Question
The Problem The unfortunate effects of the University-wide budget cuts are seemingly limitless, with practically every department hurting in some way. Due to lack of
The Problem
The unfortunate effects of the University-wide budget cuts are seemingly limitless, with practically every department hurting in some way. Due to lack of funding, the online class registration portal is no longer available; weve turned back the clock about twenty years! In order to register for classes, you must be physically present at the Registrars Office in Millican Hall. (If it makes you feel any better, all UCF students are in the same boat!) Upon arriving to the Registrars office (which runs from 12:00 PM to 5:00 PM, 7 days a week). Practice using stacks and queues.
Your Assignment is to write a simulation that models Registration over n number of days, where the simulation runs over each minute of every day, from 12 PM to 5 PM.
Implementation
You must use the following structs, as shown. You may, at your own discretion, add struct members as you see fit. The next member of the student struct assumes the use of a linked-list queue.
typdef struct ucfClass {
char ID[10]; // class ID, ex: "COP-3502" char days[6]; // a combination of "MTWRF" (days) char time[20]; // class time, ex: "10:30 AM - 12:00 PM"
} class;
typedef struct ucfStudent {
char lastName[21]; char firstName[21]; int ID; int laptopID; int errorCode; int numClasses; class *classes; int enterTime; int timeLeft; struct ucfStudent *next; // pointer to next student in queue } student; | |
Outside Line:
For a given day, you will first read in, from a file, all the students that will ultimately arrive during that day. Students will be in chronological order in the file i.e. the first student in the file will be first to enter the Registrars office. You must malloc space for each student, save their appropriate (read-in) information into their student node, and then you will enqueue them into, what we refer to as, the outside line. What is the outside line? The outside line (which is clearly a queue) will contain all of the students who are expected to come during that particular day. As time moves forward, you will remove (dequeue) students from the outside line if the currentTime (your daily looping variable over each minute) equals the arrival time of the student who is at the front of the outside line. Once a student arrives (is dequeued from the outside line), they are immediately enqueued into the laptop waiting line. Note: it is possible that multiple students will arrive at the Registrars office at the same time. Meaning, multiple students can possibly have the same arrival time within the outside line. Students that arrive at the same time are placed in the laptop line in the order that they arrive (based on the chronological order of the input file). The choice is yours as to whether this outside line will be an array based queue or a linked list based queue. Either way, you will be using pointers since each student is saved in a struct student, which is referenced by way of a pointer.
Laptop Waiting Line:
As mentioned, as soon as a student enters the Registrars office, they must stand in line to check out a
laptop. You will implement this line as a queue. The choice is yours as to whether this will be an array based queue or a linked-list based queue. Either way, you will be using pointers since each student is saved in a struct student, which is referenced by way of a pointer.
Stacks of Laptops:
The laptops are to be stored in a stack and are identified by their unique serial number. Therefore, you will implement this as a stack. The input file will have a list of laptops at the beginning which will be placed into the stack. This is constant over the whole simulation i.e.: no new laptops are added after the beginning. However, the order in which they are stored in the stack will surely change, just as the order of trays in a cafeteria change. Some students will use the laptops longer than others (because of mistakes during registration), resulting in the laptops being placed back on the stack at variable times. Note: the laptop stack will NOT reset to its original state after each simulated day. Rather, the order of the laptops, at the end of any given day, will be the order for the start of the next day. The choice is yours as to whether this will be an array based stack or a linked-list based stack.
Laptop Return Line: The laptop return line will also be implemented as a queue. Again, the choice is yours as to whether this will be an array based queue or a linked-list based queue.
Completed Registrations: Successful registrations will be saved into an ordered linked-list, which will be ordered alphabetically (by last name and then by first name). Although students may have the same last name or the same first name, it is guaranteed that all students have a unique first and last name combination. All registrations in this linked list must be erased on a daily basis at the time of printing the daily summary. Well just assume they get saved into a master UCF database (beyond the scope of this assignment).
And now the nuts and bolts:
- When a student arrives at the Registrars office, that student immediately enters the laptop checkout line (during the same minute).
- Once at the front of the laptop check-out line, you can only leave this line once a laptop is available. So even if you are at the front of the line, if no laptops are available, you will not leave the line. Lets say a laptop becomes available at 12:20 PM, then, also at 12:20 PM, you will leave the laptop line and work with the LDM to check out a laptop, which then takes one minute, as described below, resulting in your getting the laptop at 12:21 PM.
