Question
THE CODE IS IN JAVA Lab 10: ArrayLists and Files in a GUI Application For this lab, you will work on a simple GUI application.
THE CODE IS IN JAVA
Lab 10: ArrayLists and Files in a GUI Application
For this lab, you will work on a simple GUI application. The starting point for your work consists of four files (TextCollage, DrawTextItem, DrawTextPanel, and SimpleFileChooser) in the code directory. These files are supposed to be in package named "textcollage". Start an Eclipse project, create a package namedtextcollage in that project, and copy the four files into the package. To run the program, you should run the file TextCollage.java, which contains the main routine. You will need to look through the DrawTextItem.java and SimpleFileChooser.java, but the only file that you have to edit is DrawTextPanel.java.
Part 1: ArrayLists
You can run TextCollage to see what it does. As it stands, you can click the drawing area to place a string on the canvas. The text of the string comes from the input box at the bottom of the window. The program only has support for one string. If you click the mouse again, the previous string disappears, and the string appears at the location of the new mouse click. A string in the program is represented by a variable of type DrawTextItem. In addition to the string itself, aDrawTextItem holds information about the appearance of the string, such as its color, font, background color, rotation, and whether it has a border. (The doMousePress method, where strings are created, has some lines that you can uncomment to experiment with these options.) Your first job is to replace the variable theString, which is a single DrawTextItem, with a variable of typeArrayList that can hold any number of text items. You will then have to modify the program wherever theString was used. For example, in thedoMousePress method, you should add the newly created DrawTextItem variable to the arraylist, instead of assigning it to theString. When you have finished, you should be able to add multiple strings to the picture, and the "Undo" and "Clear" commands in the "Edit" menu should work. (The "Save Image" command in the "File" menu and the commands in the "Options" menu also work, but they were already working before you made any changes.)
Part 2: Files
The program is already able to save the contents of the drawing area as an image. But when you do this, there is no way to go back and edit the strings in the picture. To be able to do that, you should save all the information that defines the strings, not just the image where the strings are displayed. That's what the "Save" and "Open" commands are for in the "File" menu. The "Save" command should create a text file that describes the contents of the image (that is, the background color and the arraylist of DrawTextItems). The "Open" command should be able to read a file created by the "Save" command and restore the state of the program. You can look at the implementation of the "Save Image" command for some hints. Note in particular the use of try..catch to catch any exceptions that occur during the file manipulation; you should always do something similar when you work with files, and you should report any errors to the user. First, implement the "Save" command. Use the method fileChooser.getOutputFile to get a File from the user. (fileChooser is a varialble of type SimpleFileChooser, which is already defined. See the implementation of "Save Image" for an example of using it.) Create a PrintWriter to write to the file. Output a text representation of the background color of the image, then write at least the text and color of each DrawTextItem in the arraylist. Don't forget to close the PrintWriter. Note that you can represent a color c as the three integers c.getRed(), c.getGreen(), and c.getBlue(). Design the format for your output file so that it will be easy to read the data in later. Putting every output value on a separate line is the easiest approach. Test your work by saving a file and looking at its contents. Next, you can implement the "Open" command. Use the method fileChooser.getInputFile to let the user select a file for input. Create a Scanner to read from the file. You should use the scanner to read the contents of the file back into the program and reconstruct the picture represented by the contents of the file. Of course, this will only succeed if the file is one that was previously saved from the program using the Save command (or if it follows exactly the same syntax as such a file). Note: As long as each item is on its own line in the file, you can use scanner.nextLine() to read items from the file. If you are trying to read an integer, use Integer.parseInt(scanner.nextLine()). You should know the order in which the data items were written, and you should read them in the same order. If any error occurs, it means that the file is not of the correct form. Don't forget to call canvas.repaint() at the end, to make the new image visible. Ideally, if an error does occur, you should not change the current contents of the image.
Part 3: Improve the program!
To complete your program, you should design at least one additional feature and add it to the program. You should consult with your course advisor about what would be appropriate and how to do it. Grading will be based in part on creativity, ambition, and degree of consultation. You will probably need to extend your file format to accommodate the new features. For example, you could add new options to control the appearance of strings. The DrawTextItem class, which is used to represent the strings that are drawn in the picture, has a variety of properties that can be applied to the strings. Some examples can be found, commented out, in the doMousePressed() method in the DrawTextPanel class. Another possibility would be to allow the user to drag text items around on the screen, after they have been placed. Or, you could add the ability to create a random text collage using a bunch of strings selected at random from a text file specified by the user.
