Question
/** * Test Harness for Lab 04 * (c) 2019 Terri Davis * * You will make the change(s) required on the following lines: *
/** * Test Harness for Lab 04 * (c) 2019 Terri Davis * * You will make the change(s) required on the following lines: * Line 13: complete the declaration for an array to hold objects using the RingOut interface * Line 20: complete the control statement for the enhanced for loop to process the array */ public class Test_Lab04 { // Declare an array to hold objects for testing // The array must be declared as 'private static' in order to work properly private static ....... ; public static void main(String[] args) { loadArray( ); // Write the statement for the enhanced for looop here // Do not change the body of the loop for ............ /* * Make NO CHANGES to code below this block comment! */ { determineTaxStatus( oneItem ); System.out.printf( "%s", oneItem.toString( ) ); } } // end main private static void loadArray( ) { shoppingCart[0] = new Fruit( "Strawberry", 2.56, 1.33 ); shoppingCart[1] = new Vegetable( "Cucumber", 0.45, 3 ); shoppingCart[2] = new GiftCard( "Amazon", 100.00 ); shoppingCart[3] = new Fruit( "Blackberry", 4.18, 0.88 ); shoppingCart[4] = new Vegetable( "Bok Choy", 1.98, 2 ); shoppingCart[5] = new GiftCard( "Barnes & Noble", 50.00 ); } // end loadArray private static void determineTaxStatus( RingOut item ) { if( item.chkTaxable( ) ) System.out.printf( "%n%nThe next item is taxable at a rate of %.4f%%.", item.TAX_RATE ); else System.out.printf( "%n%nThe next item is not taxable." ); } } // end Test_Lab04
/** * Vegetable * (c)2019 Terri Davis * * You will make the change(s) necessary for this class to work correctly with the completed test harness */ public class Vegetable extends Produce { private double price; private int count; public Vegetable() { super( ); } public Vegetable( String type, double prc, int cnt ) { super( type ); setPrice( prc ); setCount( cnt ); } public final void setPrice( double prc ) { price = prc; } public final void setCount( int cnt ) { count = cnt; } public final double getPrice( ) { return price; } public final int getCount( ) { return count; } public String toString( ) { String taxStatus; if( chkTaxable( ) ) taxStatus = "is"; else taxStatus = "is not"; return String.format( "%s The price is $%.2f each for %d.%nExtended Price: $%.2f.", super.toString( ), getPrice( ), getCount( ), calcExtendedPrice( ) ); } }
/** * RingOut * (c) 2019 Terri Davis * * No changes should be made to this code! */ public interface RingOut { /* The following attribute will be available to all objects using this interface. We would normally want to define TAX_RATE as "final" and "static".... Interface variables in Java are ALWAYS final & static by default, so we don't have to include those specifiers. HOWEVER, it's a good idea to note that information for the sake of documentation. Also note: by default, if a variable is final, it is also essentially a CONSTANT */ double TAX_RATE = .0825; /* * DEFAULT interface methods are FULLY DEFINED/CONCRETE methods * available to ALL OBJECTS/CLASSES that use the interface. * They **may be** overridden, if needed. This default method * WILL NOT be overridden or altered in any way in any code * using the interface. */ default public boolean chkTaxable( ) { String sprClassStr = getClass( ).getSuperclass( ).toString( ); int loc = sprClassStr.indexOf( " " ); String classStr = sprClassStr.substring( loc + 1 ); if( classStr.matches( "Produce" ) ) { return false; } else { return true; } } /* * The following header defines rules for an interface method * THAT MUST be FULLY DEFINED/MADE CONCRETE in any class that * uses this interface. * The method header must be used EXACTLY AS SHOWN in those * classes using this interface - access specification, type, * and parameter list MUST BE EXACT. */ public double calcExtendedPrice( ); }
/** * Produce class * (c) 2019 Terri Davis * * You will make the change(s) necessary for this class to work correctly with the completed * test harness */ public abstract class Produce { private String product; public Produce( ) { } public Produce( String type ) { setProduct( type ); } public final void setProduct( String type ) { product = type; } public final String getProduct( ) { return product; } public String toString( ) { return String.format( "%nThe %s item is %s.", getClass( ).toString( ).substring( getClass( ).toString( ).indexOf( " " ) + 1 ), getProduct( ) ); } }
