Question
___________________________________ the Park Class: public class Park { public int minimumAge; public double minimumHeight; public int minimumTickets; /** * This method should have semantics very
___________________________________
the Park Class:
public class Park { public int minimumAge; public double minimumHeight; public int minimumTickets; /** * This method should have semantics very similar to the attemptToRide method in the homework * However, this time, since the Ride fields are public, we have to check them against * the Park object's minimums. * Determine the requirements as follows: for of the three requirements, if the Ride object has a requirement * that is at least the Park object's minimum, use that. Otherwise, use the Park's minimum. * (Don't change the Ride object's fields) * If the Person is old enough, tall enough, and has enough tickets, * subtract that number of tickets from the Person and return true * If the Ride is null, or the Person is null, return false without changing anything. * If any of this Park's, or the Person's or Ride's fields are negative, this should return false * without changing anything. * @param guest who is attempting to ride * @param ride to be ridden * @return true if they were able to ride, false otherwise. * return false if ride is null or invalid values are detected. */ public boolean ride(Person guest, Ride ride) { return true; } }
__________________________________
the Person Class:
/* * A class that represents a person in an amusement park. * It stores its age, height, and number of tickets. */
public class Person { //unlike homework, the fields are public, which means anyone can modify them public int age; public double height; public int tickets; //if you don't write a constructor, Java creates a constructor that does nothing. //this leaves the fields with default values }
_________________________________________
the Ride Class:
/* * A class that represents a ride in an amusement park * It stores the requirements for riding */
public class Ride { //unlike homework, the fields are public, which means anyone can modify them
public int ageReq; public int ticketReq; public double heightReq; //if you don't write a constructor, Java creates a constructor that does nothing. //this leaves the fields with default values }
__________________________________
the Test Class:
import junit.framework.TestCase;
public class TestClass extends TestCase{ Person defaultsPerson; Person fivesPerson; Person negAgePerson; Person negHeightPerson; Person negTicketsPerson; Person lowAgePerson; Person lowHeightPerson; Person lowTicketsPerson;
Ride defaultsRide; Ride fivesRide; Ride negAgeRide; Ride negHeightRide; Ride negTicketsRide;
Park defaultsPark; Park threesPark; Park negAgePark; Park negHeightPark; Park negTicketsPark; Park highAgePark; Park highHeightPark; Park highTicketsPark; public void setUp() { //create a bunch of objects to use for testing later defaultsPerson = new Person(); fivesPerson = new Person(); fivesPerson.age = 5; fivesPerson.height = 5.0; fivesPerson.tickets = 5; negAgePerson = new Person(); negAgePerson.age = -1; negAgePerson.height = 5.0; negAgePerson.tickets = 5; negHeightPerson = new Person(); negHeightPerson.age = 5; negHeightPerson.height = -1.0; negHeightPerson.tickets = 5; negTicketsPerson = new Person(); negTicketsPerson.age = 5; negTicketsPerson.height = 5.0; negTicketsPerson.tickets = -1; lowAgePerson = new Person(); lowAgePerson.age = 2; lowAgePerson.height = 5.0; lowAgePerson.tickets = 5; lowHeightPerson = new Person(); lowHeightPerson.age = 5; lowHeightPerson.height = 2.0; lowHeightPerson.tickets = 5; lowTicketsPerson = new Person(); lowTicketsPerson.age = 5; lowTicketsPerson.height = 5.0; lowTicketsPerson.tickets = 2;
defaultsRide = new Ride(); fivesRide = new Ride(); fivesRide.ageReq = 5; fivesRide.heightReq = 5.0; fivesRide.ticketReq = 5; negAgeRide = new Ride(); negAgeRide.ageReq = -1; negAgeRide.heightReq = 5.0; negAgeRide.ticketReq = 5; negHeightRide = new Ride(); negHeightRide.ageReq = 5; negHeightRide.heightReq = -1.0; negHeightRide.ticketReq = 5; negTicketsRide = new Ride(); negTicketsRide.ageReq = 5; negTicketsRide.heightReq = 5.0; negTicketsRide.ticketReq = -1;