BELOW ARE THE VARIOUS CLASSES
package textcollage;
import java.awt.BorderLayout;
import java.awt.Color;
import java.awt.Dimension;
import java.awt.Font;
import java.awt.Graphics;
import java.awt.Graphics2D;
import java.awt.RenderingHints;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.MouseAdapter;
import java.awt.event.MouseEvent;
import java.awt.image.BufferedImage;
import java.io.File;
import java.util.ArrayList;
import javax.imageio.ImageIO;
import javax.swing.BorderFactory;
import javax.swing.JColorChooser;
import javax.swing.JLabel;
import javax.swing.JMenu;
import javax.swing.JMenuBar;
import javax.swing.JMenuItem;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
import javax.swing.JTextField;
import javax.swing.KeyStroke;
/**
* A panel that contains a large drawing area where strings
* can be drawn. The strings are represented by objects of
* type DrawTextItem. An input box under the panel allows
* the user to specify what string will be drawn when the
* user clicks on the drawing area.
*/
public class DrawTextPanel extends JPanel {
// As it now stands, this class can only show one string at at
// a time! The data for that string is in the DrawTextItem object
// named theString. (If it's null, nothing is shown. This
// variable should be replaced by a variable of type
// ArrayList that can store multiple items.
private DrawTextItem theString; // change to an ArrayList !
private Color currentTextColor = Color.BLACK; // Color applied to new strings.
private Canvas canvas; // the drawing area.
private JTextField input; // where the user inputs the string that will be added to the canvas
private SimpleFileChooser fileChooser; // for letting the user select files
private JMenuBar menuBar; // a menu bar with command that affect this panel
private MenuHandler menuHandler; // a listener that responds whenever the user selects a menu command
private JMenuItem undoMenuItem; // the "Remove Item" command from the edit menu
/**
* An object of type Canvas is used for the drawing area.
* The canvas simply displays all the DrawTextItems that
* are stored in the ArrayList, strings.
*/
private class Canvas extends JPanel {
Canvas() {
setPreferredSize( new Dimension(800,600) );
setBackground(Color.LIGHT_GRAY);
setFont( new Font( "Serif", Font.BOLD, 24 ));
}
protected void paintComponent(Graphics g) {
super.paintComponent(g);
((Graphics2D)g).setRenderingHint(RenderingHints.KEY_ANTIALIASING,
RenderingHints.VALUE_ANTIALIAS_ON);
if (theString != null)
theString.draw(g);
}
}
/**
* An object of type MenuHandler is registered as the ActionListener
* for all the commands in the menu bar. The MenuHandler object
* simply calls doMenuCommand() when the user selects a command
* from the menu.
*/
private class MenuHandler implements ActionListener {
public void actionPerformed(ActionEvent evt) {
doMenuCommand( evt.getActionCommand());
}
}
/**
* Creates a DrawTextPanel. The panel has a large drawing area and
* a text input box where the user can specify a string. When the
* user clicks the drawing area, the string is added to the drawing
* area at the point where the user clicked.
*/
public DrawTextPanel() {
fileChooser = new SimpleFileChooser();
undoMenuItem = new JMenuItem("Remove Item");
undoMenuItem.setEnabled(false);
menuHandler = new MenuHandler();
setLayout(new BorderLayout(3,3));
setBackground(Color.BLACK);
setBorder(BorderFactory.createLineBorder(Color.BLACK, 2));
canvas = new Canvas();
add(canvas, BorderLayout.CENTER);
JPanel bottom = new JPanel();
bottom.add(new JLabel("Text to add: "));
input = new JTextField("Hello World!", 40);
bottom.add(input);
add(bottom, BorderLayout.SOUTH);
canvas.addMouseListener( new MouseAdapter() {
public void mousePressed(MouseEvent e) {
doMousePress( e );
}
} );
}
/**
* This method is called when the user clicks the drawing area.
* A new string is added to the drawing area. The center of
* the string is at the point where the user clicked.
