Question
The following code segment will be used for the remaining questions.The code in the classes Bar and Foo is complete, and ExamI contains only a
The following code segment will be used for the remaining questions.The code in the classes Bar and Foo is complete, and ExamI contains only a partially completed main method (in other words there are no additional methods in the class ExamI, but there could be more code in the main method) .NOTE:Pay attention to the "None of the above" selections for these questions - they may require you to write additional work beyond just circling the answer.Also some of these questions may have more than one correct answer listed - you should mark ALL that apply in these cases (YY points each):
public class Bar {
private int myInt;
public Bar() { myInt = 5; }
public void method1(int x) { myInt = x; }
public int method2(int x) { return 2*x; }
}
public class Foo extends Bar {
public Foo() { super(); }
public int method2(int x) { return 4; }
}
public class ExamI {
public static void main(String[] args) {
Bar aBar = new Bar();
Foo aFoo = new Foo();
... // The rest of the code follows from this point
}
}
7. Which of the following BEST describes the relationship between the classes Bar and Foo and Object (Mark ALL that apply):
a. Foo is a subclass of Bar.
b. Bar is a subclass of Foo.
c. Object is a subclass of Bar.
d. Foo is a subclass of Object.
8. Which of the following lines will print out 5? (Mark ALL that apply)
a. System.out.println(aBar.method2() + 1);
b. System.out.println(aFoo.method2() + 1);
c. System.out.println(aBar.method2() - 5);
d. System.out.println(aFoo.method2() - 5);
e. System.out.println(aBar.myInt);
f. System.out.println(aFoo.myInt);
9. Given the code above, suppose we have the following method header in the class ExamI:
public static int sampleMethod2(Foo f)
Which of the following are correct calls to the method sampleMethod2? (Mark ALL that apply)
a. int x = sampleMethod2(aBar);
b. int x = sampleMethod2(aFoo);
c. int x = sampleMethod2();
d. None of the above calls are correct - in the space below provide a correct way to call this method from the main method of ExamI:
10. In the code above, what would be a correct way to call the method method2 in the Bar class from the main method of the class ExamI? (Mark ALL that apply):
a. Bar.method2();
b. method2();
c. aBar.method2();
d. None of the above - in the space below indicate what the correct syntax for invoking method2 would be:
11. Given only the code you can see above, what would be a correct way for the class ExamI to set myInt to 10? (Mark ALL that apply):
a. aBar.method1(10);
b. aFoo.method1(10);
c. aBar.myInt = 10;
d. ExamI cannot set myInt to 10
Step by Step Solution
There are 3 Steps involved in it
Step: 1
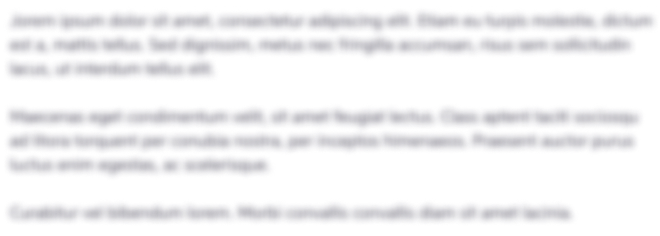
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started