Question
The assignment is very easy and includes test to see if the codes in each classes are correct also i use java (IDE netbeans it
The assignment is very easy and includes test to see if the codes in each classes are correct also i use java (IDE netbeans it really doesn't matter as long as it work perfectly fine). The classes and the test are included below with the questions.
--------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
Coordinate class//
/* * To change this license header, choose License Headers in Project Properties. * To change this template file, choose Tools | Templates * and open the template in the editor. */ package chess;
/** * * @author Oluwafunbi Aboderin */ public class Coordinate { public Coordinate(int column, int row) throws IndexOutOfBoundsException{ if ( (column7)) throw new IndexOutOfBoundsException("column must be between 0 and 7,inclusive"); if ( (row7)) throw new IndexOutOfBoundsException("row must be between 0 and 7,inclusive");}
public Coordinate(char column, char row) throws IndexOutOfBoundsException{ if ( (column'h')) throw new IndexOutOfBoundsException("column must be between a and h,inclusive"); if ( (row'8')) throw new IndexOutOfBoundsException("row must be between 1 and 8,inclusive"); }
public Coordinate(String coordinate) throws IndexOutOfBoundsException{ if (coordinate.length() != 2) throw new IllegalArgumentException ("Coordinate is a 2-character string"); char column = coordinate.charAt(0); char row = coordinate.charAt(1); // this(x,y) except it must be the first statement!\par if ( (column'h')) throw new IndexOutOfBoundsException("x must be between a and h, inclusive"); if ( (row'8')) throw new IndexOutOfBoundsException("y must be between 1 and 8, inclusive"); }
}
----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
Coordinate test//
/* * To change this license header, choose License Headers in Project Properties. * To change this template file, choose Tools | Templates * and open the template in the editor. */
import chess.Coordinate;
import org.junit.After; import org.junit.AfterClass; import org.junit.Assert; import org.junit.Before; import org.junit.BeforeClass; import org.junit.Test; import static org.junit.Assert.*;
/** * * @author Cheryl */ public class CoordinateTest { public CoordinateTest() { } // TODO add test methods here. // The methods must be annotated with annotation @Test. For example: // @Test public void testConstructorGood() { Coordinate c = new Coordinate(7,6); Assert.assertEquals(6, c.getRowNumber()); Assert.assertEquals(7, c.getColumnNumber()); } @Test public void testConstructorColumnTooHigh() { try { Coordinate c = new Coordinate(8,0); fail(); } catch (IndexOutOfBoundsException e) { /* Expected */ } } @Test public void testConstructorRowTooHigh() { try { Coordinate c = new Coordinate(0,8); fail(); } catch (IndexOutOfBoundsException e) { /* Expected */ } } @Test public void testCharConstructorGood() { Coordinate c = new Coordinate('h','7'); Assert.assertEquals('7', c.getRow()); Assert.assertEquals('h', c.getColumn()); Assert.assertEquals(6, c.getRowNumber()); Assert.assertEquals(7, c.getColumnNumber()); } @Test public void testCharConstructorColumnTooHigh() { try { Coordinate c = new Coordinate('i','1'); fail(); } catch (IndexOutOfBoundsException e) { /* Expected */ } } @Test public void testCharConstructorRowTooLow() { try { Coordinate c = new Coordinate('a','0'); fail(); } catch (IndexOutOfBoundsException e) { /* Expected */ } } @Test public void testStringConstructorGood() { Coordinate c = new Coordinate("a1"); Assert.assertEquals('a', c.getColumn()); Assert.assertEquals('1', c.getRow()); Assert.assertEquals(0, c.getColumnNumber()); Assert.assertEquals(0, c.getRowNumber()); } @Test public void testStringConstructorTooLong() { try { Coordinate c = new Coordinate("a0 "); fail(); } catch (IllegalArgumentException e) { /* Expected */ } } @Test public void testStringConstructorTooShort() { try { Coordinate c = new Coordinate("a"); fail(); } catch (IllegalArgumentException e) { /* Expected */ } } @Test public void testRowColumnLow () { Coordinate c = new Coordinate(0,0); Assert.assertEquals('a', c.getColumn()); Assert.assertEquals('1', c.getRow()); } @Test public void testRowColumnHigh () { Coordinate c = new Coordinate(7,7); Assert.assertEquals('h', c.getColumn()); Assert.assertEquals('8', c.getRow()); } @Test public void testName () { Coordinate c = new Coordinate(0,0); String s = c.name(); Assert.assertEquals("a1", s); } @Test public void testtoString () { Coordinate c = new Coordinate(0,0); String s = c.toString(); Assert.assertEquals("(0,0)", s); } }