* @param e the mouse event that was generated when the user clicked
*/
public void doMousePress( MouseEvent e ) {
String text = input.getText().trim();
if (text.length() == 0) {
input.setText("Hello World!");
text = "Hello World!";
}
DrawTextItem s = new DrawTextItem( text, e.getX(), e.getY() );
s.setTextColor(currentTextColor); // Default is null, meaning default color of the canvas (black).
// SOME OTHER OPTIONS THAT CAN BE APPLIED TO TEXT ITEMS:
// s.setFont( new Font( "Serif", Font.ITALIC + Font.BOLD, 12 )); // Default is null, meaning font of canvas.
// s.setMagnification(3); // Default is 1, meaning no magnification.
// s.setBorder(true); // Default is false, meaning don't draw a border.
// s.setRotationAngle(25); // Default is 0, meaning no rotation.
// s.setTextTransparency(0.3); // Default is 0, meaning text is not at all transparent.
// s.setBackground(Color.BLUE); // Default is null, meaning don't draw a background area.
// s.setBackgroundTransparency(0.7); // Default is 0, meaning background is not transparent.
theString = s; // Set this string as the ONLY string to be drawn on the canvas!
undoMenuItem.setEnabled(true);
canvas.repaint();
}
/**
* Returns a menu bar containing commands that affect this panel. The menu
* bar is meant to appear in the same window that contains this panel.
*/
public JMenuBar getMenuBar() {
if (menuBar == null) {
menuBar = new JMenuBar();
String commandKey; // for making keyboard accelerators for menu commands
if (System.getProperty("mrj.version") == null)
commandKey = "control "; // command key for non-Mac OS
else
commandKey = "meta "; // command key for Mac OS
JMenu fileMenu = new JMenu("File");
menuBar.add(fileMenu);
JMenuItem saveItem = new JMenuItem("Save...");
saveItem.setAccelerator(KeyStroke.getKeyStroke(commandKey + "N"));
saveItem.addActionListener(menuHandler);
fileMenu.add(saveItem);
JMenuItem openItem = new JMenuItem("Open...");
openItem.setAccelerator(KeyStroke.getKeyStroke(commandKey + "O"));
openItem.addActionListener(menuHandler);
fileMenu.add(openItem);
fileMenu.addSeparator();
JMenuItem saveImageItem = new JMenuItem("Save Image...");
saveImageItem.addActionListener(menuHandler);
fileMenu.add(saveImageItem);
JMenu editMenu = new JMenu("Edit");
menuBar.add(editMenu);
undoMenuItem.addActionListener(menuHandler); // undoItem was created in the constructor
undoMenuItem.setAccelerator(KeyStroke.getKeyStroke(commandKey + "Z"));
editMenu.add(undoMenuItem);
editMenu.addSeparator();
JMenuItem clearItem = new JMenuItem("Clear");
clearItem.addActionListener(menuHandler);
editMenu.add(clearItem);
JMenu optionsMenu = new JMenu("Options");
menuBar.add(optionsMenu);
JMenuItem colorItem = new JMenuItem("Set Text Color...");
colorItem.setAccelerator(KeyStroke.getKeyStroke(commandKey + "T"));
colorItem.addActionListener(menuHandler);
optionsMenu.add(colorItem);
JMenuItem bgColorItem = new JMenuItem("Set Background Color...");
bgColorItem.addActionListener(menuHandler);
optionsMenu.add(bgColorItem);
}
return menuBar;
}
/**
* Carry out one of the commands from the menu bar.
* @param command the text of the menu command.
*/
private void doMenuCommand(String command) {
if (command.equals("Save...")) { // save all the string info to a file
JOptionPane.showMessageDialog(this, "Sorry, the Save command is not implemented.");
}
else if (command.equals("Open...")) { // read a previously saved file, and reconstruct the list of strings
JOptionPane.showMessageDialog(this, "Sorry, the Open command is not implemented.");
canvas.repaint(); // (you'll need this to make the new list of strings take effect)
}
else if (command.equals("Clear")) { // remove all strings
theString = null; // Remove the ONLY string from the canvas.
undoMenuItem.setEnabled(false);
canvas.repaint();
}
else if (command.equals("Remove Item")) { // remove the most recently added string
theString = null; // Remove the ONLY string from the canvas.
