Answered step by step
Verified Expert Solution
Question
1 Approved Answer
The goal of this assignment is to review the use of classes and dynamic arrays in C + + , both in the context of
The goal of this assignment is to review the use of classes and dynamic arrays in C both in the context of a maze generation application. The assignment is worth a total of points. If you have any questions or need any help, please visit us during office hours andor post questions on edstem.
If you need to post any of your actual source code on edstem for any reason, please be sure to tag the post as being private visible to instructors only, so that you don't inadvertently share code with others and violate class rules on plagiarism.
What is a Maze?
A maze is a puzzle, with starting and ending points, in which a player is tasked to find a path connecting an starting point to an ending point. Many algorithms for automatically generating random mazes have been proposed. In this assignment we will implement a randomized depthfirst search approach that uses dynamic arrays. For the context of this assignment, every maze is twodimensional and it only contains starting and ending cell. There should be exactly one path connecting both cells. The example below illustrates a random maze of dimensions n m n rows and m columns. Note that the starting point always happens at cell and the ending point at n m
Maze Generation
A data structure for representing a maze in memory may be a two dimensional array in which every cell encodes whether each of the walls is closed or open. We can assume each cell has walls: north, south, east, and west.
The algorithm for generating a maze starts with a grid where only walls are removed, north for the starting position, and south for the ending position, as illustrated in the x grid below.
A common approach for maze generation involves removing interior walls iteratively. At each iteration a wall is removed to connect two adjacent cells. This iterative process must follow these rules:
walls to be removed should be selected randomly. Use std::rand to generate random numbers and std::srand to provide a seed to the random number generator
there should be exactly one path connecting the starting and ending cells
every cell must be reachable from the starting cell
The algorithm below should be followed in your implementation. This is not the most efficient way to solve the problem of maze generation, however it is easy to understand and can be implemented with the support of simple data structures such as dynamic arrays std::vector in C
We strongly suggest you to trace this algorithm on paper using a small example eg a x grid until you fully understand how it works, before starting to code.
create empty dynamic array A
mark cell as visited
insert cell at the end of A
while A is not empty
current remove last element from A
neighborscurrents neighbors not visited yet
if neighbors is not empty
insert current at the end of A
neigh pick a random neighbor from neighbors
remove the wall between current and neigh
mark neigh as visited
insert neigh at the end of A
endif
endwhile
In order to match the autograder tests, picking a random neighbor must follow this procedure: "check the neighbors of a cell in NSEW order and append the ones that were not visited yet into an empty vector neighbors, then use the index idx below to pick a random neighbor with neighborsidx
idx std::randRANDMAX u neighbors.size;
Your Task
Your goal in this assignment is to develop a command line tool that will generate a random maze, given some options provided by the user.
Command Line Arguments
Your program must accept the following command line arguments:
the seed value for the random number generator
number of rows in the grid N
number of cols in the grid M
file name for the output
The seed argument is very important as it initializes the random number generator. If you change the seed, you will generate a different maze. In your code make sure you call this function exactly once before generating the maze:
std::srandseed;
The last argument will be used to save the generated maze into a text file. Note that you can provide any value as fname. See the example below:
$ generator example.txt
Maze file format
The file format for saving the maze is just a two dimensional array of integers, where each integer is used to represent a cell and its walls. Each integer in the matrix ranges from to The idea behind this representation is that the walls are encoded using bits, and the ingeters are just their corresponding values in decimal notation. The figure below illustrates the encoding:
When saving the grid, the output file must be a text file in which cell values are separated by a single whitespace, and organized in n rows and m columns the grid dimensions For example, the image below shows one grid with rows and columns. The text file representation of
Step by Step Solution
There are 3 Steps involved in it
Step: 1
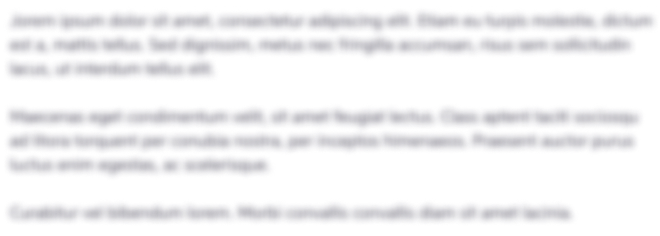
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started