Question
The goal of this lab is to create an ArraySetTests.java file which contain JUnit tests. These tests should have the following properties. Each method of
The goal of this lab is to create an ArraySetTests.java file which contain JUnit tests. These tests should have the following properties.
Each method of ArraySet is tested
One of the ArraySet.java implementations passes all tests
One implementation fails one or more tests
The tests help to identify an flaw in the implementation that fails which can then be described
Writing test cases is simply a matter of writing methods annotated with @Test which are then identified by a testing framework and run when requested. Below is are the beginnings of such a test set which appear in the provided template ArraySetTests.java file. You should add tests to this file.
import java.io.*; import org.junit.*; import static org.junit.Assert.*; import java.util.*; import org.junit.Test; // fixes some compile problems with annotations public class ArraySetTests{ public static void main(String args[]) { org.junit.runner.JUnitCore.main("ArraySetTests"); } @Test public void constructor1(){ ArraySet a = new ArraySet(); assertEquals(0, a.size()); assertEquals("[]", a.toString()); } @Test public void constructor2(){ ArraySet a = new ArraySet(5); assertEquals(0, a.size()); } @Test public void containsNothing2(){ ArraySet a = new ArraySet(); assertFalse(a.contains("hi")); assertFalse(a.contains("bye")); assertFalse(a.contains("Blackbird")); } // ADD ADDITIONAL TESTS HERE }
3.1 Assertion Functions
JUnit provides a series of assertion functions which check that the answers produced by methods produce the expected results. The most common of these are the following.
assertTrue(code): Fail the test unless code evaluates to true
assertFalse(code): Fail the test unless code evaluates to false
assertEquals(expect, actual): Fail the test unless the statement expect.equals(actual) evaluates to true. By convention, the earlier argument is the result expected by the test while the later argument is the actual result produced by the test.
What should be edited:
import java.io.*; import org.junit.*; import static org.junit.Assert.*; import java.util.*; import org.junit.Test; // fixes some compile problems with annotations
public class ArraySetTests{
public static void main(String args[]) { org.junit.runner.JUnitCore.main("ArraySetTests"); }
@Test public void constructor1(){ ArraySet a = new ArraySet(); assertEquals(0, a.size()); assertEquals("[]", a.toString()); } @Test public void constructor2(){ ArraySet a = new ArraySet(5); assertEquals(0, a.size()); } @Test public void containsNothing2(){ ArraySet a = new ArraySet(); assertFalse(a.contains("hi")); assertFalse(a.contains("bye")); assertFalse(a.contains("Blackbird")); }
// ADD ADDITIONAL TESTS HERE
}
What should not be modified impl1:
// A simple implementation of a Set of unique elements, none of which // are .equals(..) to any other in the set. Provides add(), remove(), // and contains() methods. public class ArraySet
// Virtual size private int size;
// Make a default capacity set public ArraySet(){ this(10); }
// Create a list with indicated initial capacity @SuppressWarnings("unchecked") public ArraySet(int n){ this.data = (T []) new Object[n]; this.size = 0; }
// Virtual size of the set: returns the number of unique elements in // the set public int size(){ return this.size; } // Helper to ensure that the data array has space for n elements @SuppressWarnings("unchecked") private void ensureCapacity(int n){ if(this.data.length < n){ // Must increase capacity: double in size T b[] = (T []) new Object[2 * this.data.length + 1]; for(int i=0; i // Determine if the argument x is present in the set public boolean contains(T x){ for(int i=0; i // Ensure that the given x is part of the set by adding it to the // iternal array expanding if needed. Return true if the element is // added and the size increases. If the elemnt is already present, // do not modify the set and return false. public boolean add(T x){ if(this.contains(x)){ return false; // Already present, don't change } // New element this.ensureCapacity(this.size+1); data[this.size] = x; this.size++; return true; } // Ensure that the given x is NOT part of the set by eliminating it // from the internal array. Return true if an element is removed // from the set and the size decreases. If the elemnt is not // present, return false. public boolean remove(T x){ if(!this.contains(x)){ return false; // Not present, don't change } // Find the element and nullify it for(int i=0; i // Pretty print the set public String toString(){ if(this.size()==0){ return "[]"; } StringBuffer sb = new StringBuffer(); sb.append("["); for(int i=0; i What should not be modified impl2: // A simple implementation of a Set of unique elements, none of which // are .equals(..) to any other in the set. Provides add(), remove(), // and contains() methods. public class ArraySet // Default internal capacity on creation private static int defaultSize = 10; // Internal store of objects private T [] data; // Virtual size private int size; // Make a default capacity set public ArraySet(){ this(defaultSize); } // Create a list with indicated initial capacity @SuppressWarnings("unchecked") public ArraySet(int n){ this.data = (T []) new Object[n]; this.size = 0; } // Virtual size of the set: returns the number of unique elements in // the set public int size(){ return this.size; } // Helper to ensure that the data array has space for n elements @SuppressWarnings("unchecked") private void ensureCapacity(int n){ if(this.data.length < n){ // Must increase capacity: double in size T b[] = (T []) new Object[2 * this.data.length + 1]; for(int i=0; i b[i] = data[i]; } this.data = b; } } // Determine if the argument x is present in the set public boolean contains(T x){ for(int i=0; i if(this.data[i].equals(x)){ return true; } } return false; } // Ensure that the given x is part of the set by adding it to the // iternal array expanding if needed. Return true if the element is // added and the size increases. If the elemnt is already present, // do not modify the set and return false. public boolean add(T x){ if(this.contains(x)){ return false; // Already present, don't change } // New element this.ensureCapacity(this.size+1); data[this.size] = x; this.size++; return true; } // Ensure that the given x is NOT part of the set by eliminating it // from the internal array. Return true if an element is removed // from the set and the size decreases. If the elemnt is not // present, return false. public boolean remove(T x){ if(!this.contains(x)){ return false; // Not present, don't change } // Find the element and shift over elements to overwrite it int xdx = 0; while(xdx < this.size() && !this.data[xdx].equals(x)){ xdx++; } // Located the index of x, shift elements over to overwrite it for(int i=xdx+1; i this.data[i-1] = this.data[i]; } this.size--; return true; } // Pretty print the set public String toString(){ if(this.size()==0){ return "[]"; } StringBuffer sb = new StringBuffer(); sb.append("["); for(int i=0; i sb.append(this.data[i].toString()); sb.append(", "); } sb.append(this.data[this.size-1]); sb.append("]"); return sb.toString(); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
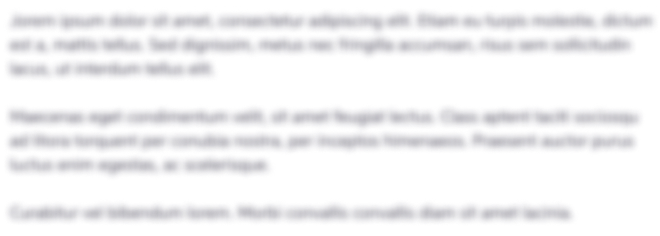
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started