undoMenuItem.setEnabled(false);
canvas.repaint();
}
else if (command.equals("Set Text Color...")) {
Color c = JColorChooser.showDialog(this, "Select Text Color", currentTextColor);
if (c != null)
currentTextColor = c;
}
else if (command.equals("Set Background Color...")) {
Color c = JColorChooser.showDialog(this, "Select Background Color", canvas.getBackground());
if (c != null) {
canvas.setBackground(c);
canvas.repaint();
}
}
else if (command.equals("Save Image...")) { // save a PNG image of the drawing area
File imageFile = fileChooser.getOutputFile(this, "Select Image File Name", "textimage.png");
if (imageFile == null)
return;
try {
// Because the image is not available, I will make a new BufferedImage and
// draw the same data to the BufferedImage as is shown in the panel.
// A BufferedImage is an image that is stored in memory, not on the screen.
// There is a convenient method for writing a BufferedImage to a file.
BufferedImage image = new BufferedImage(canvas.getWidth(),canvas.getHeight(),
BufferedImage.TYPE_INT_RGB);
Graphics g = image.getGraphics();
g.setFont(canvas.getFont());
canvas.paintComponent(g); // draws the canvas onto the BufferedImage, not the screen!
boolean ok = ImageIO.write(image, "PNG", imageFile); // write to the file
if (ok == false)
throw new Exception("PNG format not supported (this shouldn't happen!).");
}
catch (Exception e) {
JOptionPane.showMessageDialog(this,
"Sorry, an error occurred while trying to save the image: " + e);
}
}
}
}
package textcollage;
import java.awt.Component;
import java.io.File;
import javax.swing.JFileChooser;
import javax.swing.JOptionPane;
/**
* This class provides a slightly simplified interface to one of Java's
* standard JFileChooser dialogs. An object of type SimpleFileChooser
* has methods that allow the user to select files for input or output.
* If the object is used several times, the same JFileChooser is used
* each time. By default, the dialog box is set to the user's home
* directory the first time it is used, and after that it remembers the
* current directory between one use and the next. However, methods
* are provided for setting the current directory. (Note: On Windows,
* the user's home directory will probably mean the user's "My Documents"
* directory".)
*/
public class SimpleFileChooser {
private JFileChooser dialog; // The dialog, which is created when needed.
/**
* Reset the default directory in the dialog box to the user's home
* directory. The next time the dialog appears, it will show the
* contents of that directory.
*/
public void setDefaultDirectory() {
if (dialog != null)
dialog.setCurrentDirectory(null);
}
/**
* Set the default directory for the dialog box. The next time the
* dialog appears, it will show the contents of that directory.
* @param directoryName A File object that specifies the directory name.
* If this name is null, then the user's home directory will be used.
*/
public void setDefaultDirectory(String directoryName) {
if (dialog == null)
dialog = new JFileChooser();
dialog.setCurrentDirectory(new File(directoryName));
}
/**
* Set the default directory for the dialog box. The next time the
* dialog appears, it will show the contents of that directory.
* @param directoryName The name of the new default directory. If the
* name is null, then the user's home directory will be used.
*/
public void setDefaultDirectory(File directory) {
if (dialog == null)
dialog = new JFileChooser();
dialog.setCurrentDirectory(directory);
}
/**
* Show a dialog box where the user can select a file for reading.
* This method simply returns getInputFile(null,null).
* @see #getInputFile(Component, String)
* @return the selected file, or null if the user did not select a file.
*/
public File getInputFile() {
return getInputFile(null,null);
}
/**
* Show a dialog box where the user can select a file for reading.
* This method simply returns getInputFile(parent,null).
* @see #getInputFile(Component, String)
* @return the selected file, or null if the user did not select a file.
*/
public File getInputFile(Component parent) {
return getInputFile(parent,null);
}
/**
* Show a dialog box where the user can select a file for reading.
* If the user cancels the dialog by clicking its "Cancel" button or
* the Close button in the title bar, then the return value of this
* method is null. Otherwise, the return value is the selected file.
* Note that the file has to exist, but it is not guaranteed that the
* user is allowed to read the file.
* @param parent If the parent is non-null, then the window that contains
* the parent component becomes the parent window of the dialog box. This
* means that the window is "blocked" until the dialog is dismissed. Also,
* the dialog box's position on the screen should be based on the position of
* the window. Generally, you should pass your application's main window or
* panel as the value of this parameter.
