Answered step by step
Verified Expert Solution
Question
00
1 Approved Answer
The cpp file is given Lab13.cpp #include #include #include #include using namespace std; class ReviewType { public: ReviewType(); // Default Constructor ReviewType(string, int); // Parameterized
The cpp file is given
Lab13.cpp #include #include #include #include using namespace std; class ReviewType { public: ReviewType(); // Default Constructor ReviewType(string, int); // Parameterized Constructor ReviewType(const ReviewType&); // Copy Constructor ~ReviewType(); // Destructor // TOTO: Implement the assignment operator overload. ReviewType& operator=(const ReviewType&); // TODO: Implement the greater than operator overload as a member function. bool operator>(const ReviewType&) const; // TODO: Implement the less than operator overload as a member function. bool operator // TODO: Implement the equality operator overload as a member function. bool operator==(const ReviewType&) const; // TODO: Just for practice implementing a NON-MEMBER function, implement // the not-equal operator overload as a NON-MEMBER function. friend bool operator!=(const ReviewType& first, const ReviewType& second) const; bool isGreater(const ReviewType&) const; bool isLess(const ReviewType&) const; bool isEqual(const ReviewType&) const; bool isNotEqual(const ReviewType&) const; string getMovieName(); void printInfo(); private: int generateRating(); string movieName; int numberOfRatings; int sumOfRatings; int *arrayPointer; }; // Default Constructor ReviewType::ReviewType() { movieName = "Default"; numberOfRatings = 0; sumOfRatings = 0; arrayPointer = NULL; } // Parameterized Constructor ReviewType::ReviewType(string movieName, int numOfRatings) { this->movieName = movieName; if (numOfRatings > 0) { this->numberOfRatings = numOfRatings; arrayPointer = new int[numberOfRatings]; for (int i = 0; i { arrayPointer[i] = generateRating(); sumOfRatings += arrayPointer[i]; } } } // Copy Constructor ReviewType::ReviewType(const ReviewType &other) { this->movieName = other.movieName; this->numberOfRatings = other.numberOfRatings; this->sumOfRatings = other.sumOfRatings; if (numberOfRatings > 0) { arrayPointer = new int[numberOfRatings]; for (int i = 0; i arrayPointer[i] = other.arrayPointer[i]; } } // The array pointer might be NULL if the movie has // 0 ratings. If the movie has more than 0 ratings, // the dynamically allocated memory will be deleted // when an instance of the ReviewType class goes // out of scope. ReviewType::~ReviewType() { if (arrayPointer != NULL) delete[] arrayPointer; } bool ReviewType::isGreater(const ReviewType &other) const { return sumOfRatings > other.sumOfRatings; } bool ReviewType::isLess(const ReviewType &other) const { return sumOfRatings } bool ReviewType::isEqual(const ReviewType &other) const { return sumOfRatings == other.sumOfRatings; } bool ReviewType::isNotEqual(const ReviewType &other) const { return sumOfRatings != other.sumOfRatings; } string ReviewType::getMovieName() { return movieName; } void ReviewType::printInfo() { cout cout cout cout for (int i = 0; i cout cout } // For simplicity, a user rating is simulated with a // random number between 1 and 10. int ReviewType::generateRating() { return (rand() % 10) + 1; } /////////////////////////////////////////////////////////////// /// Please do not change any of the code above this line. /// /////////////////////////////////////////////////////////////// /// Write your operator overload implementations here: //////////////////////////////////////////////////////////////// /// After completing your operator overload implementations, /// /// you may uncomment the statements in main that use the /// /// overloads, but other than uncommenting those lines, /// /// please don't change any of the code in main. /// //////////////////////////////////////////////////////////////// int main() { srand(time(NULL)); ReviewType movie1("Movie 1", 12); ReviewType movie2("Movie 2", 12); movie1.printInfo(); movie2.printInfo(); cout if (movie1.isGreater(movie2)) cout if (movie1.isEqual(movie2)) cout if (movie1.isLess(movie2)) cout if (movie1.isNotEqual(movie2)) cout cout // the above tests don't make use