Question
The code works without errors, but zeros are assigned to the predictions in the newly written .csv file. How can I get this file to
The code works without errors, but zeros are assigned to the predictions in the newly written .csv file. How can I get this file to print actual predictions? It would be great if you could modify the code. By the way, it would be great if the k-nearest neighbors algorithm is also in the code.
#include
// A struct to represent a rating given by a user to an item struct Rating { int user_id; int item_id; float rating; };
// A struct to represent a predicted rating for an item struct PredictedRating { int id; int user_id; int item_id; float rating; };
// Reads the ratings from a .csv file and returns them as a vector std::vector
std::ifstream file(filename); if (file.is_open()) { std::string line; // Skip the first line (header) std::getline(file, line); while (std::getline(file, line)) { Rating rating; std::sscanf(line.c_str(), "%d,%d,%f", &rating.user_id, &rating.item_id, &rating.rating); ratings.push_back(rating); } file.close(); }
return ratings; }
// Reads the test cases from a .csv file and returns them as a vector std::vector
std::ifstream file(filename); if (file.is_open()) { std::string line; // Skip the first line (header) std::getline(file, line); while (std::getline(file, line)) { PredictedRating test_case; std::sscanf(line.c_str(), "%d,%d,%d,%f", &test_case.id, &test_case.user_id, &test_case.item_id, &test_case.rating); test_cases.push_back(test_case); } file.close(); }
return test_cases; }
// Calculates the root mean squared error between the predicted ratings and the actual ratings
float calculate_rmse(const std::vector
float predict_rating_cosine(int user_id, int item_id, const std::unordered_map
}
// Calculates the dot product of two vectors float dot_product(const std::unordered_map
// Calculates the cosine similarity between two vectors float cosine_similarity(const std::unordered_map
// Predict the rating for a given user and item using cosine similarity float predict_rating_cosine(int user_id, int item_id, const std::unordered_map
// Calculate the cosine similarity between the given user and all other users std::vector<:pair float>> similarities; for (const auto& [other_user, ratings] : other_user_ratings) { float similarity = cosine_similarity(user_ratings, ratings); if (similarity > 0) { similarities.emplace_back(other_user, similarity); } }
// Sort the users by their similarity to the given user std::sort(similarities.begin(), similarities.end(), [](const auto& a, const auto& b) { return a.second > b.second; });
// Use the top k most similar users to predict the rating constexpr int k = 50; if (similarities.size()
int main() { // Read in the training and test data std::vector
// Initialize a map to store the ratings given by each user std::unordered_map
// Initialize a map to store the ratings received by each item std::unordered_map
std::fstream fout;
// opens an existing csv file or creates a new file. fout.open("report.csv", std::ios::out | std::ios::app);
// Predict the ratings for the test set using the mean rating of the user for (auto& predicted_rating : test_set) { predicted_rating.rating = predict_rating_cosine(predicted_rating.user_id, predicted_rating.item_id, user_ratings); fout
// Calculate the root mean squared error float rmse = calculate_rmse(test_set, training_set); std::cout
return 0; }
The code here also works without errors, but the new .csv file is assigned nan values as a prediction. So it still doesn't work as intended. You can work on both codes, but in the meantime, since the k-nearest neighbor algorithm is not used in this code, the code may need to be modified additionally to use this algorithm.
