Question
The following formula gives the distance between two points, (x,y) and (x,y) in the Cartesian plane: sqrt{(x_2 - x_1)^2 + (y_2 - y_1)^2} ( Given
The following formula gives the distance between two points,(x,y)and(x,y)in the Cartesian plane:
\sqrt{(x_2 - x_1)^2 + (y_2 - y_1)^2}
(
Given the center and a point on the circle, you can use this formula to find the radius of the circle.
Instructions
Write a program that prompts the user to enter the center and a point on the circle. The program should then output the circle's radius, diameter, circumference, and area. Your program must have at least the following functions:
- distance: This function takes as its parameters four numbers that represent two points in the plane and returns the distance between them.
- radius: This function takes as its parameters four numbers that represent the center and a point on the circle, calls the functiondistanceto find the radius of the circle, and returns the circle's radius.
- circumference: This function takes as its parameter a number that represents the radius of the circle and returns the circle's circumference. (Ifris the radius, the circumference is2r.)
- area: This function takes as its parameter a number that represents the radius of the circle and returns the circle's area. (Ifris the radius, the area isr.) Assume that = 3.1416.
Format your output withsetprecision(2)to ensure the proper number of decimals for testing!
Answer is given with code and sample output.
Explanation:
Code :
#include
#include
#include
using namespace std;
const float PI = 3.1416;
float distance(float x1,float x2,float y1,float y2){
float Distance;
//condition of points i.e. either of them is center or boundary points.
if((x1 != 0 || y1 !=0) && (x2 != 0 || y2 != 0))
Distance = sqrt(pow((x2-x1),2)+pow((y2-y1),2));
else
Distance = 2*sqrt(pow((x2-x1),2)+pow((y2-y1),2));
return Distance;
}
float radius(float x1,float x2,float y1,float y2){
float Radius;
//condition of points i.e. either of them is center or boundary points.
if((x1 == 0 && y1 ==0) || (x2 == 0 && y2 == 0)){
Radius = sqrt(pow((x2-x1),2)+pow((y2-y1),2));
}
else
Radius = sqrt(pow((x2-x1),2)+pow((y2-y1),2))/2;
return Radius;
}
float circumference(float R){
return 2*PI*R;
}
float area(float R){
return PI*pow(R,2);
}
int main() {
//variable declaration..
float x1,x2,y1,y2,Distance,Radius,Circuference,Area;
//Taking user input...
cout<<" Enter 1st point x-coordinate : ";
cin >> x1;
cout<<" Enter 1st point y-coordinate : ";
cin >> y1;
cout<<" Enter 2nd point x-coordinate : ";
cin >> x2;
cout<<" Enter 2nd point y-coordinate : ";
cin >> y2;
Radius = radius(x1,x2,y1,y2);
cout< cout< cout< cout< return 0; } Check for function usedistance Description Searched your code for a specific pattern: double.+distance\(.+ Description Searched your code for a specific pattern: double.+radius\(.+ Description Searched your code for a specific pattern: double.+circumference\( Description Searched your code for a specific pattern: double.+area\(
Step by Step Solution
There are 3 Steps involved in it
Step: 1
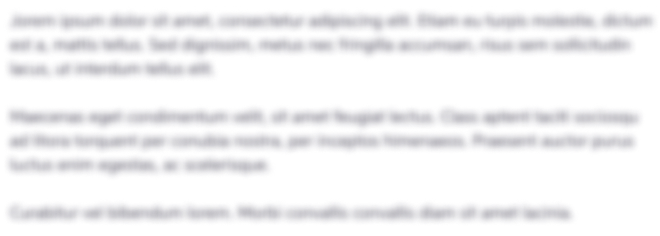
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started