of the operator overloads. Uncomment the code below to // test your operator overload implementations. /* cout if (movie1 > movie2) cout if (movie1 == movie2) cout if (movie1 cout if (movie1 != movie2) cout cout // Here we're testing the overload of the assignment operator. Because the assignment operator // overload returns a pointer to the current object, these assignments can be chained. ReviewType movie3("Movie 3", 8); movie3.printInfo(); movie1 = movie2 = movie3; // Now movie1 and movie2 should have the same content as movie3. // (including having the same movieName as movie3). movie1.printInfo(); movie2.printInfo(); movie3.printInfo(); */ return 0; } Instructions: The Review Type class (much of which is provided for you) holds an array of ratings for a movie. The sum of each movie's ratings is held in the class variable sumOfRatings. Each instance of the Review Type class can be created with a different number of ratings, so the size of the array is dynamically allocated. Various constructors, a destructor, a printinfo function, a getMovieName function, and several Boolean returning functions are provided for you. The Boolean returning functions compare the sumOfRatings of a Review Type object passed in the parameter to the sumOfRatings of the current object. Read through the provided code and ensure you understand what each function does. If you run the provided code, you'll see that two movies are compared without the use of operator overloading. Several lines in the main function that will use operator overloading are commented out. Your task will be to implement the five operator overloads that are proto-typed in the class header. Once your implementation is complete, you may uncomment the lines in main that call your overloaded operators. These are your specific tasks: 1)Following the design in the class header, overload the> operator as a member function. True is 2) Following the design in the class header, overload the
The cpp file is given


Lab13.cpp
#include
#include
#include
#include
using namespace std;
class ReviewType
{
public:
ReviewType(); // Default Constructor
ReviewType(string, int); // Parameterized Constructor
ReviewType(const ReviewType&); // Copy Constructor
~ReviewType(); // Destructor
// TOTO: Implement the assignment operator overload.
ReviewType& operator=(const ReviewType&);
// TODO: Implement the greater than operator overload as a member function.
bool operator>(const ReviewType&) const;
// TODO: Implement the less than operator overload as a member function.
bool operator
// TODO: Implement the equality operator overload as a member function.
bool operator==(const ReviewType&) const;
// TODO: Just for practice implementing a NON-MEMBER function, implement
// the not-equal operator overload as a NON-MEMBER function.
friend bool operator!=(const ReviewType& first, const ReviewType& second) const;
bool isGreater(const ReviewType&) const;
bool isLess(const ReviewType&) const;
bool isEqual(const ReviewType&) const;
bool isNotEqual(const ReviewType&) const;
string getMovieName();
void printInfo();
private:
int generateRating();
string movieName;
int numberOfRatings;
int sumOfRatings;
int *arrayPointer;
};
// Default Constructor
ReviewType::ReviewType()
{
movieName = "Default";
numberOfRatings = 0;
sumOfRatings = 0;
arrayPointer = NULL;
}
// Parameterized Constructor
ReviewType::ReviewType(string movieName, int numOfRatings)
{
this->movieName = movieName;
if (numOfRatings > 0)
{
this->numberOfRatings = numOfRatings;
arrayPointer = new int[numberOfRatings];
for (int i = 0; i
{
arrayPointer[i] = generateRating();
sumOfRatings += arrayPointer[i];
}
}
}
// Copy Constructor
ReviewType::ReviewType(const ReviewType &other)
{
this->movieName = other.movieName;
this->numberOfRatings = other.numberOfRatings;
this->sumOfRatings = other.sumOfRatings;
if (numberOfRatings > 0)
{
arrayPointer = new int[numberOfRatings];
for (int i = 0; i
arrayPointer[i] = other.arrayPointer[i];
}
}
// The array pointer might be NULL if the movie has
// 0 ratings. If the movie has more than 0 ratings,
// the dynamically allocated memory will be deleted
// when an instance of the ReviewType class goes
// out of scope.
