The main program reads information of five courses and five students as input and stores the information into an ArrayList of Courses and an ArrayList
The main program reads information of five courses and five students as input and stores the information into an ArrayList of Courses and an ArrayList of Students. The program then sorts both ArrayLists and outputs the sorted information.
Course has private fields:
- String department
- Integer number
Course has a constructor and member methods:
- Course(dept, num) - set department to parameter dept and number to parameter num
- compareTo(otherCourse) - to enable sorting of Course objects, return -1, 0, or 1 according to the comparisons of the private members between Course and otherCourse. Precedence of comparisons: department (lowest first), then number (lowest first)
- toString() - return a string representation of a course in the format "department number"
Student has private fields:
- String firstName;
- String lastName;
- double GPA;
Student has a constructor and member methods:
- Student(first, last, gradeAverage) - set firstName to parameter first, lastName to parameter last, and GPA to parameter gradeAverage
- compareTo(otherStudent) - to enable sorting of Student objects, return -1, 0, or 1 according to the comparisons of the private members between Student and otherStudent. Precedence of comparisons: GPA (highest first), then lastName (lowest first), then firstName (lowest first)
- toString() - return a string representation of a student in the format "GPA lastName, firstName"
Ex: If the input is:
Chemistry 250 Chemistry 300 Chemistry 200 Biology 200 Biology 100 Ravi Coltrane 3.75 Oliver Lake 2.9 Lol Coxhill 3.5 John Zorn 2.4 Joe Lavano 2.4
the output is:
Biology 100 Biology 200 Chemistry 200 Chemistry 250 Chemistry 300 3.75 Coltrane, Ravi 3.50 Coxhill, Lol 2.90 Lake, Oliver 2.40 Lavano, Joe 2.40 Zorn, John
LabProgram.java
import java.util.Scanner;
import java.util.ArrayList;
import java.util.Collections;
public class LabProgram {
public static void main(String[] args) {
int j;
Scanner scnr = new Scanner(System.in);
ArrayList courses = new ArrayList();
ArrayList students = new ArrayList();
// Input 5 courses
for (j = 0; j < 5; ++j) {
courses.add(new Course(scnr.next(), scnr.nextInt()));
}
// Input 5 students
for (j = 0; j < 5; ++j) {
students.add(new Student(scnr.next(), scnr.next(), scnr.nextDouble()));
}
// Sort courses and students
Collections.sort(courses);
Collections.sort(students);
// Output courses and students
for (j = 0; j < courses.size(); ++j) {
System.out.println(courses.get(j));
}
System.out.println();
for (j = 0; j < students.size(); ++j) {
System.out.println(students.get(j));
}
}
}
Course.java
import java.util.Scanner;
import java.util.ArrayList;
import java.util.Collections;
class Course implements Comparable {
private String department;
private Integer number;
public Course(String aDepartment, Integer aNumber) {
department = aDepartment;
number = aNumber;
}
@Override
public int compareTo(Course c) {
if (department.compareTo(c.department) == 0) {
return number.compareTo(c.number);
}
return department.compareTo(c.department);
}
public String toString() {
return department + " " + number;
}
}
class Student implements Comparable{
private String firstName;
private String lastName;
private double gpa;
public Student(String aFirstName, String aLastName, double aGpa) {
firstName = aFirstName;
lastName = aLastName;
gpa = aGpa;
}
@Override
public int compareTo(Student s) {
if(gpa == s.gpa) {
if(lastName.compareTo(s.lastName)==0) {
return firstName.compareTo(s.firstName);
}
else {
return lastName.compareTo(s.lastName);
}
}
return new Double(s.gpa).compareTo(gpa);
}
public String toString() {
return gpa+" "+lastName+", "+firstName;
}
}
Student.java
/* Type your code here for class Student. */
Step by Step Solution
There are 3 Steps involved in it
Step: 1
java import javautilScanner import javautilArrayList import javautilCollections public class LabProg...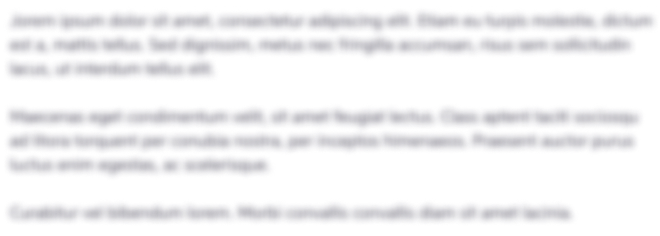
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started