Answered step by step
Verified Expert Solution
Question
1 Approved Answer
The code needs to produce nodes for the current state. and when the current state isnt the goal state, its supposed to create child nodes
The code needs to produce nodes for the current state. and when the current state isnt the goal state, its supposed to create child nodes and pick the node with the smallest manhattan distance and make that the next node in the list. Then print all of the states of the board until the goal state classÂ
Step by Step Solution
There are 3 Steps involved in it
Step: 1
The code you provided appears to be an implementation of the A search algorithm with different heuristic functions manhattan and misplaced tile count ...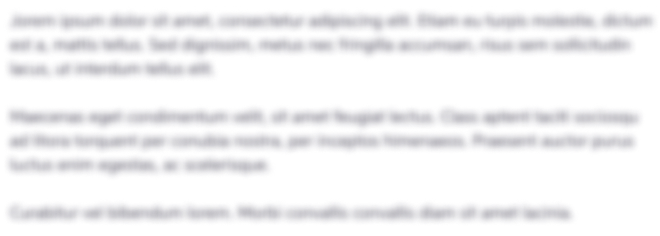
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started