Question
Tests: /* * compiling: * javac -cp .:someJunitVersion.jar Thisfile.java * * running: * java -cp .:someJunitVersion.jar Thisfile * */ import org.junit.*; import org.junit.rules.Timeout; import static
Tests:
/* * compiling: * javac -cp .:someJunitVersion.jar Thisfile.java * * running: * java -cp .:someJunitVersion.jar Thisfile * */ import org.junit.*; import org.junit.rules.Timeout; import static org.junit.Assert.*; import java.util.*; public class TileTests { public static void main(String args[]){ org.junit.runner.JUnitCore.main("TileTests"); } // 1 second max per method tested @Rule public Timeout globalTimeout = Timeout.seconds(1); public static String[] splitIcon(String lines){ return lines.split(" "); } // constructor constraints // no characters in the tile? raise the exception. @Test public void tile_01() { RuntimeException ex = null; try { Tile t = new Tile(new String[]{},1); } catch (RuntimeException e){ ex = e; if (e.getMessage().equals("empty icon")){ return; } } fail("empty icon should have raised RuntimeException."+ex); } // jagged tile icon (non-rectangular)? raise the exception. @Test public void tile_02() { RuntimeException ex = null; try { Tile t = new Tile(new String[]{"abc","def","ragged!","123"},1); } catch (RuntimeException e){ ex = e; if (e.getMessage().equals("jagged icon")){ return; } } fail("empty icon should have raised RuntimeException."+ex); } // correctly constructed Tiles should be able to report back their sizeV, sizeH, ID, and iconRows. // vertical sizes correctly calulated. @Test public void tile_03() { Tile t1 = new Tile(new String[]{"abcde","12345","_+X+_"},44); Tile t2 = new Tile(new String[]{"ABCD","EFGH","IJKL","MNOP"},99999); assertEquals(3,t1.getSizeV()); assertEquals(4,t2.getSizeV()); } // horizontal sizes correctly calculated. @Test public void tile_04() { Tile t1 = new Tile(new String[]{"abcde","12345","_+X+_"},44); Tile t2 = new Tile(new String[]{"ABCD","EFGH","IJKL","MNOP"},99999); assertEquals(5,t1.getSizeH()); assertEquals(4,t2.getSizeH()); } // ID's correctly reported. @Test public void tile_05() { Tile t1 = new Tile(new String[]{"abcde","12345","_+X+_"},44); Tile t2 = new Tile(new String[]{"ABCD","EFGH","IJKL","MNOP"},99999); assertEquals(44,t1.getId()); assertEquals(99999,t2.getId()); } // getting icon rows should work. @Test public void tile_06() { Tile t1 = new Tile(new String[]{"abcde","12345","_+X+_"},44); Tile t2 = new Tile(new String[]{"ABCD","EFGH","IJKL","MNOP"},44); assertEquals("abcde",t1.getIconRow(0)); assertEquals("12345",t1.getIconRow(1)); assertEquals("_+X+_",t1.getIconRow(2)); assertEquals("ABCD",t2.getIconRow(0)); assertEquals("EFGH",t2.getIconRow(1)); assertEquals("IJKL",t2.getIconRow(2)); assertEquals("MNOP",t2.getIconRow(3)); } // resetIcon should reject candidate icons that don't preserve the number of rows. @Test public void tile_07() { Tile t1 = new Tile(new String[]{"A234","B234","C234"},7); String[] newIcon = {"1234","1234","1234","1234"}; // too many rows boolean got = t1.resetIcon(newIcon); assertEquals(false,got); // check that no changes occurred. assertEquals("A234",t1.getIconRow(0)); assertEquals("B234",t1.getIconRow(1)); assertEquals("C234",t1.getIconRow(2)); }
// resetIcon should reject candidate icons that don't preserve the number of columns. @Test public void tile_08() { Tile t1 = new Tile(new String[]{"A234","B234","C234"},7); String[] newIcon = {"1234567","1234567","1234567"}; // too many columns boolean got = t1.resetIcon(newIcon); assertEquals(false,got); // check that no changes occurred. assertEquals("A234",t1.getIconRow(0)); assertEquals("B234",t1.getIconRow(1)); assertEquals("C234",t1.getIconRow(2)); } // resetIcon should reject candidate icons that aren't rectangular. @Test public void tile_09() { Tile t1 = new Tile(new String[]{"A234","B234","C234"},7); String[] newIcon = {"1234","too long in the middle","1234"}; // jagged. boolean got = t1.resetIcon(newIcon); assertEquals(false,got); // check that no changes occurred. assertEquals("A234",t1.getIconRow(0)); assertEquals("B234",t1.getIconRow(1)); assertEquals("C234",t1.getIconRow(2)); } // resetIcon