#include
#include
#include
#include
#include
#include
#include
#include
// A struct to represent a rating given by a user to an item
struct Rating {
int user_id;
int item_id;
float rating;
};
// A struct to represent a predicted rating for an item
struct PredictedRating {
int id;
int user_id;
int item_id;
float rating;
};
// Reads the ratings from a .csv file and returns them as a vector
std::vector
std::vector
std::ifstream file(filename);
if (file.is_open()) {
std::string line;
// Skip the first line (header)
std::getline(file, line);
while (std::getline(file, line)) {
Rating rating;
std::sscanf(line.c_str(), "%d,%d,%f", &rating.user_id, &rating.item_id, &rating.rating);
ratings.push_back(rating);
}
file.close();
}
return ratings;
}
// Reads the test cases from a .csv file and returns them as a vector
std::vector
std::vector
std::ifstream file(filename);
if (file.is_open()) {
std::string line;
// Skip the first line (header)
std::getline(file, line);
while (std::getline(file, line)) {
PredictedRating test_case;
std::sscanf(line.c_str(), "%d,%d,%d,%f", &test_case.id, &test_case.user_id, &test_case.item_id, &test_case.rating);
test_cases.push_back(test_case);
}
file.close();
}
return test_cases;
}
// Calculates the root mean squared error between the predicted ratings and the actual ratings
float calculate_rmse(const std::vector
float sum_squared_error = 0.0f;
for (const auto& predicted_rating : predicted_ratings) {
auto actual_rating_iter = std::find_if(actual_ratings.begin(), actual_ratings.end(), [&](const Rating& r) {
return r.user_id == predicted_rating.user_id && r.item_id == predicted_rating.item_id;
});
if (actual_rating_iter != actual_ratings.end()) {
sum_squared_error += std::pow(predicted_rating.rating - actual_rating_iter->rating, 2);
}
}
return std::sqrt(sum_squared_error / predicted_ratings.size());
}
float predict_rating_cosine(int user_id, int item_id, const std::unordered_map
// Get the ratings for the given user
const std::vector
// Create a map of the ratings for the given user
std::unordered_map
for (const Rating& rating : ratings) {
user_vec[rating.item_id] = rating.rating;
}
float sum_product = 0.0f;
float sum_user_squares = 0.0f;
float sum_other_user_squares = 0.0f;
// Find the other users who have rated the same item
for (const auto& [other_user_id, other_ratings] : user_ratings) {
if (other_user_id == user_id) {
continue;
}
for (const Rating& rating : other_ratings) {
if (rating.item_id == item_id) {
// Calculate the dot product
sum_product += rating.rating * user_vec[item_id];
// Calculate the magnitudes
sum_user_squares += std::pow(user_vec[item_id], 2);
sum_other_user_squares += std::pow(rating.rating, 2);
break;
}
}
}
// Calculate the cosine similarity
float cosine_similarity = sum_product / std::sqrt(sum_user_squares * sum_other_user_squares);
// Predict the rating
float rating = 0.0f;
for (const Rating& r : ratings) {
rating += r.rating;
}
return rating * cosine_similarity;
}
int main() {
// Read the ratings from the file
std::vector
// Group the ratings by user
std::unordered_map
for (const Rating& rating : ratings) {
user_ratings[rating.user_id].push_back(rating);
}
// Read the test cases from the file
std::vector
// Predict the ratings and write them to the file
std::ofstream file("predictions.csv");
file
for (const PredictedRating& test_case : test_cases) {
float rating = predict_rating_cosine(test_case.user_id, test_case.item_id, user_ratings);
file
}
file.close();
// Calculate the RMSE
float rmse = calculate_rmse(test_cases, ratings);
std::cout
return 0;
}
Please type similar .csv files and check if the code prints predicted ratings, thank you in advance for your answer.
Please don't spam.
0,0.000000 1,0.000000 2,0.000000 3,0.000000 4,0.000000 5,0.000000 6,0.000000 7,0.000000 8,0.000000 9,0.000000 10,0.000000 11,0.000000 12,0.000000 13,0.000000 14,0.000000 15,0.000000 16,0.000000 170.000000 ID, Predicted 0 , nan 1 , nan 2 , nan 3 , nan 4 , nan 5 , nan 6 , nan 7 , nan 8 , nan 9 , nan 10 , nan 11 , nan 12 , nan 13 , nan 14 , nan 15 , nan 16 nanStep by Step Solution
There are 3 Steps involved in it
Step: 1
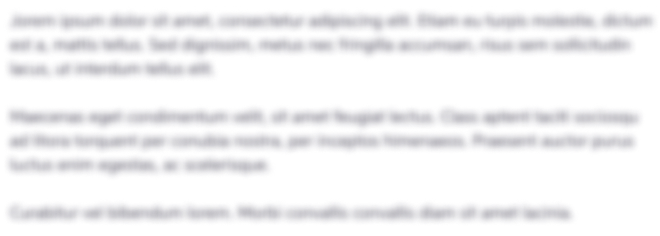
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started