defaultsPark = new Park(); threesPark = new Park(); threesPark.minimumAge = 3; threesPark.minimumHeight = 3.0; threesPark.minimumTickets = 3; negAgePark = new Park(); negAgePark.minimumAge = -1; negAgePark.minimumHeight = 3.0; negAgePark.minimumTickets = 3; negHeightPark = new Park(); negHeightPark.minimumAge = 3; negHeightPark.minimumHeight = -1.0; negHeightPark.minimumTickets = 3; negTicketsPark = new Park(); negTicketsPark.minimumAge = 3; negTicketsPark.minimumHeight = 3.0; negTicketsPark.minimumTickets = -1; highAgePark = new Park(); highAgePark.minimumAge = 10; highAgePark.minimumHeight = 3.0; highAgePark.minimumTickets = 3; highHeightPark = new Park(); highHeightPark.minimumAge = 3; highHeightPark.minimumHeight = 10.0; highHeightPark.minimumTickets = 3; highTicketsPark = new Park(); highTicketsPark.minimumAge = 3; highTicketsPark.minimumHeight = 3.0; highTicketsPark.minimumTickets = 10; } //test default values (for primitive numeric variables these are generally 0 values) public void test00() { assertTrue(defaultsPark.ride(defaultsPerson, defaultsRide)); assertEquals(0, defaultsPerson.tickets); assertTrue(defaultsPark.ride(defaultsPerson, defaultsRide)); } //test nulls //why didn't we test null.ride(...)? public void test01() { assertFalse(defaultsPark.ride(null, null)); assertFalse(defaultsPark.ride(fivesPerson, null)); assertFalse(defaultsPark.ride(null, fivesRide)); assertEquals(5, fivesPerson.tickets); assertEquals(5, fivesRide.ticketReq); } //test negative values public void test02() { assertFalse(negAgePark.ride(fivesPerson, fivesRide)); assertFalse(negHeightPark.ride(fivesPerson, fivesRide)); assertFalse(negTicketsPark.ride(fivesPerson, fivesRide)); assertFalse(threesPark.ride(negAgePerson, fivesRide)); assertFalse(threesPark.ride(negHeightPerson, fivesRide)); assertFalse(threesPark.ride(negTicketsPerson, fivesRide)); assertFalse(threesPark.ride(fivesPerson, negAgeRide)); assertFalse(threesPark.ride(fivesPerson, negHeightRide)); assertFalse(threesPark.ride(fivesPerson, negTicketsRide)); } //test ride-set requirements public void test03() { assertTrue(threesPark.ride(fivesPerson, fivesRide)); assertEquals(0, fivesPerson.tickets); assertFalse(threesPark.ride(fivesPerson, fivesRide)); assertFalse(threesPark.ride(defaultsPerson, fivesRide)); assertFalse(threesPark.ride(lowAgePerson, fivesRide)); assertFalse(threesPark.ride(lowHeightPerson, fivesRide)); assertFalse(threesPark.ride(lowTicketsPerson, fivesRide)); assertEquals(2, lowTicketsPerson.tickets); assertEquals(3, threesPark.minimumAge); assertEquals(3.0, threesPark.minimumHeight); assertEquals(3, threesPark.minimumTickets); } //test park-set requirements public void test04() { assertTrue(threesPark.ride(fivesPerson, defaultsRide)); assertEquals(2, fivesPerson.tickets); assertFalse(threesPark.ride(fivesPerson, defaultsRide)); fivesPerson.tickets = 5; assertFalse(highAgePark.ride(fivesPerson, fivesRide)); assertFalse(highHeightPark.ride(fivesPerson, fivesRide)); assertFalse(highTicketsPark.ride(fivesPerson, fivesRide)); assertEquals(5, fivesPerson.tickets); assertEquals(5, fivesRide.ageReq); assertEquals(5.0, fivesRide.heightReq); assertEquals(5, fivesRide.ticketReq); } //test other combinations public void test05() { Person testPerson = new Person(); testPerson.age = 12; testPerson.height = 4.0; testPerson.tickets = 8; assertFalse(highAgePark.ride(testPerson, fivesRide)); testPerson = new Person(); testPerson.age = 12; testPerson.height = 8.0; testPerson.tickets = 4; assertFalse(highAgePark.ride(testPerson, fivesRide)); testPerson = new Person(); testPerson.age = 8; testPerson.height = 12.0; testPerson.tickets = 4; assertFalse(highHeightPark.ride(testPerson, fivesRide)); testPerson = new Person(); testPerson.age = 4; testPerson.height = 12.0; testPerson.tickets = 8; assertFalse(highHeightPark.ride(testPerson, fivesRide)); testPerson = new Person(); testPerson.age = 4; testPerson.height = 8.0; testPerson.tickets = 12; assertFalse(highTicketsPark.ride(testPerson, fivesRide)); testPerson = new Person(); testPerson.age = 8; testPerson.height = 4.0; testPerson.tickets = 12; assertFalse(highTicketsPark.ride(testPerson, fivesRide)); assertEquals(5, fivesRide.ageReq); assertEquals(5.0, fivesRide.heightReq); assertEquals(5, fivesRide.ticketReq); } }
_________________________________________
Please complete the program above by using Java Language and post a picture of the passing test.