* @param dialogTitle a title to be displayed in the title bar of the dialog
* box. If the value of this parameter is null, then the dialog title will
* be "Select Input File".
* @return the selected file, or null if the user did not select a file.
*/
public File getInputFile(Component parent, String dialogTitle) {
if (dialog == null)
dialog = new JFileChooser();
if (dialogTitle != null)
dialog.setDialogTitle(dialogTitle);
else
dialog.setDialogTitle("Select Input File");
int option = dialog.showOpenDialog(parent);
if (option != JFileChooser.APPROVE_OPTION)
return null; // User canceled or clicked the dialog's close box.
File selectedFile = dialog.getSelectedFile();
return selectedFile;
}
/**
* Show a dialog box where the user can select a file for writing.
* This method simply calls getOutputFile(null,null,null)
* @see #getOutputFile(Component, String, String)
* @return the selcted file, or null if no file was selected.
*/
public File getOutputFile() {
return getOutputFile(null,null,null);
}
/**
* Show a dialog box where the user can select a file for writing.
* This method simply calls getOutputFile(null,null,null)
* @see #getOutputFile(Component, String, String)
* @return the selcted file, or null if no file was selected.
*/
public File getOutputFile(Component parent) {
return getOutputFile(parent,null,null);
}
/**
* Show a dialog box where the user can select a file for writing.
* This method calls getOutputFile(parent,dialogTitle,null)
* @see #getOutputFile(Component, String, String)
* @return the selcted file, or null if no file was selected.
*/
public File getOutputFile(Component parent, String dialogTitle) {
return getOutputFile(parent,dialogTitle,null);
}
/**
* Show a dialog box where the user can select a file for writing.
* If the user cancels the dialog by clicking its "Cancel" button or
* the Close button in the title bar, then the return value of this
* method is null. A non-null value indicates that the user specified
* a file name and that, if the file exists, then the user wants to
* replace that file. (If the user selects a file that already exists,
* then the user will be asked whether to replace the existing file.)
* Note that it is not quaranteed that the selected file is actually
* writable; the user might not have permission to create or modify the file.
* @param parent If the parent is non-null, then the window that contains
* the parent component becomes the parent window of the dialog box. This
* means that the window is "blocked" until the dialog is dismissed. Also,
* the dialog box's position on the screen should be based on the position of
* the window. Generally, you should pass your application's main window or
* panel as the value of this parameter.
* @param dialogTitle a title to be displayed in the title bar of the dialog
* box. If the value of this parameter is null, then the dialog title will
* be "Select Input File".
* @param defaultFile when the dialog appears, this name will be filled in
* as the name of the selected file. If the value of this parameter is null,
* then the file name box will be empty.
* @return the selected file, or null if the user did not select a file.
*/
public File getOutputFile(Component parent,
String dialogTitle, String defaultFile) {
if (dialog == null)
dialog = new JFileChooser();
if (dialogTitle != null)
dialog.setDialogTitle(dialogTitle);
else
dialog.setDialogTitle("Select Output File");
if (defaultFile == null)
dialog.setSelectedFile(null);
else
dialog.setSelectedFile(new File(defaultFile));
while (true) {
int option = dialog.showSaveDialog(parent);
if (option != JFileChooser.APPROVE_OPTION)
return null; // User canceled or clicked the dialog's close box.
File selectedFile = dialog.getSelectedFile();
if ( ! selectedFile.exists() )
return selectedFile;
else { // Ask the user whether to replace the file.
int response = JOptionPane.showConfirmDialog( parent,
"The file \"" + selectedFile.getName()
+ "\" already exists. Do you want to replace it?",
"Confirm Save",
JOptionPane.YES_NO_CANCEL_OPTION,
JOptionPane.WARNING_MESSAGE );
if (response == JOptionPane.CANCEL_OPTION)
return null; // User does not want to select a file.
if (response == JOptionPane.YES_OPTION)
return selectedFile; // User wants to replace the file
// A "No" response will cause the file dialog to be shown again.
}
}
}
}
package textcollage;
import java.awt.BasicStroke;
import java.awt.Color;
import java.awt.Font;
import java.awt.FontMetrics;
import java.awt.Graphics;
import java.awt.Graphics2D;
/**
* An object of type DrawText can draw a string in
* a graphics context, with various effects, such as
* color, magnification, and rotation The string
* is specified in the constructor and cannot be changed.
