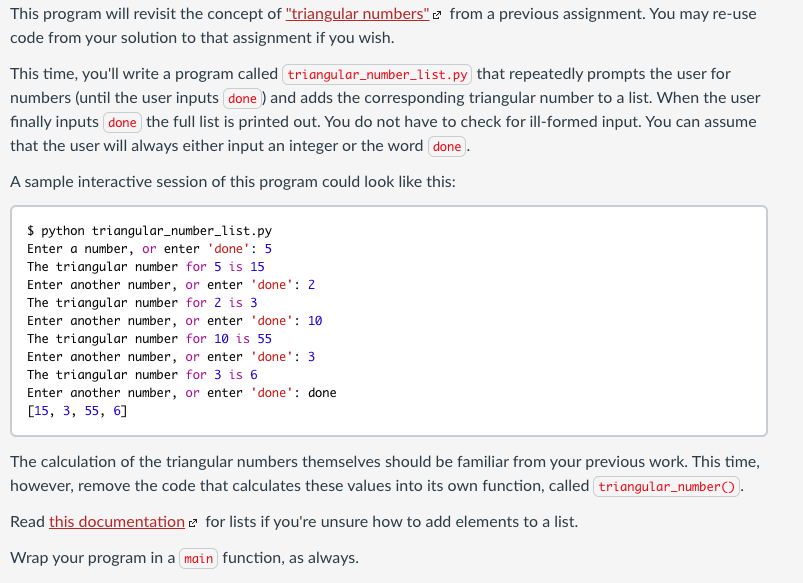
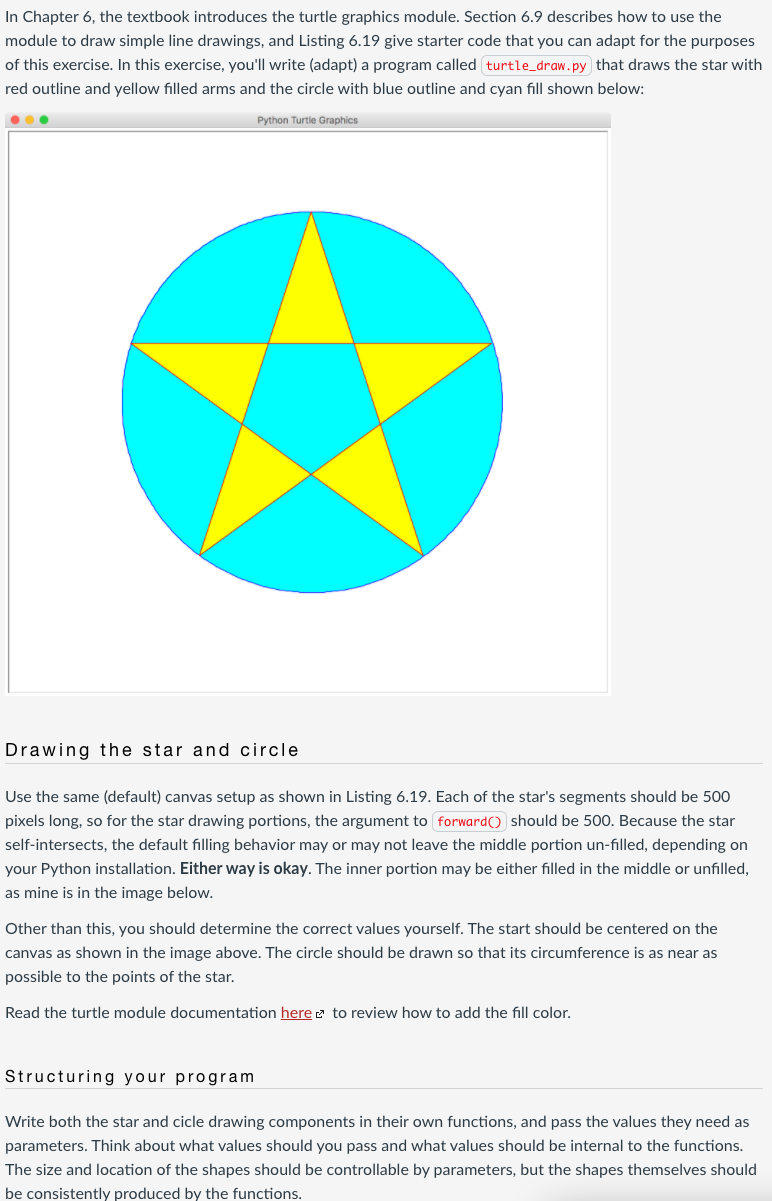
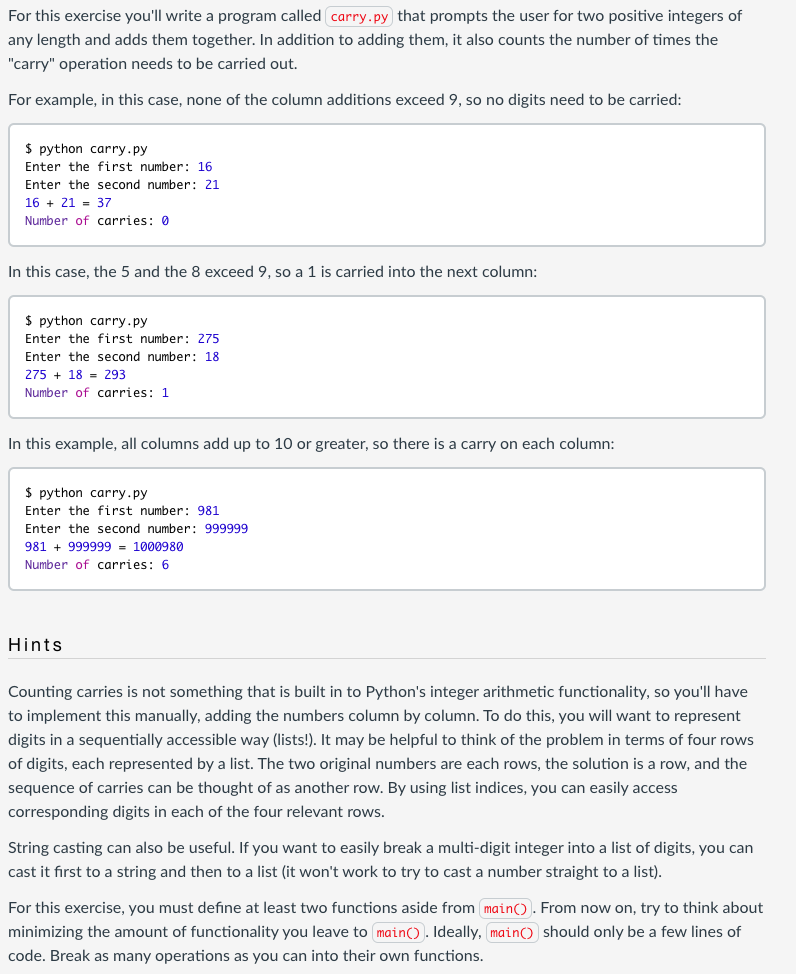
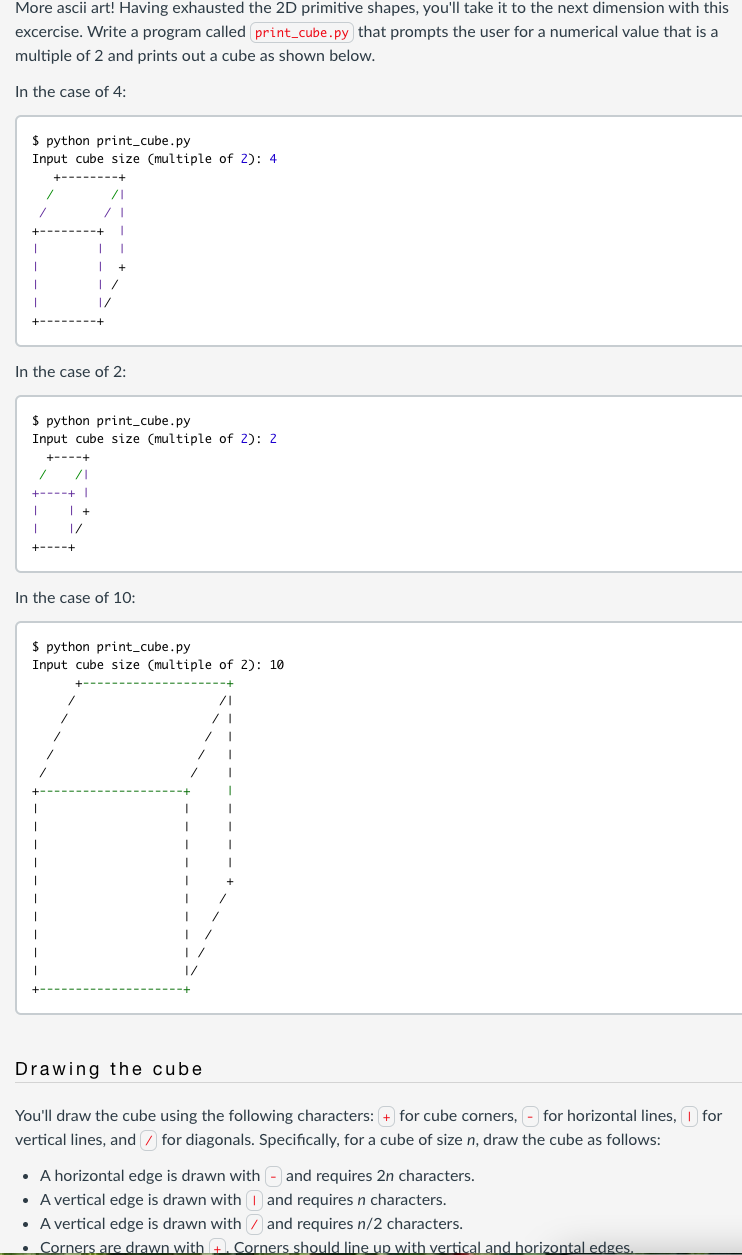
Thank you
This program will revisit the concept of "triangular numbers" from a previous assignment. You may re-use code from your solution to that assignment if you wish. This time, you'll write a program called triangular_number_list.py that repeatedly prompts the user for numbers (until the user inputs done ) and adds the corresponding triangular number to a list. When the user finally inputs done the full list is printed out. You do not have to check for ill-formed input. You can assume that the user will always either input an integer or the word done. A sample interactive session of this program could look like this: $ python triangular_number_list.py Enter a number, or enter 'done' : 5 The triangular number for 5 is 15 Enter another number, or enter 'done': 2 The triangular number for 2 is 3 Enter another number, or enter 'done': 10 The triangular number for 10 is 55 Enter another number, or enter 'done': 3 The triangular number for 3 is 6 Enter another number, or enter 'done': done [15, 3, 55, 6] The calculation of the triangular numbers themselves should be familiar from your previous work. This time, however, remove the code that calculates these values into its own function, called triangular_number(). Read this documentation e for lists if you're unsure how to add elements to a list. Wrap your program in a main function, as always. In Chapter 6, the textbook introduces the turtle graphics module. Section 6.9 describes how to use the module to draw simple line drawings, and Listing 6.19 give starter code that you can adapt for the purposes of this exercise. In this exercise, you'll write (adapt) a program called turtle_draw.py that draws the star with red outline and yellow filled arms and the circle with blue outline and cyan fill shown below: Python Turtle Graphics Drawing the star and circle Use the same (default) canvas setup as shown in Listing 6.19. Each of the star's segments should be 500 pixels long, so