Question
The instruction is below. The ImageLib.h and ImageLib.lib are code for an image provided by the instructor. C++ LANGUAGE Here is the code that I've
The instruction is below. The ImageLib.h and ImageLib.lib are code for an image provided by the instructor. C++ LANGUAGE
Here is the code that I've done so far.
#include #include "ImageLib.h"
// forward declarations void newImage(image in); void changePicColor(pixel& p, int row, int col); void mirrorPic(image img);
// main() // Preconditions: test1.gif exist and is a valid GIF file. // Postconditions: output.gif will written and it will contain the mirror image of test1.gif // with different colors. int main() { // Read the test image test1.gif image input = ReadGIF("test1.gif"); if (input.rows == 0) { cout
// create image with different colors newImage(input);
// output results WriteGIF("output.gif", input);
// wait for user input before exiting system("PAUSE"); DeallocateImage(input); return 0; }
// newImage: modifies the input image to produce different color image // Preconditions: the input image (in) has in.rows * in.cols pixel allocated // Postconditions: the input image is modified as instructed in the assignment page void newImage(image in) { for (int row = 0; row
// changePicColor: change the color of a single pixel // Preconditions: None // Postconditions: The blue color band is change as (color - (row number % 7)), and the red // color band is change as (color + (col number % 9)), green color stays the same void changePicColor(pixel& p, int row, int col) { p.blue = p.blue - (row % 7); p.red = p.red + (col % 9);
// making sure the bytes are not underflowing or overflowing if (p.blue 255) { p.blue = 255; } if (p.red 255) { p.red = 255; } }
// mirrorPic: // Preconditions: // Postconditions: the input image is mirrored. void mirrorPic(image img) { }
I've done everything until 7b, and I'm not sure how to approach the rest of the problem. We are still using the basic to C++ to answer this question so we aren't allowed to answer it with advanced material that we haven't been taught yet.
Below is the ImageLib.h file that the instructor gave us'
/* This file describes the interface to the ImageLib library
/* for use by students in CSS 501. This library provides
/* functionality to read/write GIF images and process them in
/* memory using a procedural style.
/*
/*****************************************************************/
#pragma once
#include
using namespace std;
/*
* Type definitions
*/
typedef unsigned char byte; // A single byte of data
typedef struct { // A pixel stores 3 bytes of data:
byte red; // intensity of the red component
byte green; // intensity of the green component
byte blue; // intensity of the blue component
} pixel;
// A simple image data structure:
// rows is the height of the image (the number of rows of pixels)
// cols is the width of the image (the number of columns of pixels)
// pixels is a 2D array of the image pixels
// The first dimension of pixels varies from 0 to rows-1
// The second dimension of pixels varies from 0 to cols-1
// The pixel at row i and column j is accessed by pixels[i][j].
// With the following definition:
// image myimage;
// We could access the red component of the pixel at row 10, column 20 by:
// myimage.pixels[10][20].red
typedef struct {
int rows, cols; /* pic size */
pixel **pixels; /* image data */
} image;
/*
* Function prototypes
*/
/*
* ReadGIF:
* Preconditions: filename refers to a file that stores a GIF image.
* Postconditions: returns the image contained in "filename" using the
* conventions described for the image type above.
* If the load is unsuccessful, returns an image with
* rows = 0, cols = 0, pixels = nullptr.
*/
image ReadGIF(string filename);
/*
* WriteGIF:
* Preconditions: filename is valid filename to store a GIF image.
* inputImage holds an image using the conventions described
* for the image type above.
* Postconditions: inputImage is saved as a GIF image at the location
* specified by filename.
*/
void WriteGIF(string filename, image inputImage);
/*
* DeallocateImage:
* Preconditions: inputImage contains an image using the conventions
* for the image type described above.
* Postconditions: the memory allocated to the pixels of inputImage has
* been deallocated. Also, the image values are set to:
* rows = 0, cols = 0, pixels = nullptr.
*/
void DeallocateImage(image &inputImage);
/*
* CopyImage:
* Preconditions: inputImage contains an image using the conventions
* for the image type described above.
* Postconditions: if sufficient memory is available, a copy of inputImage
* is returned using newly allocated memory.
* Otherwise, the returned image has:
* rows = 0, cols =0 , pixels = nullptr.
*/
image CopyImage(image inputImage);
/*
* CreateImage:
* Preconditions: rows and cols describe the desired size of the new image.
* Postconditions: if sufficient memory is available, a new image
* is returned using newly allocated memory.
* Each pixel has red = 0, green =0, blue = 0.
* Otherwise, the returned image has:
* rows = 0, cols =0, pixels = nullptr.
*/
image CreateImage(int rows, int cols);
2. Create a new C++ project in Visual Studio called "Program1". The project should and Empty C++ Project. If your project has stdafx.h or targetver.h, then you did this incorrectly. Your code should compile without these files and you should not turn them in. Remember where you save the project. 3. Download the following files and place them in .../Program 1/Program 1/: ImageLib.h , ImageLib.lib, ImageLib.pdb, test1.gif. You should not change the contents of these files in order to complete the assignment. 4. Examine ImageLib.h in order to understand the interface for reading and writing GIF images. You should not be able to access the implementation details of ImageLib.lib (this is abstraction, I am hiding the details). Note that images are not first-class objects. For example, there is no copy constructor or assignment operator that performs a deep copy. Functions are provided in the library for these purposes. 5. Add Imagelib.h and ImageLib.lib to your project. (Project - Add Existing Item.) 6. Create a new source file in Program1 called main.cpp (Project - Add New Item). We are now ready to start coding. 7. Edit main.cpp to accomplish the following in order: a. Read the image test1.gif. b. For every pixel of this image: i. Subtract row mod 7 from the blue component of the pixel, where row is the row of the current pixel. The mod operator uses the % symbol. ii. Add col mod 9 to the red component of the pixel, col is the column of the current pixel. iii. The green component should be unchanged. iv. As you are doing this, make sure to check for overflow and underflow, since bytes are unable to store values below 0 or above 255. If you find overflow, set the byte to 255. If you find underflow, set the byte to 0. c. Create a new image that is the mirror-image of the image from the previous step. (The left side and the right side are reversed.) d. Save the new image to a file called output.gif. e. Read output.gif back into a new image variable. f. Compare each pixel in the newly read image to the one that you saved in step d (not the original). Are there any differences? g. Count and output to the console the number of pixels that have a different values in red, green, or blue. (If a pixel has a difference in any of the three values, then that pixels counts once toward the count. A pixel cannot count more than once.)Step by Step Solution
There are 3 Steps involved in it
Step: 1
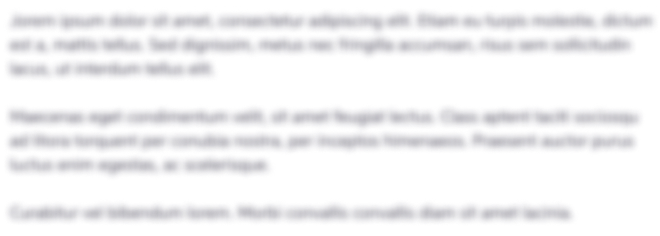
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started