Answered step by step
Verified Expert Solution
Question
1 Approved Answer
The ADT Bag is a group of items, much like what you might have with a bag of groceries. In a software development cycle, specification,




The ADT Bag is a group of items, much like what you might have with a bag of groceries. In a software development cycle, specification, design, implementation, test/debug, and documentation are typical activities. The details are provided in the rest of the document.
ADT Bag Specification: (Note: You should not change the names of the operations in your program. This should be included in an interface.) Specify operations to create an empty bag that can hold up to 50 items, put an item at the end of the list of the bag (insert(item)), remove the last item in the bag ( removeLast()), remove a random item from the bag (removeRandom(), (Note: a random item is an item at a random index.) get the index of the first occurrence of an item from the bag if it is existed (get(item)), get a reference to an item at position index( get(index)), check how many items are in the bag (size()), check to see if the bag is full (isFull()), check to see if the bag is empty( isEmpty()), and completely empty the bag (makeEmpty()).
ADT Bag Design: Complete a UML diagram to include all classes that are needed to meet the specifications. An interface class is usually defined to include all operations. A class implementing this interface provides implementation details. Exceptions should to be considered when operations are designed. Java has two types of exceptions: checked exceptions and runtime exceptions. Checked exceptions are instances of classes that are sub classes of java.lang.Exception class. They must be handled locally or explicitly thrown from the method. They are typically used when the method encounters a serious problem. In some cases, the error may be considered serious enough that the program should be terminated. Runtime exceptions occur when the error is not considered as serious. These types of exceptions can often be prevented by fail-safe programming. For example, it is fairly easy to avoid allowing an array index to go out of range, a situation that causes the runtime exception ArrayIndexOutOfBoundsException to be thrown. Runtime exceptions are instances of classes that are subclasses of the java.lang.RuntimeException class. RuntimeException is a subclass of java.lang.Exception that relaxes the requirement forcing the exception to be either handled or explicitly thrown by the method. In general, some operations of an ADT list can be provided with an index value. If the index value is out of range, an exception should be thrown. Therefore, a subclass of IndexOutOfBoundException needs to be defined. Also, an exception is needed when the list storing the items becomes full. A subclass of java.lang.RuntimeException should be defined for this erroneous situation. A full ADT bag should throw an exception when a new item is inserted.
ADT Bag Implementation: Data structure array must be used to store all items in an ADT Bag list. The element type of the array should be Object type. Implement all classes included in the design. Javadoc comments need be written during this activity.
7
Class comments must be included right above the corresponding class header. Method comments must be included right above the corresponding a method header. All comments must be written in Javadoc format. There will be no credits if comments are not complete, and/or not written in Javadoc format.
ADT Bag Test/Debug: Note: It is required to store all testing data in a file. No credit will be given if not. It is required to use decomposition design technique. No credit will be given if not. To test ADT bag design, all operations in the design must be tested. In general, an empty ADT Bag is created, and then, fill the bag list with items read from a text file, invoke any operations via the list, and always display the list after it is changed. It is not efficient to let main to do all. Method main should be very small and should be the only method in the class. It should invoke a method (for example, start) that is decomposed into more methods (for example, fillList, displayList) in a separate class. Every method should be designed as a single-minded method. For example, Class ADTBagTest contains method main; class ADTBagUtility is a helper class. Both classes are used for testing purposes.
public class ADTBagTest{
bbpublic static void main(String[] args){
ADTBagUtility.start(); } }
public class ADTBagUtility {
/**
* Creates a bag of items, and change items in the bag, and displays items.
*/
public static void start(){
ADTBagArrayBased list = new ADTBagArrayBased();
//Fill the bag.
//A Scanner object must be used to read item information from a file.
//Items need to be created before they are put into the bag.
fillList(list);
//Display items in the bag.
displayList(list);
}
/**
*Stores items into a bag.
* @param list A reference to a bag
*/
public static void fillList(ADTBagArrayBased list){
Scanner input =
//add items into the bag and/or remove items from the bag.
//All operations in ADT Bag design must be used/tested here.
}
/**
* Displays items in the bag.
* @param list A reference to a bag
*/
public static void displayList(ADTBagArrayBased list){
}
}
Note: An Item type can be designed to represent an item in the bag. A String object can be used to represent the item type in the bag.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
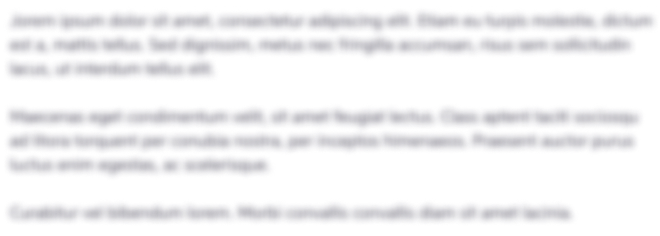
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started