Question
THANKS Question 1 Where is the storage for the hello string literal allocated in memory when this program is run? #include int main(void) { char
THANKS
Question 1
Where is the storage for the "hello" string literal allocated in memory when this program is run?
#include int main(void) { char * p = "hello"; printf("%s ", p); return 0; }
Question 1 options:
A | No memory is allocated to "hello". |
B | On the heap. |
C | Somewhere else. |
D | On the stack. |
Question 2
Where is the memory for array s allocated?
#include int main(void) { char s[] = "hello"; printf("%s ", s); return 0; }
Question 2 options:
A | On the stack. |
B | Somewhere else. |
C | On the heap. |
Question 3
Is this initialization correct?
char c = "b";
Question 3 options:
A | True |
B | False |
Question 4
What does the following code print?
#include int main(void) { char c = '0'; if (c) printf("yes "); else printf("no "); return 0; }
Question 4 options:
A | None of the above. |
B | It prints "no." |
C | It crashes. |
D | It does not compile. |
E | It prints "yes." |
F | The standard does not define what the program should print. |
Question 5
What does the following code print (on a 32-bit machine)?
#include void printSize(char * p) { printf("%zu ", sizeof(p)); } int main(void) { char * p = NULL; printSize(p); return 0; }
Question 5 options:
A | It crashes. |
B | It does not compile. |
C | 1 |
D | 4 |
Question 6
What's the value of sizeof(char)?
Question 6 options:
A | 8 |
B | It depends on the compiler and operating system, but it's at least 8. |
C | 1 |
D | It's either 4 on a 32-bit machine or 8 a 64-bit machine. |
Question 7
What happens when you compile and run this program? (To print the return value you can use echo $? in the command-line window right after running the program.)
#include int main(void) { char c = NULL; return c != '\0'; }
Question 7 options:
A | It returns -1. |
B | It compiles just fine, even with -Wall -Wextra. |
C | It produces a compilation error. |
D | It crashes. |
E | It produces a compilation warning, but then it returns 0. |
Question 8
Consider the following program.
/* Return the first character of a string. * If passed a NULL pointer or an empty * string, return '\0'. */ char firstChar(char const * str) { if (!str) return '\0'; return *str; }
Does it handle the empty string input according to the specification?
Question 8 options:
A | True |
B | False |
Question 9
What does the following program print when executed?
#include int main(void) { char c[] = "3G"; c[0]++; printf("%s ", c); return 0; }
Question 9 options:
A | 4G |
B | G |
C | It prints nothing because it crashes when it tries to modify read-only memory. |
D | 3H |
Question 10
What does the following program print when run?
#include int main(void) { char str[] = "complement"; char * p = str; p[sizeof(str)-6] = 'i'; printf("That's quite a %s! ", str); return 0; }
Question 10 options:
A | That's quite a compiement! |
B | That's quite a complement! |
C | The program does not compile. |
D | That's quite a compliment! |
Step by Step Solution
There are 3 Steps involved in it
Step: 1
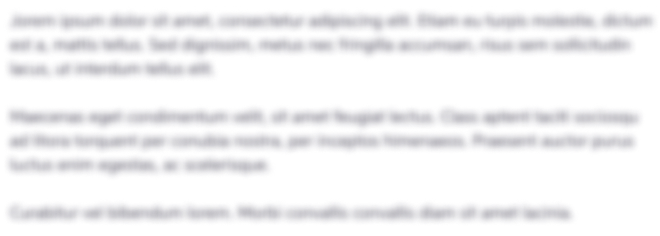
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started