* The position and other properties can be changed.
*/
public class DrawTextItem {
private final String string; // The String that is drawn by this item.
private Font font = null;
private int x = 0;
private int y = 0;
private Color textColor = Color.BLACK;
private Color background = null;
private boolean border = false;
private double rotationAngle = 0;
private double magnification = 1;
private double textTransparency = 0;
private double backgroundTransparency = 0;
/**
* Create a DrawTextItem to draw a specified string. Initially,
* the position is set to (0,0) and all properties have their
* default values.
* @throws NullPointerException if the parameter is null
*/
public DrawTextItem(String stringToDraw) {
this(stringToDraw,0,0);
}
/**
* Create a DrawTextItem to draw a specified string. Initially,
* the position is set to (x,y) and all other properties have their
* default values. Note that x and y give the position of
* the center of the string.
* @param x the x-coordinate where the string will be drawn.
* @param y the y-coordinate where the string will be drawn.
* @throws NullPointerException if the parameter is null
*/
public DrawTextItem(String stringToDraw, int x, int y) {
if (stringToDraw == null)
throw new NullPointerException("String can't be null.");
string = stringToDraw;
this.x = x;
this.y = y;
}
/**
* Draw this item's string in the given Graphics constext,
* applying all of this item's property settings.
* @param g the graphics context in which the string will
* be drawn. Note that this is assumed to be actually of
* type Graphics2D (which should not be a problem).
*/
public void draw(Graphics g) {
Graphics2D g2 = (Graphics2D)g.create();
if (font != null)
g2.setFont(font);
FontMetrics fm = g2.getFontMetrics();
int width = fm.stringWidth(string);
int height = fm.getAscent() + fm.getDescent();
g2.translate(x,y);
if (magnification != 1) {
float pixelSize = 1/(float)magnification;
g2.setStroke( new BasicStroke(pixelSize) ); // magnification won't apply to border
g2.scale(magnification,magnification);
}
if (rotationAngle > 0)
g2.rotate( -Math.PI * (rotationAngle / 180));
Color colorToUseForText = textColor;
if (colorToUseForText == null)
colorToUseForText = g2.getColor();
if (background != null) {
if (backgroundTransparency == 0)
g2.setColor(background);
else
g2.setColor( new Color( background.getRed(), background.getGreen(), background.getBlue(),
(int)(255*(1-backgroundTransparency))) );
g2.fillRect(-width/2 - 3, -height/2 - 3, width + 6, height + 6);
}
if (textTransparency == 0)
g2.setColor(colorToUseForText);
else
g2.setColor( new Color( colorToUseForText.getRed(),
colorToUseForText.getGreen(), colorToUseForText.getBlue(),
(int)(255*(1-textTransparency)) ) );
if (border)
g2.drawRect(-width/2 -3, -height/2 - 3, width + 6, height + 6);
g2.drawString(string,-width/2, -height/2 + fm.getAscent());
}
/**
* Returns the string that is drawn by this DrawTextItem. The
* string is set in the constructor and cannot be changed.
*/
public String getString() {
return string;
}
/**
* Set the background color. If the value is non-null, then
* a rectangle of this color is drawn behind the string.
* If the value is null, no background rectangle is drawn.
* The default value is null.
*/
public void setBackground(Color background) {
this.background = background;
}
/**
* Set the level of transparency of the background color, where 0 indicates
* no transparency and 1 indicates completely transparent. If the value
* is not 1, then the transparency level of the background color is set to
* this value. If the background color is null, then the value of
* backgroundTransparency is ignored. The default value is zero.
* @param backgroundTransparency the background transparency level, in the range 0 to 1
* @throws IllegalArgumentException if the parameter is less than 0 or greater than 1
*/
public void setBackgroundTransparency(double backgroundTransparency) {
if (backgroundTransparency < 0 || backgroundTransparency > 1)
throw new IllegalArgumentException("Transparency must be in the range 0 to 1.");
this.backgroundTransparency = backgroundTransparency;
}
/**
* If border is set to true, then a rectangular border is drawn around
* the string. The default value is false.
*/
public void setBorder(boolean border) {
this.border = border;
}
/**
* Sets the font to be used for drawing the string. A value of
* null indicates that the font that is set for the graphics context
* where the string is drawn should be used. The default value is
* null.
