Question
The answer I got for when I posted this question didn't work I tried messing around with it but it wasn't the output I needed.
The answer I got for when I posted this question didn't work I tried messing around with it but it wasn't the output I needed.
Also can you show me both the modified versions of both files and not just send or receive but both, thank you
IN C
Modify the code to allow half-duplex (simple two-way) communication by having the following interaction between the two programs: vcsend asks the user for a string and sends it to vcrec vcrec echos the received string on screen vcrec asks the user for a string and sends it to vcsend vcsend echos the received string on screen Repeat steps 1-4 until the user enters a period by itself in either vcsend (step 1) or vcrec (step When the user enters a period, close the connection, and exit both vcrec and vcsend
vcsend code:
#include
/* We must give the hostname and portnumber on the command line. This * checks that the user has typed both. */ if (argc < 3) { printf("error: Invoke this program via: "); printf(" vcsend
{ printf("enter line> "); /* Read input from user */ fgets(chrline, sizeof(chrline) - 1, stdin); /* Check for the period to end the connection and also convert any * newlines into null characters */ for (i = 0 ; i < (sizeof(chrline) - 1) ; i++) { if ( (chrline[i] == ' ') || (chrline[i] == '\0') ) break; } if (chrline[i] == ' ') chrline[i] = '\0'; /* get rid of newline */ length = strlen(chrline); if (chrline[0] == '.') /* end of stream */ { printf("sender: termination requested "); break; /* Exit out of loop */ } ret = send(msgsock, chrline, length + 1, 0); if (ret < 0) { perror("sender: write() failed. "); break; /* Exit out of loop */ } } /* Closing the data socket from vcsend will trigger recv() on vcrec to * return 0, which will also cause vcrec to close its sockets and exit */ close(msgsock); return (0); } /* end of main */
vcrec code:
#include
int size, length, ret, k; /* Process the command line for the buffer size, if given */ if (argc > 1) { size = atoi(argv[1]); /* Validate that the given argument is between 1 and sizeof(buf) - 1 * Set to the default size if argument is invalid */ if (size < 1 || size > sizeof(buf) - 1) size = sizeof(buf) - 1; } else { size = sizeof(buf) - 1; /* Default size */ } /* Create the listen socket. This is a TCP socket, so use SOCK_STREAM * Exit if the socket cannot be created */ sock = socket(AF_INET, SOCK_STREAM, 0); if (sock < 0) { perror("receiver: socket() failed. "); return (-1); } /* Bind the socket to an IP address and port. We will use the IP address * INADDR_ANY, which tells the system to assign the IP address, and the * port number 0, which tells the system to assign a random port number. * * First we have to set the fields in the sockaddr_in object "name" and then * we can call bind(). Again, exit if bind() fails. */ name.sin_family = AF_INET; /* TCP/IP family */ name.sin_addr.s_addr = INADDR_ANY; /* INADDR_ANY = assigned by system */ name.sin_port = htons(0); /* 0 = assigned by system */ ret = bind(sock,(struct sockaddr *)&name,sizeof name); if (ret < 0) { perror("receiver: bind() failed. "); return (-1); } /* In order to use vcsend to send data to this program, we need to know * what port number the system just assigned this program. So this segment * calls getsockname() to update the sockaddr_in object "name" with the * system assigned values and then print that info to the screen. */ length = sizeof name; ret = getsockname(sock, (struct sockaddr *)&name, (socklen_t *)&length); if (ret < 0) { perror("receiver: getsockname() failed. "); return (-1); } sleep(1); /* pause for clean screen display */ printf(" receiver: process id: %d ", (int)getpid()); printf(" receiver: IP address: %d.%d.%d.%d", (ntohl(name.sin_addr.s_addr) & 0xff000000) >> 24,
(ntohl(name.sin_addr.s_addr) & 0x00ff0000) >> 16, (ntohl(name.sin_addr.s_addr) & 0x0000ff00) >> 8, (ntohl(name.sin_addr.s_addr) & 0x000000ff)); printf(" receiver: port number: %hu", ntohs(name.sin_port)); printf(" "); fflush(stdout); /* Now we will call listen() and wait for a client to connect. The * accept() function will block until there is a client or an error. */ listen(sock,5); /* up to 5 clients can connect. only 1st is accepted */ k = sizeof caller; msgsock = accept(sock, (struct sockaddr *)&caller, (socklen_t *)&k); /* We only reach this point when there is an error or a client. We can * check the value of msgsock (the data socket) to see which has happened */ if (msgsock == -1) { perror("receiver: accept() failed. "); } else { printf(" receiver: Valid connection received. "); printf("receiver: Sending handshake. "); fflush(stdout); /* let vcsend know we are ready by sending a single character */ buf[0]= '0'; send(msgsock, buf, 1, 0); printf("receiver: Waiting for client.... "); do { bzero(buf,sizeof buf); /* zero buffer to remove old data */ /* recv() will block until the client sends information, the client * closes the connection or an error occurs on the data socket. */ ret = recv(msgsock, buf, size, 0); if (ret < 0) { perror("receiver: recv() failed. "); } if (ret == 0) { printf(" received-->sender has ended connection "); } else { printf(" received-->%s ",buf); } } while (ret != 0); /* Exit loop only when client ends connection */ } /* When we exit the recv() loop, the client has ended the connection, so * all that remains is closing the sockets. */ printf("receiver: ending session also and exiting. ");
close(msgsock); /* close data socket */ close(sock); /* close listen socket */ return (0); } /* end of main */
Step by Step Solution
There are 3 Steps involved in it
Step: 1
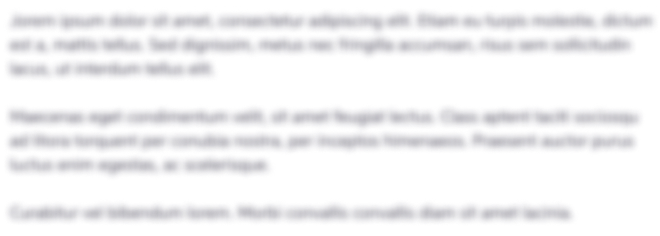
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started