/** * GiftCard * (c) 2019 Terri Davis * * You will make the change(s) necessary for this class to work correctly with the * completed test harness */ public class GiftCard { private String provider; private double amount; public GiftCard() { } public GiftCard( String who, double howMuch ) { setProvider( who ); setAmount( howMuch ); } public final void setProvider( String who ) { provider = who; } public final void setAmount( double howMuch ) { amount = howMuch; } public final String getProvider( ) { return provider; } public final double getAmount( ) { return amount; } public String toString( ) { String taxStatus; if( chkTaxable( ) ) taxStatus = "is"; else taxStatus = "is not"; return String.format( "%nThe %s to %s has a value of $%.2f. The total cost is $%.2f, including tax.", getClass( ).toString( ).substring( getClass( ).toString( ).indexOf( " " ) + 1 ), getProvider( ), getAmount( ), calcExtendedPrice( ) ); } }
/** * Fruit * (c) 2019 Terri Davis * * You will make the change(s) necessary for this class to work correctly with the * completed test harness */ public class Fruit extends Produce { private double price; private double weight; public Fruit() { super( ); } public Fruit( String type, double prc, double wt ) { super( type ); setPrice( prc ); setWeight( wt ); } public final void setPrice( double prc ) { price = prc; } public final void setWeight( double wt ) { weight = wt; } public final double getPrice( ) { return price; } public final double getWeight( ) { return weight; } public String toString( ) { String taxStatus; if( chkTaxable( ) ) taxStatus = "is"; else taxStatus = "is not"; return String.format( "%s The price is $%.2f per pound and the amount is %.2f pounds.%nExtended Price: $%.2f.", super.toString( ), getPrice( ), getWeight( ), calcExtendedPrice( ) ); } }
Lab 04 Instructions Using an Interface General Information This lab requires you to make code changes to support implementing an interface. Review the text's material regarding interfaces before beginning Background Your users have asked you to begin building a shopping cart system for a grocery store. Right now, there aren't many classes to be included in the application, but these few classes (in unrelated hierarchies) must all be able to calculate an extended price and must all have access to the local sales tax rate. You will need to make changes in classes Produce, Fruit, Vegetable, and GiftCard to support the interface RingOut (provided to you). You will also need to add two lines of code to the test harness (Test_Lab04). Given: UML describing the classes and interface A zipped folder containing Complete code for the RingOut interface (DO NOT CHANGE THIS CODE) Starting point code for the following classes: o Produce o Fruit o Vegetable o GiftCard A test harness: Test_Lab04; you will need to make modifications to this code Expected output from the test harness Task: Make the necessary modifications Requirements RingOut The complete interface code has been provided to you. This interface includes one attribute, taxRate, which will be available to all objects implementing the interface. It also includes a default method, chkTaxable, with a concrete definition provided. The availability of default methods in interfaces is an enhancement new to SE8 of Java. You will make no changes to the interface code Produce The Produce class must be modified to use the interface RingOut. No interface methods need to be defined in this class. Fruit You will need to provide code to support the RingOut interface. Make no other changes in the class. Vegetable You will need to provide code to support the RingOut interface. Make no other changes in the class. GiftCard Modify the GiftCard class to use the RingOut interface. You will need to provide code to support the interface. Make no other changes in the class. Test_Lab04 Make the necessary changes to the test harness: 1. Declare an array to hold 6 objects of mixed types/classes implementing the RingOut interface Do not move the declaration from where it has been started. Write the enhanced for loop control statement to process the array. Do not change the body of the loop as provided 2. Testing Your test results should exactly match those provided. Submitting Your Work Create a zipped folder containing five (5) source modules: Produce.java Fruit.java Vegetable.java .GiftCard.java Test Labe4.java Expected Output The next item is not taxable. The Fruit item is Strawberry. The price is $2.56 per pound and the amount is 1.33 pounds. Extended Price: $3.40 The next item is not taxable. The Vegetable item is Cucumber. The price is $0.45 each for 3 Extended Price: $1.35 The next item is taxable at a rate of 0.0825% The GiftCard to Amazon has a value of $100.00. The total cost is $108.25, including tax. The next item is not taxable. The Fruit item is Blackberry. The price is $4.18 per pound and the amount is 0.88 pounds. Extended Price: $3.68. The next item is not taxable. The Vegetable item is Bok Choy. The price is $1.98 each for 2. Extended Price: $3.96. The next item is taxable at a rate of 0.0825% The GiftCard to Barnes& Noble has a value of $50.00. The total cost is $54.13, including tax
Step by Step Solution
There are 3 Steps involved in it
Step: 1
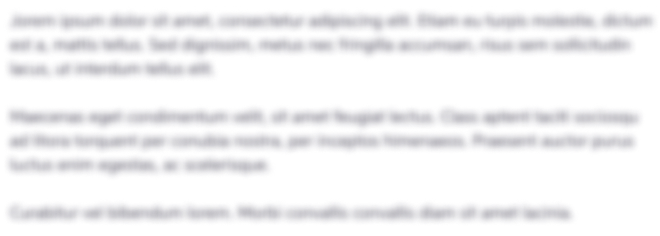
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started