*/
public void setFont(Font font) {
this.font = font;
}
/**
* Set a magnification level that is applied when the string is drawn.
* A magnification of 2 will double the size; a value of 0.5 will cut
* it in half. Negative values will produce backwards, upside-down text.
* Zero is not a legal value, and an attempt to set the magnification to
* zero will cause an IllegalArgumentException. The default value is
* 1, Indicating no magnification.
*/
public void setMagnification(double magnification) {
if (magnification == 0)
throw new IllegalArgumentException("Magnification cannot be 0.");
this.magnification = magnification;
}
/**
* Sets the angle of the baseline of the string with respect to the
* horizontal. The angle is specified in degrees. The default is
* 0, which gives normal, unrotated, horizontal text.
*/
public void setRotationAngle(double rotationAngle) {
this.rotationAngle = rotationAngle;
}
/**
* Sets the color to be used for drawing the text (and the border if there is one).
* The default value is null, which means that the color that is set in the
* graphics context where the string is drawn will be used for drawing the text.
*/
public void setTextColor(Color textColor) {
this.textColor = textColor;
}
/**
* Set the level of transparency of the text color, where 0 indicates
* no transparency and 1 indicates completely transparent. If the value
* is not 1, then the transparency level of the text color is set to
* this value. This property is also applied to the border, if there is one.
* The default value is zero.
* @param textTransparency the text transparency level, in the range 0 to 1
* @throws IllegalArgumentException if the parameter is less than 0 or greater than 1
*/
public void setTextTransparency(double textTransparency) {
if (textTransparency < 0 || textTransparency > 1)
throw new IllegalArgumentException("Transparency must be in the range 0 to 1.");
this.textTransparency = textTransparency;
}
/**
* Set the x-coordinate where the string is drawn. This gives the position of
* the center of the string.
*/
public void setX(int x) {
this.x = x;
}
/**
* Set the y-coordinate where the string is drawn. This gives the position of
* the center of the string.
*/
public void setY(int y) {
this.y = y;
}
/**
* returns the value of the background color property
*/
public Color getBackground() {
return background;
}
/**
* returns the value of the background transparency property
*/
public double getBackgroundTransparency() {
return backgroundTransparency;
}
/**
* returns the value of the border property
*/
public boolean getBorder() {
return border;
}
/**
* returns the value of the font property
*/
public Font getFont() {
return font;
}
/**
* returns the value of the magnification property
*/
public double getMagnification() {
return magnification;
}
/**
* returns the value of the rotation angle property
*/
public double getRotationAngle() {
return rotationAngle;
}
/**
* returns the value of the text color property
*/
public Color getTextColor() {
return textColor;
}
/**
* returns the value of the text transparency property
*/
public double getTextTransparency() {
return textTransparency;
}
/**
* returns the x-coord of the center of the string
*/
public int getX() {
return x;
}
/**
* returns the y-coord of the center of the string
*/
public int getY() {
return y;
}
}
package textcollage;
import java.awt.Dimension;
import java.awt.Toolkit;
import javax.swing.JFrame;
/**
* This main program simply shows a window that contains
* a DrawTextPanel and the menu bar for that panel.
*/
public class TextCollage {
public static void main(String[] args) {
JFrame frame = new JFrame("Text Collage");
DrawTextPanel panel = new DrawTextPanel();
frame.setContentPane( panel );
frame.setJMenuBar(panel.getMenuBar());
frame.setResizable(false);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.pack();
Dimension screenSize = Toolkit.getDefaultToolkit().getScreenSize();
frame.setLocation( (screenSize.width - frame.getWidth())/2,
(screenSize.height - frame.getHeight())/2 );
frame.setVisible(true);
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
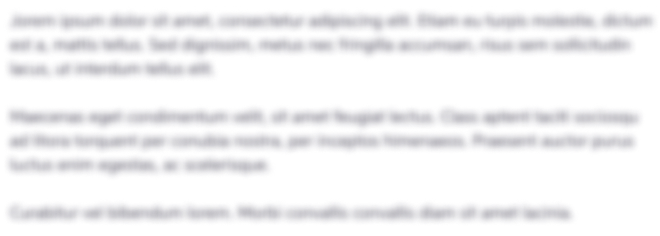
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started