should work when the same size is given! @Test public void tile_10() { Tile t1 = new Tile(new String[]{"A2345","B2345","C2345"},7); String[] newIcon = {"TACO1","TACO2","YUMMY"}; // jagged. boolean got = t1.resetIcon(newIcon); assertEquals(true,got); // check that no changes occurred. assertEquals("TACO1",t1.getIconRow(0)); assertEquals("TACO2",t1.getIconRow(1)); assertEquals("YUMMY",t1.getIconRow(2)); } // toString should combine rows with newlines between (but not after). @Test public void tile_11() { Tile t1 = new Tile(new String[]{"A2345","B2345","C2345"},13); assertEquals("A2345 B2345 C2345",t1.toString()); Tile t2 = new Tile(new String[]{"ABCD","EFGH","IJKL","MNOP"},44); assertEquals("ABCD EFGH IJKL MNOP",t2.toString()); } // equals vs non-Tiles should return false. @Test public void tile_12() { // none of these are comparing against a Tile, so all should be false equality checks. assertNotEquals(new Tile(new String[]{"A2345","B2345","C2345"},13),null); assertNotEquals(new Tile(new String[]{"A2345","B2345","C2345"},13),"not a tile"); assertNotEquals(new Tile(new String[]{"A2345","B2345","C2345"},13),"A2345 B2345 C2345"); assertEquals("A2345 B2345 C2345",new Tile(new String[]{"A2345","B2345","C2345"},13).toString()); } // equals vs Tiles with different icons should return false. @Test public void tile_13() { assertNotEquals(new Tile(new String[]{"A2345","B2345","C2345"},13),new Tile(new String[]{"111111","222222","333333","444444"},13)); assertNotEquals(new Tile(new String[]{"A2345","B2345","C2345"},13),new Tile(new String[]{"111","222","333","444"},13)); assertNotEquals(new Tile(new String[]{"A2345","B2345","C2345"},1),new Tile(new String[]{"A2345","B2345","C2345"},99)); assertEquals("A2345 B2345 C2345",new Tile(new String[]{"A2345","B2345","C2345"},13).toString()); } // equals of same tiles should work! @Test public void tile_14() { assertEquals(new Tile(new String[]{"A2345","B2345","C2345"},13), new Tile(new String[]{"A2345","B2345","C2345"},13) ); assertEquals(new Tile(new String[]{"111","222","333","444"},13), new Tile(new String[]{"111","222","333","444"},13) ); } }
class Tile A Tile represents one slidable piece of the puzzle. It has its visual representation as well as its identification fields . private String[] icon. This string[] stores a 2D group of characters that, when printed on separate lines, gives our visual representation of the piece * private int id. This is a unique int for cach Tile, which is the only thing that actually identifies if a tile is in its correct place Manual Inspection Criteria (5%): all fields are private. methods public Tile(stringtl icon, int id). Basic constructor. Enforces that the icon is rectangular and has at least one character in it. When that's not the case, it must do the appropriate action of these: throw new RuntimeException ("empty icon") throw new RuntimeException("jagged icon" ) . public int getsizev(). Returns the number of rows (vertical height) of the tile Public int getsizeH ( ) . Returns the number of columns (horizontal width) of the tile. public int getid). Returns a copy of id. . public string getIconRow (int i) . Returns a copy of row i from icon. (Remember, row o is the top when we print it out). public boolean resetIcon(stringtl icon). The only way to change the icon is to give a completely new stringt 1. It must enforce that the same dimensions are preserved from the old to the new value, and only make any changes when that is the case. It returns a boolean indicating whether it made changes or not. eoverride public string tostring). Builds and returns the multi-line string that is the icon's contents joined together with newline characters. (Its last character is not a newline; only add newline characters between rows of the icon) eoverride public boolean equals (Object other), when other is also a Tile, and id's match, and every single character in the icons also matches, this returns true (otherwise, false)Step by Step Solution
There are 3 Steps involved in it
Step: 1
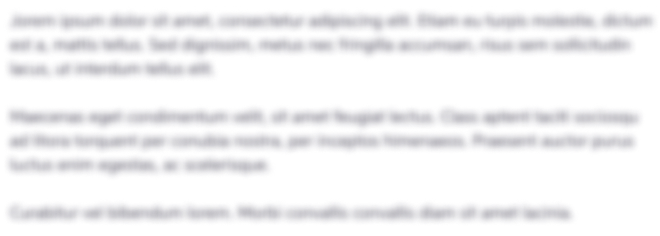
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started