--------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
Piece class//
/* ** Good reference on ENUMs: https://docs.oracle.com/javase/tutorial/java/javaOO/enum.html
*/ package chess;
/** * * @author Schramm */ public enum ChessPieces { PAWN ('P'), KNIGHT ('N'), BISHOP ('B'), ROOK ('R'), QUEEN ('Q'), KING ('K'); private final char shortName;
ChessPieces (char shortName) { this.shortName = shortName; } public char getShortName() { return this.shortName; } }
---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
Piece test//
/* * To change this license header, choose License Headers in Project Properties. * To change this template file, choose Tools | Templates * and open the template in the editor. */
import chess.Piece; import chess.ChessColour; import chess.ChessPieces; import chess.Square; import org.junit.Assert; import org.junit.Test; import static org.junit.Assert.*;
/** * * @author Cheryl */ public class PieceTest { public PieceTest() { }
// TODO add test methods here. // The methods must be annotated with annotation @Test. For example: // @Test public void testConstructorUnoccupiedWhite() { Piece p = new Piece ( ChessColour.WHITE, ChessPieces.KING ); Assert.assertEquals('K', p.getShortName()); Assert.assertEquals(ChessColour.WHITE, p.getColour()); Assert.assertEquals(ChessPieces.KING, p.getName()); } @Test public void testConstructorUnoccupiedBlack() { Piece p = new Piece ( ChessColour.BLACK, ChessPieces.KING ); Assert.assertEquals('k', p.getShortName()); Assert.assertEquals(ChessColour.BLACK, p.getColour()); Assert.assertEquals(ChessPieces.KING, p.getName()); } @Test public void testConstructorShortNameBlack() { Piece p = new Piece ( 'k' ); Assert.assertEquals('k', p.getShortName()); Assert.assertEquals(ChessColour.BLACK, p.getColour()); Assert.assertEquals(ChessPieces.KING, p.getName()); } @Test public void testConstructorShortNameWhite() { Piece p = new Piece ( 'K' ); Assert.assertEquals('K', p.getShortName()); Assert.assertEquals(ChessColour.WHITE, p.getColour()); Assert.assertEquals(ChessPieces.KING, p.getName()); } @Test public void testConstructorShortNameInvalid() { try { Piece p = new Piece ( 'L' ); Assert.fail(); } catch (IllegalArgumentException e) { /*Should catch */ } } @Test public void testToString() { Piece p = new Piece ( ChessColour.WHITE, ChessPieces.KING ); Assert.assertEquals("WHITE KING", p.toString()); } }
---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
Chess board class//
/* * To change this license header, choose License Headers in Project Properties. * To change this template file, choose Tools | Templates * and open the template in the editor. */ package chess;
/** * * @author Cheryl */ public class ChessBoard { private Square board[][]; private ChessColour activeColour; private int fullMove; public ChessBoard() { board = new Square[8][8]; for (int c=0; c
----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
chess board test//
/* * To change this license header, choose License Headers in Project Properties. * To change this template file, choose Tools | Templates * and open the template in the editor. */ import chess.Coordinate; import chess.Piece; import chess.Square; import org.junit.Assert;
import org.junit.Test; import static org.junit.Assert.*;
/** * * @author Cheryl */ public class SquareTest { public SquareTest() { }
// TODO add test methods here. // The methods must be annotated with annotation @Test. For example: // @Test public void testConstructorUnoccupied() { Square s = new Square ( new Coordinate(0,1) ); Assert.assertEquals(0, s.getColumnNumber()); Assert.assertEquals(1, s.getRowNumber()); Assert.assertEquals('a', s.getColumn()); Assert.assertEquals('2', s.getRow()); Assert.assertEquals(s.getPiece(), null); Assert.assertFalse("Square should be unoccupied", s.isOccupied()); } @Test public void testConstructorOccupied() { Piece p = new Piece('p'); Square s = new Square ( new Coordinate(0,1), p ); Assert.assertEquals(0, s.getColumnNumber()); Assert.assertEquals(1, s.getRowNumber()); Assert.assertEquals('a', s.getColumn()); Assert.assertEquals('2', s.getRow()); Assert.assertEquals(p, s.getPiece()); Assert.assertTrue("Square should be occupied", s.isOccupied()); } @Test public void testDeleteUnoccupied() { Square s = new Square ( new Coordinate(0,0) ); Piece p = s.deletePiece(); Assert.assertEquals(null, p); Assert.assertFalse( s.isOccupied() ); } @Test public void testDeleteOccupied() { Piece p = new Piece('p'); Square s = new Square ( new Coordinate(0,0), p ); Piece deletedPiece = s.deletePiece(); Assert.assertEquals(deletedPiece, p); Assert.assertFalse( s.isOccupied() ); } @Test public void testAddUnoccupied() { Square s = new Square ( new Coordinate(0,0) ); Piece p = new Piece('p'); Piece previousPiece = s.addPiece(p); Assert.assertEquals(null, previousPiece); Assert.assertEquals(s.getPiece(), p); Assert.assertTrue( s.isOccupied() ); } @Test public void testAddOccupied() { Piece p = new Piece('p'); Square s = new Square ( new Coordinate(0,0), p ); Piece secondPiece = new Piece('P'); Piece previousPiece = s.addPiece(secondPiece); Assert.assertEquals(p, previousPiece); Assert.assertEquals(s.getPiece(), secondPiece); Assert.assertTrue( s.isOccupied() ); } @Test public void testtoStringUnoccupied () { Square s = new Square ( new Coordinate(0,0) ); String str = s.toString(); Assert.assertEquals("Square(0,0): ", str); } @Test public void testtoStringOccupied () { Piece p = new Piece('p'); Square s = new Square ( new Coordinate(1,0), p ); String str = s.toString(); Assert.assertEquals("Square(1,0):"+p.toString(), str); } }
--------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
Instructions for the lab also please only edit the classes not the test
SYSC2004 Winter 2018 Assignment 1 Objectives Write one or more simple classes, to practice coding constructors, accessors and mutators. Write a client of these simple classes, to practice making objects and calling methods on those objects Use test-driven development to guide the writing of the simple classes. Background (Retain for use in later Assignments, as well) Our goal is to have-by the last assignment-a fully functioning chess game that you can play with your friends. In this assignment, we will work on the underlying logic of the game; in later courses, you will know this as the "model" or the "business logic". No GUls-not yet. We must all agree on the rules of our chess game. For chess aficionados, please temper your zeal and stick to the basic moves; no castling, for instance. Perhaps you will be so proud of your program at the end of term that you will continue to work on it and n all these final details! 1. Set up of the chessboard We will all use the following image as our agreed-upon setup (Citation: https:/goo.g/images/g5YtUW)and these instructions https:/www.thespruce.com/setting-up-a chess-board-611545. Note: On the image, the Pointy-Crown is Queen whilst the Round-Crown is King SYSC2004 Winter 2018 Assignment 1 Objectives Write one or more simple classes, to practice coding constructors, accessors and mutators. Write a client of these simple classes, to practice making objects and calling methods on those objects Use test-driven development to guide the writing of the simple classes. Background (Retain for use in later Assignments, as well) Our goal is to have-by the last assignment-a fully functioning chess game that you can play with your friends. In this assignment, we will work on the underlying logic of the game; in later courses, you will know this as the "model" or the "business logic". No GUls-not yet. We must all agree on the rules of our chess game. For chess aficionados, please temper your zeal and stick to the basic moves; no castling, for instance. Perhaps you will be so proud of your program at the end of term that you will continue to work on it and n all these final details! 1. Set up of the chessboard We will all use the following image as our agreed-upon setup (Citation: https:/goo.g/images/g5YtUW)and these instructions https:/www.thespruce.com/setting-up-a chess-board-611545. Note: On the image, the Pointy-Crown is Queen whilst the Round-Crown is King
Step by Step Solution
There are 3 Steps involved in it
Step: 1
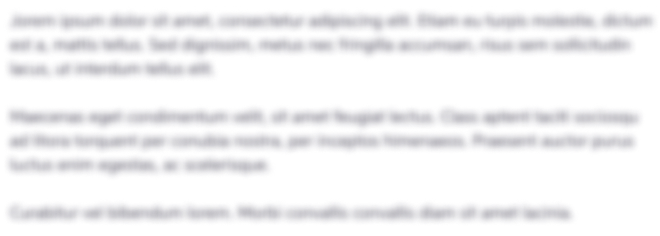
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started