for the star drawing portions, the argument to forward should be 500. Because the star self-intersects, the default filling behavior may or may not leave the middle portion un-filled, depending on your Python installation. Either way is okay. The inner portion may be either filled in the middle or unfilled, as mine is in the image below. Other than this, you should determine the correct values yourself. The start should be centered on the canvas as shown in the image above. The circle should be drawn so that its circumference is as near as possible to the points of the star. Read the turtle module documentation here e to review how to add the fill color. Structuring your program Write both the star and cicle drawing components in their own functions, and pass the values they need as parameters. Think about what values should you pass and what values should be internal to the functions. The size and location of the shapes should be controllable by parameters, but the shapes themselves should be consistently produced by the functions. For this exercise you'll write a program called carry.py that prompts the user for two positive integers of any length and adds them together. In addition to adding them, it also counts the number of times the "carry" operation needs to be carried out. For example, in this case, none of the column additions exceed 9, so no digits need to be carried: $ python carry.py Enter the first number: 16 Enter the second number: 21 16 + 21 = 37 Number of carries: 0 In this case, the 5 and the 8 exceed 9, so a 1 is carried into the next column: $ python carry.py Enter the first number: 275 Enter the second number: 18 275 + 18 = 293 Number of carries: 1 In this example, all columns add up to 10 or greater, so there is a carry on each column: $ python carry.py Enter the first number: 981 Enter the second number: 999999 981 + 999999 = 1000980 Number of carries: 6 Hints Counting carries is not something that is built in to Python's integer arithmetic functionality, so you'll have to implement this manually, adding the numbers column by column. To do this, you will