(const T& data=T{},Node* next=nullptr, Node* prev=nullptr){ data_=data; next_=next; prev_=prev; } }; Node* front_; Node*
template | |
class Sentinel{ | |
struct Node{ | |
T data_; | |
Node* next_; | |
Node* prev_; | |
Node(const T& data=T{},Node* next=nullptr, Node* prev=nullptr){ | |
data_=data; | |
next_=next; | |
prev_=prev; | |
} | |
}; | |
Node* front_; | |
Node* back_; | |
public: | |
Sentinel(){ | |
front_=new Node(); | |
back_=new Node(); | |
front_->next_=back_; | |
back_->prev_=front_; | |
} | |
void push_front(const T& data); | |
~Sentinel(); | |
class const_iterator{ | |
public: | |
const_iterator(){} | |
const_iterator& operator++(){} | |
const_iterator operator++(int){} | |
const_iterator& operator--(){} | |
const_iterator operator--(int){} | |
bool operator==(const_iterator rhs){} | |
bool operator!=(const_iterator rhs){} | |
const T& operator*()const{} | |
}; | |
class iterator:public const_iterator{ | |
public: | |
iterator(); | |
iterator& operator++(){} | |
iterator operator++(int){} | |
iterator& operator--(){} | |
iterator operator--(int){} | |
T& operator*(){} | |
const T& operator*()const{} | |
}; | |
const_iterator cbegin() const{} | |
iterator begin(){} | |
const_iterator cend() const{} | |
iterator end(){} | |
}; | |
template | |
void Sentinel | |
} | |
template | |
Sentinel | |
} |
To support this add the following functions to each of DList and Sentinel classes:
iterator begin(); const_iterator cbegin() const;
These functions returns an iterator/const_iterator to the first node in the list, end() if list is empty.
iterator end(); const_iterator cend() const;
these functions returns an iterator/const_iterator to the node just after the last node in the linked list.
The const_iterator and iterators must support the following operations:
bool operator==(const_iterator rhs); - function returns true if rhs and current object refer to the same node bool operator!=(const_iterator rhs); - function returns true if rhs and current object does not refer to the same node
iterator operator++(); //prefix ++ iterator operator++(int); //postfix ++ const_iterator operator++(); //prefix ++ const_iterator operator++(int); //postfix ++
iterator advances to next node in list if iterator is not currently at end(). The iterator returned depends if it is prefix or postfix. prefix operator returns iterator to current node. postfix operator returns iterator to node before the increment.
iterator operator--(); //prefix -- iterator operator--(int); //postfix -- const_iterator operator--(); //prefix -- const_iterator operator--(int); //postfix --
iterator refer to the node "previous" to the linked list. The iterator returned depends if it is prefix or postfix. prefix operator returns iterator to current node. postfix operator returns iterator to node before the decrement.
const T& operator*() const; //in const_iterator const T& operator*() const; //in iterator
returns a const reference to data in the node referred to by the iterator.
T& operator*();
returns a reference to data in the node referred to by the iterator.
Please implement iterator and const_iterator for function Sentinel classes in C++ code language, thank you!
Step by Step Solution
There are 3 Steps involved in it
Step: 1
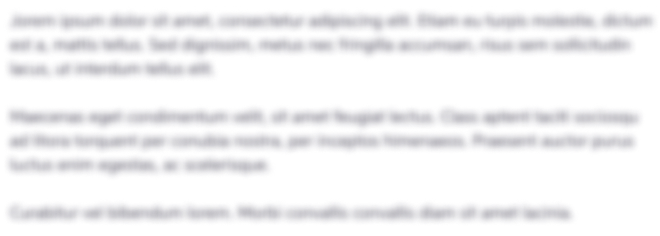
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started