Question
The assignment goal is to program a simple game winner consisting of an arbitrary number of players and staff competing against each other. The program
The assignment goal is to program a simple game winner consisting of an arbitrary number of players and staff competing against each other. The program extends Assignment 1. Each player and staff is assigned a unique numerical id and a random value between 0 and 1. The player or staff with the highest numerical value wins. Please fufill all of the requirements.
B.1 Person, Player, and Staff Classes Design three java classes, Person, Player, and Staff, stored in the package person:
Player and Staff are both subclasses of the superclass Person.
A private int data field named id stores the general Person identifier (default 0).
A private int data field named playerId stores the Player identifier (default 0).
A private int data field named staffId stores the Staff identifier (default 0).
A private double data field named val stores a random Person value (default 0).
A static totalPersonNum stores the total number of Person objects.
A static totalPlayerNum stores the total number of Player objects.
A static totalStaffNum stores the total number of Staff objects.
A printVal method prints the id and val of each Person object: System.out.println(" Person Id " + id + " from a total of " + totalPersonNum + "; Value " + val);
A printVal method in the Player class overrides the printVal method in the Person class as follows: System.out.println(" Player Id " + id + " from a total of " + totalPlayerNum);
A printVal method in the Staff class overrides the printVal method in the Person class as follows: System.out.println(" Staff Id " + id + " from a total of " + totalStaffNum);
You can assign additional methods as necessary.
B.2 Winner Class Design a Winner class, stored in the package game:
The main Java method containing the following code:
public static void main(String[] args) {
Person[] personArray = new Person[numPerson];
Winner w = new Winner(); w.init_game(personArray);
Person p = w.find_person_winner(personArray);
if (p!= null) {
System.out.println("Person Winner is: ");
p.printVal(); }
Player player = w.find_player_winner(personArray);
if (player != null) {
System.out.println("Player Winner is: ");
player.printVal(); }
Staff staff = w.find_staff_winner(personArray);
if (staff != null) {
System.out.println("Staff Winner is: ");
staff.printVal(); } }
A method called init_game where an arbitrary number of players and staff, adding up together to numPerson, gets instantiated with a sequential id number for all persons, a playerId and a staffId depending on whether they are players or staff, and a random value between 0 and 1. The person array with all their ids and values needs to be printed.
A method called find_person_winner where random values assigned to each person are compared and the person, independent on whether it is a player or staff, is the winner. Printout should include corresponding person winner id and value.
A method called find_player_winner where random values assigned to each player are compared and the player with the largest value is the winner. Printout should include corresponding player winner id and value.
A method called find_staff_winner where random values assigned to each staff are compared and the staff with the largest value is the winner. Printout should include corresponding staff winner id and value.
B.3 Program Output
The program output should be as follows:
Sample output:
Player Id 1 from a total of 4; Person Id 0 from a total of 10; Value 0.3410558546174507
Staff Id 1 from a total of 6; Person Id 1 from a total of 10; Value 0.32276652976761844
Staff Id 2 from a total of 6; Person Id 2 from a total of 10; Value 0.2843950932400461
Player Id 2 from a total of 4; Person Id 3 from a total of 10; Value 0.23059375935512438
Staff Id 3 from a total of 6; Person Id 4 from a total of 10; Value 0.40332348169310195
Player Id 3 from a total of 4; Person Id 5 from a total of 10; Value 0.9604519121200479
Player Id 4 from a total of 4; Person Id 6 from a total of 10; Value 0.2078388983133619
Staff Id 4 from a total of 6; Person Id 7 from a total of 10; Value 0.6024115515524845
Staff Id 5 from a total of 6; Person Id 8 from a total of 10; Value 0.8792031765049189
Staff Id 6 from a total of 6; Person Id 9 from a total of 10; Value 0.8501641109009124
Sample winner output (find_person_winner case):
Person Winner is: Player Id 3 from a total of 4; Person Id 5 from a total of 10; Value 0.9604519121200479
Step by Step Solution
There are 3 Steps involved in it
Step: 1
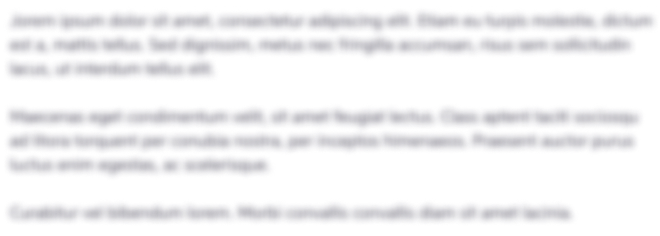
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started