Question
That is a c++ data structure question. I have 3 folders which are stackADT.h , linkedStack.h and main.cpp . In these 3 files, the places
That is a c++ data structure question. I have 3 folders which are stackADT.h, linkedStack.h and main.cpp. In these 3 files, the places that need to be filled in bold letters
You are given two header files called stackADT.h and linkedStack.h which are used in order to implement a stack structure by using a linked list. You will use the help of this linked-list type stacks in order to write a program which will convert decimal numbers into binary numbers. Program will ask the user to enter a decimal number. The general algorithm you are expected to implement by using the given linked stack structure is as follows; After the number is entered, program will push that numbers mod result by 2 into the stack and the number will be divided by 2. This will continue until the number is greater than 0 and when the number becomes 0, you will terminate the loop and print the binary equivalent of that decimal number in parallel. The remainders which are stored in the stack will be popped in a last in first out order this operation will show the binary representation of the desired number. In the program files provided, certain code snippets are removed for you to fill and specified via comment columns. You are expected to both implement the decimal to binary conversion algorithm using the linked type stacks and fill in the necessary code implementations for the linked-stack structure. Define the necessary stack object in your main program. 1) Implement necessary code snippets in order to prevent recursively calling header files/ overlapping header files. 2) Complete the code for following functions: a) isEmptyStack(): Checks the stack whether or not its empty and returns true if it is empty, false otherwise. b) isFullStack(): Checks whether the stack is full or not. Returns true if the stack is full and returns false if it is empty. c) initializeStack(): Function for advancing the stackTop and initialize the stack accordingly. 3) Fill in the implementation of assignment operator overload. 4) Use the help of completed linked-stack structure and implement a decimal to binary conversion algorithm on your main program by using it. Create necessary objects and define a function for calling the algorithm if you need to.
Thats the stackADT.h folder template
virtual bool isEmptyStack() const = 0; //Function to determine whether the stack is empty.
virtual bool isFullStack() const = 0; //Function to determine whether the stack is full.
virtual void push(const Type& newItem) = 0; //Function to add newItem to the stack.
virtual Type top() const = 0; //Function to return the top element of the stack.
virtual void pop() = 0;//Function to remove the top element of the stack. }; /* */
That's the linkedStack.h folder. /* */
#include
#include "stackADT.h"
using namespace std;
template
template
bool isEmptyStack() const;//Function to determine whether the stack is empty.
bool isFullStack() const;//Function to determine whether the stack is full.
void initializeStack();//Function to initialize the stack to an empty state.
void push(const Type& newItem);//Function to add newItem to the stack.
Type top() const;//Function to return the top element of the stack.
void pop();//Function to remove the top element of the stack.
linkedStackType(); //Default constructor linkedStackType(const linkedStackType
~linkedStackType();//Destructor
private: nodeType
};
template
template
template
template
while (/* condition to check existance of elements in stack*/) { /* node traversal & initialization algorithm is expected here*/ } }
template
template
template
if (stackTop != NULL) { temp = stackTop; //set temp to point to the top node stackTop = stackTop->link; //advance stackTop to the next node delete temp; //delete the top node } else cout << "Cannot remove from an empty stack." << endl; }
template
if (stackTop != NULL) //if stack is not empty, make it empty initializeStack();
if (otherStack.stackTop == NULL) stackTop = NULL; else { current = otherStack.stackTop; //set current to point to the stack to be copied
stackTop = new nodeType
stackTop->info = current->info; //copy the info stackTop->link = NULL; //set the link field of the node to NULL last = stackTop; //set last to point to the node current = current->link; //set current to point to the next node
//copy the remaining stack while (current != NULL) { newNode = new nodeType
newNode->info = current->info; newNode->link = NULL; last->link = newNode; last = newNode; current = current->link; } } }
template
template
//overloading the assignment operator template
/*return*/ }
/* */
/* */ Thats the main.cpp folder
#include
using namespace std;
void testCopy(linkedStackType
int main() { /*Implement the decimal to binary conversion using the help of linked-stack*/ //Code snippets in order to test the linked-stack structure before implementing the decimal to binary algorithm*/ /* linkedStackType
//Add elements into stack stack.push(34); stack.push(43); stack.push(27);
//Use the assignment operator to copy the elements //of stack into newStack newStack = stack;
cout << "After the assignment operator, newStack: " << endl;
//Output the elements of newStack while (!newStack.isEmptyStack()) { cout << newStack.top() << endl; newStack.pop(); }
//Use the assignment operator to copy the elements //of stack into otherStack otherStack = stack;
cout << "Testing the copy constructor." << endl;
testCopy(otherStack);
cout << "After the copy constructor, otherStack: " << endl;
while (!otherStack.isEmptyStack()) { cout << otherStack.top() << endl; otherStack.pop(); }
*/
return 0; }
//Function to test the copy constructor void testCopy(linkedStackType
while (!OStack.isEmptyStack()) { cout << OStack.top() << endl; OStack.pop(); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
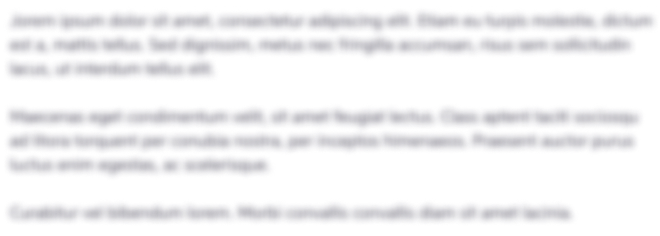
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started