Question
The A2Q1.java file simulates sequential commands sent to a large data structure. a.You will need to implement the main functions in order for it to
The A2Q1.java file simulates sequential commands sent to a large data structure.
a.You will need to implement the main functions in order for it to work.
b. Please leave the top section unchanged.
c. Edit the file below ************* YOUR CODE GOES HERE *************. There are 7 functions for you to implement:
i.initArray(): initializes the integer array.
ii.arraySize(): returns the number of valid entries held in the array.
iii.add(val): adds a new value to the array.
iv.del(val): removes a value from the array (if present).
v.sel(val): returns the position of a value in the array if present, otherwise -1.
vi.max(): returns the maximum value held in the array.
vii.min(): returns the minimum value held in the array.
d.Do not use other files or import any libraries in your implementation.
e. Your code will be judged on its correctness and performance (speed and/or memory used).
import java.util.Random;
public class A2Q1 { private final static int maxVal = 10000; public enum Command { ADD, DEL, SEL, MAX, MIN } private static int cv = Command.values().length; private static Command[] vals = Command.values(); private static Random rand = new Random(); public static Command getCom() { return vals[ rand.nextInt(cv) ]; } public static int getVal() { return rand.nextInt(maxVal); }
private final static int steps = 100 * maxVal; private static int[] arr; // store your values in this array public static void main(String[] args) { int adds = 0; // additions int sDels = 0; // successful deletions int fDels = 0; // failed deletions int sSels = 0; // successful selections int fSels = 0; // failed selections int maxs = 0; // maximum checks int mins = 0; // minimum checks long startTime = System.currentTimeMillis(); initArray(); for(int i = 0; i < steps; i++){ switch( getCom() ) { case ADD: add( getVal() ); adds++; break; case DEL: if( del( getVal() ) ) sDels++; else fDels++; break; case SEL: if( sel( getVal() ) >= 0 ) sSels++; else fSels++; break; case MAX: max(); maxs++; break; case MIN: min(); mins++; break; default: System.out.println("Command error!"); } } long executionTime = System.currentTimeMillis() - startTime; System.out.println( "Array size: " + arraySize() + " Additions: " + adds + " Successful deletions: " + sDels + " Failed deletions: " + fDels + " Successful selections: " + sSels + " Failed selections: " + fSels + " Maximum checks: " + maxs + " Minimum checks: " + mins + " Execution time: " + executionTime + "ms" ); } // ************* YOUR CODE GOES HERE ************* // 1. You need to implement all of the functions below. // 2. You may not import any libraries or use any other files. // 3. You may add other functions and variables. private static void initArray() { // TODO: Initialize the integer array (arr) here } private static int arraySize() { // TODO: return the current number of valid entries stored in arr }
private static void add(int val) { // TODO: add an integer to arr } private static boolean del(int val) { // TODO: delete an integer from arr // returns true if val was in arr, false otherwise } private static int sel(int val) { // TODO: if val is in arr, return its ordinal position, e.g. 0 if lowest // If val is not in arr, return -1 } private static int max() { // TODO: return the maximum value in arr } private static int min() { // TODO: return the minimum value in arr } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
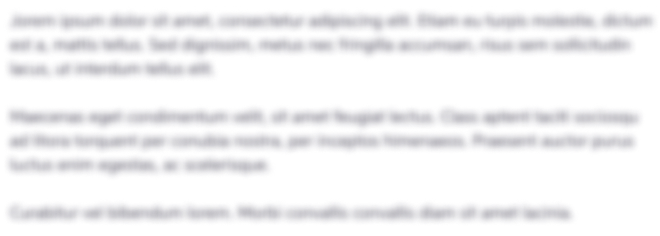
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started