Question
The files below contain the main()function and header file for a singly linked-list student database program. Using these,create a an aditional file that will work
The files below contain the main()function and header file for a singly linked-list student database program. Using these,create a an aditional file that will work with the previous two and implement the main functions for this single linked-list program. Each student entered should be sorted like the sample:
firstName, lastName, age, idNum, GPA, DOB.
James Johnson, 21, 254385987, 3.10, May 11, 1997
XXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX
Sample output
1. initialize list of students
2. add additional student to front of list
3. add additional student to rear of list
4. delete student
5. sort students alphabetically
6. sort students by id #
7. show number of students in list
8. print students
9. quit program
XXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX
// mainDriver.c (main functions) //
#include "sll_list.h"
int main()
{
int choice,list_size,id;
do
{
printf("1. initialize list of students 2. add additional student to front of list 3. add additional student to rear of list 4. delete student 5. sort students alphabetically 6. sort students by idNum 7. show number of students in list 8. print students 9. quit program ");
scanf("%d",&choice);
list_t l;
l.head=NULL;
l.tail=NULL;
switch(choice)
{
case 1://initialize list of students
scanf("%d",list_size);
l.size=list_size;
intializeList(list)t *list, FILE *inFile);
break;
case 2://add additional student to front of the list:
addToFront(l);
break;
case 3://add additional student to tail of the list:
addToRear(l);
break;
case 4://delete student
scanf("%d",id);
deleteStudent(l,id);
break;
case 5://sort students alphabetically
sortListByLN(l);
break;
case 6://sort students by id
sortListByNum(l);
break;
case 7: //number of students in the list
size(l);
break;
case 8: //printing students in the list
printStudents(l);
break;
case 9:
printf("quiting from the program");
break;
}
}while(choice!=9);
return 0;
}
XXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX
// sll_list.h (header file of singly linked-list) //
#ifndef SLL_LIST_H
#define SLL_LIST_H
#include
#include
#include
typedef struct {
char month[4];
int day, year;
} dateOfBirth;
typedef struct student {
int age;
float gpa;
int id;
dateOfBirth dob;
char lastName[20];
char firstName[15];
struct student *next;
} student_t;
typedef struct list {
student_t *head;
student_t *tail;
int size;
} list_t;
list_t *newList( );
student_t *addStudent( FILE *inFile, int whichInput );
int printMenu( );
void initializeList( list_t *list, FILE *inFile );
void addToFront( list_t *list, int whichInput, FILE *inFile );
void addToRear( list_t *list, int whichInput, FILE *inFile );
void deleteStudent( list_t *list, int idNum );
void swap( student_t *s, student_t *p);
void sortListByLN( list_t *list );
void sortListByNum( list_t *list );
int size( list_t *list );
int isEmpty( list_t *list );
void printStudents ( list_t *list );
#endif /* SLL_LIST_H */
Step by Step Solution
There are 3 Steps involved in it
Step: 1
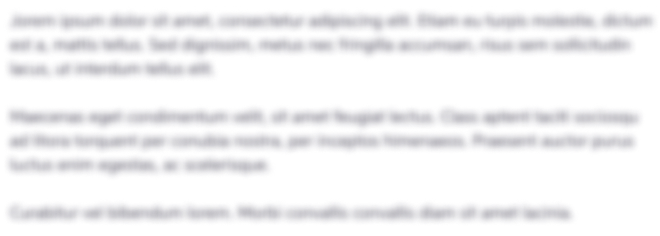
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started