want to represent digits in a sequentially accessible way (lists!). It may be helpful to think of the problem in terms of four rows of digits, each represented by a list. The two original numbers are each rows, the solution is a row, and the sequence of carries can be thought of as another row. By using list indices, you can easily access corresponding digits in each of the four relevant rows. String casting can also be useful. If you want to easily break a multi-digit integer into a list of digits, you can cast it first to a string and then to a list (it won't work to try to cast a number straight to a list). For this exercise, you must define at least two functions aside from maino). From now on, try to think about minimizing the amount of functionality you leave to main() . Ideally, main should only be a few lines of code. Break as many operations as you can into their own functions. More ascii art! Having exhausted the 2D primitive shapes, you'll take it to the next dimension with this excercise. Write a program called print_cube.py that prompts the user for a numerical value that is a multiple of 2 and prints out a cube as shown below. In the case of 4: $ python print_cube.py Input cube size (multiple of 2): 4 1 1 1 1 1/ In the case of 2: $ python print_cube.py Input cube size (multiple of 2): 2 +----+ / +----+ | 1 | + I 14 In the case of 10: $ python print_cube.py Input cube size (multiple of 2): 10 I 1 1 1 1 Drawing the cube You'll draw the cube using the following characters: + for cube corners, - for horizontal lines, I for vertical lines, and for diagonals. Specifically, for a cube of size n, draw the cube as follows: A horizontal edge is drawn with and requires 2n characters. A vertical edge is drawn with and requires n characters. A vertical edge is drawn with and requires n/2 characters. Corners are drawn with +. Corners should line up with vertical and horizontal edges. Use at least one function defined in addition to maino. From now on, try to think about minimizing the amount of functionality you leave to main() . Ideally, main() should only be a few lines of code