Question
The following program will heavily utilize the concept of nested loops. Be sure you are comfortable with this concept before beginning. Write a C++ program
The following program will heavily utilize the concept of nested loops. Be sure you are comfortable with this concept before beginning.
Write a C++ program that plays the game of Pig, where one player is a human and the other is the computer (From here on referred to as AI => Artificial Intelligence).
The game of Pig is a simple two-player dice game in which the first player to reach N or more points wins. You can specify the game threshold; traditionally the game is played to 100 but that would make testing an debugging arduous. Using a lower threshold allows for more timely testing.
This is a turn based game. On each turn, a player rolls a single six-sided die. This dice roll determines which of the two following actions will occur.
- If the player rolls a 1, then the player gets no new points and the game switches turns to the other player.
- If the player rolls a 2 through 6, then s/he can either
- Continue their turn and ROLL AGAIN, accumulating the points for that turn on the dice roll or
- Stop their turn and HOLD. If HOLD is chosen the sum of all previous rolls on this turn is added to the players overall score and the game switches turns to the other player
-
To more deeply familiarize yourself with the game functionality you can play a graphical version here: http://www.playonlinedicegames.com/pig
-
Your version of PIG will be a console game, meaning all game output will be displayed to the console in basic text.
When it is the humans turn, the program should
Show the current score of both players and the current roll. Be sure to add some line breaks to make the display easier to comprehend.
Allow the human to input r or R to roll again or h or H to hold. Your game must handle both cases (upper and lower case).This input must also be validated, providing the player with an unlimited amount of attempts to enter the correct value. That means if the player enters a "Z" in response to this question the program should say "That is an incorrect entry. Try again: " and they should be allowed as many chances as needed to enter the correct value. (use a while loop for this)
The AI should function according to the following rule:
- Keep rolling until it has accumulated 20 or more points for that turn, then HOLD. If the AI wins or rolls a 1, then the turn ends immediately, just like the human player.
- When there is a winner the game should immediately announce which party won.
- The game should allow you to play as many games as youd like.
Tips:
A dice roll can be simulated with a random number between 1 and 6
#include
srand(time(0));
roll = rand( ) % 6 + 1;
Game Architecture:
The idea of being able to play multiple games can be controlled by a while loop. The condition can be expressed as: As long as the player wants to play again. The player should be asked if they would like to play again. This should occur IMMEDIATELY when a victor has been determined. This input must also be validated, providing the player with an unlimited amount of attempts to enter the correct value.
A turn can be implemented using a nested while loop. You will need a while loop to control the humans turn and another while loop (at the same level, not nested) to control the AI loop
The humans while loop condition can be expressed as: As long as the dice roll is not a one and the human chooses roll again
The AI while loop condition can be expressed as: As long as the dice roll is not a one and the accumulated turn points are less than twenty
Game Architecture Skeleton (obviously this is simplified and you will need to add the details, but this will give you an idea of the nesting needed):
// Control multiple games
while(player wants to play again)
//Control a single game
//Define variables needed for a single game
while(game has not been won)
//human turn
while(dice roll is not a one and the human chooses roll again)
roll dice
accumulate points
ask for Roll or Hold
while(data is not valid make them try again)
//AI turn
while(dice roll is not a one and the accumulated turn points are less than twenty)
roll dice
accumulate points
//determine game status
Did someone win? How do you know?
//play again?
ask if the player wants to play again. Validate the input
Extra Challenges (THESE ARE NOT REQUIRED):
Simulate a coin flip to see who goes first. Allow the human to call heads or tails
Allow the user to specify the point total for a win
ADVICE:
Build this application one piece at a time AND TEST THOROUGHLY AS YOU GO!!
- Start with the play again loop . .. make sure it works.
- Then do the human turn and make sure it works.
- Then do the AI turn and make sure it works
Step by Step Solution
There are 3 Steps involved in it
Step: 1
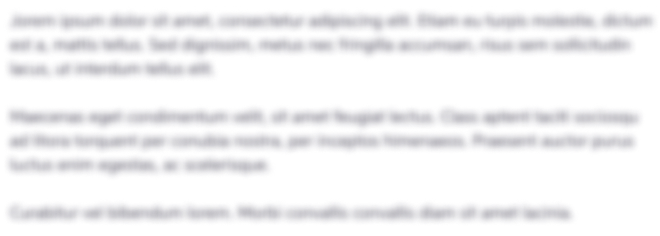
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started