Introduction This week's lab will explore a different design for the homework: one that doesn't follow principles of encapsulation. Here, we will make the object variables of the Person and Ride classes public, which means the object has no control over what values those variables can take. As a result, when we write a method that uses those variables, we have to test the variables to see if their values are valid. What you need to do Import the project into Eclipse. Look at the Person and Ride classes. They are very simple, and have no methods, only public fields. Now look at the Park class. Here, your task is to write a single, kind of complicated method. This method will take a Person and Ride, and check whether the Person can ride the Ride. This method will have to test the variable fields to ensure they make sense, which is a lot more work for you. The method should: 1. Receive a Person and a Ride as parameters. 2. If either is null, return false. 3. If the Person has a negative age, height or number of tickets, return false. 4. If the Ride has a negative value for any of its requirements, return false 5. If this Park object has a negative value for any of its minimums, return false. 6. Determine the requirements for this person to ride. For each of age, height, and tickets, use the Ride object's requirement, unless it is less than the Park's minimum, in that case use the Park's minimum for that requirement instead. 7. If the Person does not meet any of the three requirements computed in step 6, return false. A Person does not meet the requirement if their age, height, or number of tickets is less than the computed requirement. 8. Subtract the computed ticket requirement from the Person's number of tickets. (THIS IS THE ONLY STEP THAT SHOULD CHANGE ANY OBJECT'S FIELDS) 9. Return true. (Ctrl) - Introduction This week's lab will explore a different design for the homework: one that doesn't follow principles of encapsulation. Here, we will make the object variables of the Person and Ride classes public, which means the object has no control over what values those variables can take. As a result, when we write a method that uses those variables, we have to test the variables to see if their values are valid. What you need to do Import the project into Eclipse. Look at the Person and Ride classes. They are very simple, and have no methods, only public fields. Now look at the Park class. Here, your task is to write a single, kind of complicated method. This method will take a Person and Ride, and check whether the Person can ride the Ride. This method will have to test the variable fields to ensure they make sense, which is a lot more work for you. The method should: 1. Receive a Person and a Ride as parameters. 2. If either is null, return false. 3. If the Person has a negative age, height or number of tickets, return false. 4. If the Ride has a negative value for any of its requirements, return false 5. If this Park object has a negative value for any of its minimums, return false. 6. Determine the requirements for this person to ride. For each of age, height, and tickets, use the Ride object's requirement, unless it is less than the Park's minimum, in that case use the Park's minimum for that requirement instead. 7. If the Person does not meet any of the three requirements computed in step 6, return false. A Person does not meet the requirement if their age, height, or number of tickets is less than the computed requirement. 8. Subtract the computed ticket requirement from the Person's number of tickets. (THIS IS THE ONLY STEP THAT SHOULD CHANGE ANY OBJECT'S FIELDS) 9. Return true. (Ctrl)Step by Step Solution
There are 3 Steps involved in it
Step: 1
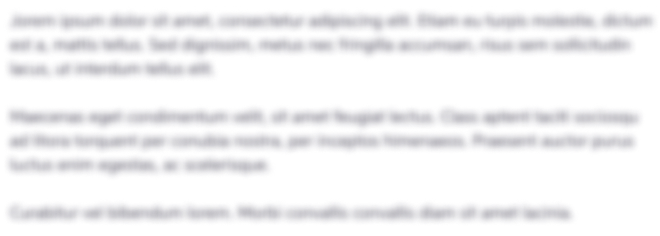
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started