Question
Test software The following program has been implemented for a newly proposed tax calculation formula for residents of a fictitious country. // incomeList[]: the array
Test software
The following program has been implemented for a newly proposed tax calculation formula for residents of a fictitious country.
// incomeList[]: the array recording the individual income items
// childList[]: the array recording the ages of children supported by this person
// parentList[]: the array recording the ages of parents supported by this person
- public double computeTax(double[] incomeList, int[] parentList, int[] childList) {
- double taxAmount = 0.0;
- double incomeAmount = 0.0;
// calculate the income amount
- for (int i = 0; i < incomeList.length; i++) {
- incomeAmount = incomeAmount + incomeList[i]; 6 }
// calculate the basic tax
- if (incomeAmount <= 40000) {
- taxAmount = incomeAmount * 0.02;
- } else if (incomeAmount > 40000 && incomeAmount <= 80000) {
- taxAmount = 800 + incomeAmount * 0.07;
- } else if (incomeAmount > 80000 && incomeAmount <= 120000) {
- taxAmount = 800 + 2800 + incomeAmount * 0.12;
- } else if (incomeAmount > 120000) {
- taxAmount = 800 + 2800 + 4800 + incomeAmount * 0.17; 15 }
// calculate the tax exemption from having children
- int taxExemption = 0;
- int numOfChild = childList.length;
- while (numOfChild > 0) {
- if (childList[numOfChild - 1] < 18) {
- taxAmount = taxAmount - 4000;
- taxExemption = taxExemption + 4000; 22 }
23 numOfChild--; 24 }
// calculate the tax exemption from having parents
- for (int j = 0; j < parentList.length; j++) {
- if (parentList[j] > 60) {
- taxAmount = taxAmount - 2000;
- taxExemption = taxExemption + 2000; 29 }
30 }
// the maximum tax exemption is 8000 each person
- if (taxExemption <= 8000) {
- if (taxAmount >= 0) {
- return taxAmount;
- } else { // i.e., taxAmount <0
- return 0; 36 }
- } else { // i.e., taxExemption > 8000
- taxAmount = taxAmount + (taxExemption - 8000);
- return taxAmount; 40 }
41}
Design test cases to achieve loop coverage on the function computeTax (list the loops covered by each test case). If it is not feasible, explain the reason. Try your best to use the minimum number of test cases to achieve the loop coverage. Consider a loop covered if at least in one test the loop body was executed 0 times, in a second test the body was executed exactly once, and in another test the body was executed more than once.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
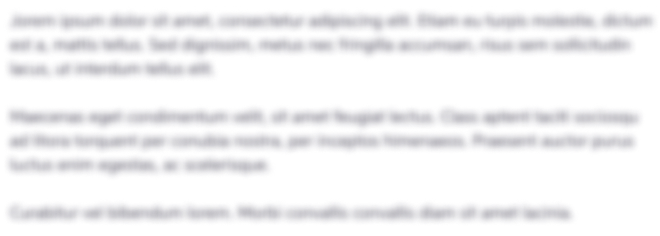
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started