Question
The Figurine module is a class that represent a collectible figurine. To accomplish the above follow these guidelines: Design and code a class named Figurine
The Figurine module is a class that represent a collectible figurine.
To accomplish the above follow these guidelines:
Design and code a class named Figurine.
Follow the usual rules for creating a module: (i.e. Compilation safeguards for the header file, namespaces and coding styles your professor asked you to follow).
Create the following constants in the Figurine header:
MAX_NAME_LEN with a value of 20.
This constant number is used to set the maximum length of the name of the Figurine.
DEFAULT_PRICE with a value of 25.25
This constant number is used to set the default price of a Figurine if an invalid value is given
Private members:
A Figurine will have the following data members:
name
This is a character array representing the nickname of the Figurine. Utilize the MAX_NAME_LEN to set the size of this array. Remember to consider the nullbyte.
pose
This is a character pointer representing the pose of the Figurine.
price
This is a double value that represents the price of the Figurine.
Public Members:
Constructors/Destructors
Figurine objects will be making use of constructors to handle their creation. There are three constructors that are required:
1.Default constructor - This constructor should set a Figurine object to a safe empty state. The notion of what the safe empty state will be left up to your design but choose reasonable values.
2.3 Argument Constructor - This constructor will take in 3 parameters:
a.A constant character pointer that represents the name of the Figurine
b.A constant character pointer representing the pose of the Figurine
c.A double value that represents the price of the Figurine
A little bit of validation needs to occur in the 3 arg constructor. If the provided constant character pointer for the name or the pose is either nullptr or an empty string then set the Figurine to a safe empty state.
Otherwise set the data members to be the values of the parameters as they are. If the provided name from the parameter is greater than MAX_NAME_LEN then simply copy over MAX_NAME_LEN characters.
Recall that the pose data member is a character pointer. This then means that dynamic memory will be required. When initializing the pose use the length of the respective parameter to determine how much memory is required. Additionally, remember that this same memory will need to be cleaned up at some point when a Figurine object goes out of scope.
Remember to close off the string with a nullbyte at the last index for all the character array data members.
If the price value provided from the parameter is less than 1 then set the price to the DEFAULT_PRICE constant.
3.Copy Constructor - This constructor will take in 1 parameter:
a.A constant Figurine reference that will be treated as a source object from which we will need a new Figurine.
In essence we are copying all the parameters of the parameter Figurine and using their values to a new Figurine with the same values. As a Figurine is composed of resources where some of them are static and others are dynamic, attention will need to be paid when copying values.
For the name and the price data members the copying can be done via shallow copying. Whereas the pose needs to apply deep copying (recall the need for independence of resources).
The steps to follow for this constructor should be the following:
Shallow copy all the static resources first
Check if the parameter Figurine's pose is in an empty state or not
If it is in an empty state then simply set the pose of the current object being created to an empty state as well.
If it isn't empty then prepare to deep copy by allocating memory for the pose based on the source Figurine's pose
After allocation copy the value from the source's pose into the current object's pose
Remember to append a null byte to the end of the pose.
Other Members
void setName(const char*);
This member function is used to set the name of the Figurine based on the parameter. Recall the need for a nullbyte at the end of the name character array.
void setPose(const char*);
This member function is used to set the pose of the Figurine based on the parameter. Recall that the pose is a character pointer thus pay attention to the need for allocation and deallocation of memory when setting the pose. Recall the need for a nullbyte at the end of the pose dynamic character array.
void setPrice(double);
This member function is used to set the price of the Figurine based on the parameter. If the price is less than 1 then set the price to the DEFAULT_PRICE.
ostream& display() const;
This member function will display the current details of the Figurine. If the Figurine is in an empty state the following will be printed:
"This Figurine has not yet been sculpted"
If the Figurine isn't empty then it will print:
"Figurine Details"
"Name: [name]"
"Pose: [pose]"
"Price: [price]"
The price should have two decimal point precision.
Refer to the sample output for details.
Lastly at the end of the function return the cout object.
Operator Overload Members
operator bool() const;
This boolean conversion operator will define the behavior of converting a Figurine into a bool value. For our context, we will use the notion of if the name is in an empty state then we will return true. Otherwise we return false
My current HEADERFILE:
#ifndef FIGURINE_H
#define FIGURINE_H
namespace ssds
{
class Figurine
{
const int MAX_NAME_LEN = 20;
const double DEFAULT_PRICE = 25.25
char Figurine_name[MAX_NAME_LEN + 1];
char* pose;
double price;
public:
Figurine();
Figurine(const char* Figurine_name, const char* pose, double price);
~Figurine();
Figurine(const& Figurine);
voit setName(const char*);
void setPose(const char*);
void setPrice(double);
ostream& display() const;
operator bool() const;
};
}
#endif
is my headerfiles based on that and what would i need to do for .cpp
Step by Step Solution
There are 3 Steps involved in it
Step: 1
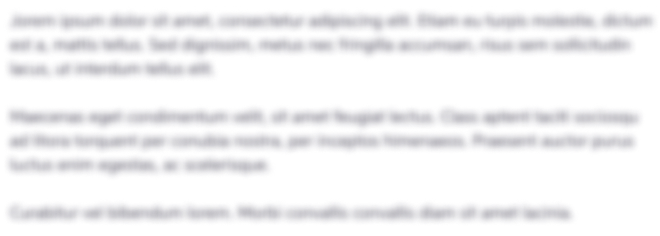
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started