Question
The assignment requires me to do the following: Create a website using HTML5 and Display a twisted triangle -You may set all parameters in the
The assignment requires me to do the following:
Create a website using HTML5 and Display a twisted triangle
-You may set all parameters in the JS file
Parameters include: vertices of triangle, amount of twist, the degree of tessellation
Optional: The use of buttons, menus or sliders to set parameters and twist other shapes
So far I have the following code created. I am having issues because the triangle won't appear. I get an error saying "Uncaught RefrenceError:getWebGLContext is not defined". I am using the most updated version of chrome btw. How can I edit my code to make it work? Please respond with the changes I should make as well as with a screenshot of the result after fixing the code.
Code:
Oops ... your browser doesn't support the HTML5 canvas element
"use strict";
var points = []; //empty array, we put the points here as we do the subdivision
var tessellationGrade;
var angle;
var renderType;
var vertices;
var radios;
window.onload = function init()
{
var canvas = document.getElementById( "gl-canvas" );
var gl = WebGLUtils.setupWebGL(canvas);
if ( !gl ) { alert( "WebGL isn't available" ); }
initVars();
initUI();
//tessellation
tessellation(vertices[0], vertices[1], vertices[2], tessellationGrade);
// Configure WebGL
gl.viewport( 0, 0, canvas.width, canvas.height );
gl.clearColor( 0.0, 0.0, 0.0, 1.0 );
// Load shaders and initialize attribute buffers
var program = initShaders( gl, "vertex-shader", "fragment-shader" );
gl.useProgram( program );
// Load the data into the GPU
var bufferId = gl.createBuffer();
gl.bindBuffer( gl.ARRAY_BUFFER, bufferId );
gl.bufferData( gl.ARRAY_BUFFER, flatten(points), gl.STATIC_DRAW );
// Associate out shader variables with our data buffer
var vPosition = gl.getAttribLocation( program, "vPosition" );
gl.vertexAttribPointer( vPosition, 2, gl.FLOAT, false, 0, 0 );
gl.enableVertexAttribArray( vPosition );
render();
};
function initVars(){
vertices = [
vec2(-0.61,-0.35), // left-down corner
vec2( 0, 0.7), // center-up corner
vec2( 0.61, -0.35) // right-down corner
];
// Twist Angle
var angleValue = document.getElementById("slider-angle").value;
angle = angleValue * Math.PI / 180;
//Render Type
radios = document.getElementsByName('render-type');
for (var i = 0, length = radios.length; i
if (radios[i].checked) {
renderType = radios[i].value;
break;
}
}
//Tessellation Grade
tessellationGrade = document.getElementById("slider-tessellation").value;
}
function initUI(){
// Twist UI
document.getElementById("slider-angle").addEventListener("input", function(e){
angle = this.value * Math.PI / 180;
rerender();
}, false);
// Render Type UI
for (var i = 0, length = radios.length; i
radios[i].onclick = function() {
renderType = this.value;
rerender();
};
}
// Tessellation Grade UI
document.getElementById("slider-tessellation").addEventListener("input", function(e){
tessellationGrade = this.value;
rerender();
}, false);
}
function twist(vector){
var x = vector[0],
y = vector[1],
d = Math.sqrt(x * x + y * y),
sinAngle = Math.sin(d * angle),
cosAngle = Math.cos(d * angle);
/*
x' = x cos(d0) - y sin(d0)
y' = x sin(d0) + y cos(d0)
*/
return [x * cosAngle - y * sinAngle, x * sinAngle + y * cosAngle];
}
function triangle (a, b, c){
a = twist(a), b = twist(b), c = twist(c);
if(renderType=="TRIANGLES"){
points.push(a, b, c);
} else {
points.push(a, b);
points.push(b, c);
points.push(a, c);
}
}
function tessellation(a, b, c, count){
if(count===0){
//when we are at the end of the recursion we push
//the triangles to the array
triangle(a,b,c);
} else {
//bisect the sides
var ab = mix( a, b, 0.5 );
var ac = mix( a, c, 0.5 );
var bc = mix( b, c, 0.5 );
--count;
/ew triangles
tessellation(a, ab, ac, count);
tessellation(c, ac, bc, count);
tessellation(b, bc, ab, count);
tessellation(ac, bc, ab, count);
}
}
function rerender(){
points = [];
tessellation(vertices[0], vertices[1], vertices[2], tessellationGrade);
gl.bufferData( gl.ARRAY_BUFFER, flatten(points), gl.STATIC_DRAW );
render();
}
function render() {
gl.clear( gl.COLOR_BUFFER_BIT );
if(renderType=="TRIANGLES"){
gl.drawArrays( gl.TRIANGLES, 0, points.length );
}
else {
gl.drawArrays( gl.LINES, 0, points.length );
}
}
Photo of result:
Step by Step Solution
There are 3 Steps involved in it
Step: 1
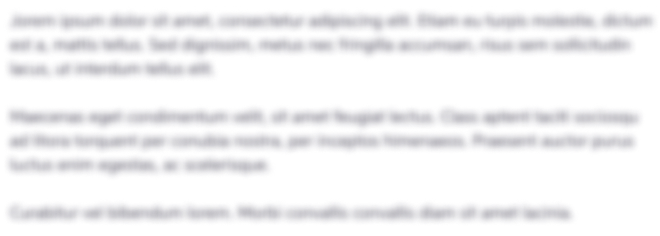
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started