ReviewType::~ReviewType()
{
if (arrayPointer != NULL)
delete[] arrayPointer;
}
bool ReviewType::isGreater(const ReviewType &other) const
{
return sumOfRatings > other.sumOfRatings;
}
bool ReviewType::isLess(const ReviewType &other) const
{
return sumOfRatings
}
bool ReviewType::isEqual(const ReviewType &other) const
{
return sumOfRatings == other.sumOfRatings;
}
bool ReviewType::isNotEqual(const ReviewType &other) const
{
return sumOfRatings != other.sumOfRatings;
}
string ReviewType::getMovieName()
{
return movieName;
}
void ReviewType::printInfo()
{
cout
cout
cout
cout
for (int i = 0; i
cout
cout
}
// For simplicity, a user rating is simulated with a
// random number between 1 and 10.
int ReviewType::generateRating()
{
return (rand() % 10) + 1;
}
///////////////////////////////////////////////////////////////
/// Please do not change any of the code above this line. ///
///////////////////////////////////////////////////////////////
/// Write your operator overload implementations here:
////////////////////////////////////////////////////////////////
/// After completing your operator overload implementations, ///
/// you may uncomment the statements in main that use the ///
/// overloads, but other than uncommenting those lines, ///
/// please don't change any of the code in main. ///
////////////////////////////////////////////////////////////////
int main()
{
srand(time(NULL));
ReviewType movie1("Movie 1", 12);
ReviewType movie2("Movie 2", 12);
movie1.printInfo();
movie2.printInfo();
cout
if (movie1.isGreater(movie2))
cout
if (movie1.isEqual(movie2))
cout
if (movie1.isLess(movie2))
cout
if (movie1.isNotEqual(movie2))
cout
cout
// the above tests don't make use of the operator overloads. Uncomment the code below to
// test your operator overload implementations.
/*
cout
if (movie1 > movie2)
cout
if (movie1 == movie2)
cout
if (movie1
cout
if (movie1 != movie2)
cout
Instructions: The Review Type class (much of which is provided for you) holds an array of ratings for a movie. The sum of each movie's ratings is held in the class variable sumOfRatings. Each instance of the Review Type class can be created with a different number of ratings, so the size of the array is dynamically allocated. Various constructors, a destructor, a printinfo function, a getMovieName function, and several Boolean returning functions are provided for you. The Boolean returning functions compare the sumOfRatings of a Review Type object passed in the parameter to the sumOfRatings of the current object. Read through the provided code and ensure you understand what each function does. If you run the provided code, you'll see that two movies are compared without the use of operator overloading. Several lines in the main function that will use operator overloading are commented out. Your task will be to implement the five operator overloads that are proto-typed in the class header. Once your implementation is complete, you may uncomment the lines in main that call your overloaded operators. These are your specific tasks: 1)Following the design in the class header, overload the> operator as a member function. True is 2) Following the design in the class header, overload the cout
// Here we're testing the overload of the assignment operator. Because the assignment operator
// overload returns a pointer to the current object, these assignments can be chained.
ReviewType movie3("Movie 3", 8);
movie3.printInfo();
movie1 = movie2 = movie3;
// Now movie1 and movie2 should have the same content as movie3.
// (including having the same movieName as movie3).
movie1.printInfo();
movie2.printInfo();
movie3.printInfo();
*/
return 0;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
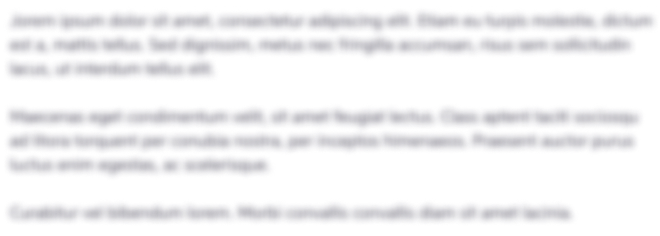
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started