- The brief interaction with the LDM takes one minute; meaning, it takes one minute to get a laptop. So if you arrive at 4:30 PM and find an empty laptop line, and even if laptops are available, you will not actually get your laptop until 4:31 PM.
- As such, a maximum of one laptop is dispensed every minute. So even if three students arrive at 1:12 PM, they will all enter the laptop line at 1:12 PM, and if all five laptops are available, the first student will get a laptop at 1:13 PM, the second at 1:14 PM, and the third at 1:15 PM.
- If a student gets a laptop at 4:31 PM, they will finish registering and will enter the laptop return line at 4:36 PM.
- The brief interaction with the LRM also takes one minute; meaning, it takes one minute to confirm successful registration and return the laptop. So if you are at the front of the laptop return line at 4:40 PM (with no mistakes on the registration), you will return the laptop and leave the Registrars office at 4:41 PM.
- On the flipside, if you are at the front of the laptop return line at 4:40 PM and you have mistakes, you still have this brief interaction with the LRM who informs you of said mistakes. You then start your second (and final) five minute registration session at 4:41 PM.
- At a given minute, you could end up with a student taking a laptop from the stack and a student, who is finished, placing a laptop on the stack. So we need a rule: always process the student returning the laptop before processing the student taking a laptop.
- Also, at a given minute, two students could be trying to enter the laptop return line at the same time. This could occur when a student, who had a mistake and had to spend another five minutes, finishes their second five minutes at the same time that another student finishes their original five minutes. So we need a rule: if more than one student is trying to enter the laptop return line at a given minute, always enqueue, first, the student who has the earliest enterTime (the student who has been at the office the longest).
- Students can arrive at the Registrars office up until 4:59 PM. You are guaranteed that no student arrive after this time.
- Although the Registrars office officially closes at 5:00 PM, the office does stay open to finish the registrations of all students who arrived by 4:59 PM. So even if 100 students arrive right at 4:59 PM, this is no problem. The Registrars office will stay open until all students have been processed. However, it is guaranteed that this will never take beyond 11:59 PM.
Input File Specifications
You will read in input from a file, "KnightsRegistrar.in". Have this AUTOMATED. Do not ask the user to enter KnightsRegistrar.in. You should read in this automatically (this will expedite the grading process). The file will contain an integer j followed by j random, unique laptop serial numbers. Next will be an integer n, followed by n number of days. For each day there will be an integer k, followed by k number of students, where each students information will be on a single line as follows:
ENTERTIME LASTNAME FIRSTNAME ID NUMCLASSES ERRORCODE
ENTERTIME is the time in minutes from 12:00 PM. LASTNAME is the last name of the student arriving. FIRSTNAME is the first name of the student arriving. ID is the student's ID number. NUMCLASSES is the number of classes the student will register. ERRORCODE is the number used to determine if the student has will make an error while registering. 1 signifies an error; 0, otherwise.
Each student line is followed by NUMCLASSES lines with class data on each line:
CLASSID DAYS TIME
CLASSID is a string representing the ID of a class (ex. "COP-3502"). DAYS is a string of letters representing the days of the week a class occurs on (ex. "MTWRF"). TIME is a string representing the time a class occurs at (ex. "10:30 AM - 12:00 PM"). Note: this time (10:30 AM - 12:00 PM) will need be stored as one string. HINT: visit Clarification section of discussion board for advice on how to read in this TIME string of characters. I will post the easy method to do this.
Output File Specifications
Your program must output to a file, called "KnightsRegistrar.out". You must follow the program specifications exactly. You will lose points for formatting errors and spelling.
For each day, print out a header with the following format:
********** Day X: **********
Where X is the nth day of the simulation. Follow this header with one blank line.
The following lines will give information about students entering, checking-out up a laptop, finishing registration and entering the laptop return line, making mistakes and having to redo the registration, and finally, returning the laptop upon successful completion of registration. These lines should be printed in the order in which the actions occur. The formatting for these lines is as follows:
TIME: STUDENT_NAME has arrived at the Registrar and entered the laptop line.
TIME: STUDENT_NAME has checked-out laptop # SERIAL.
TIME: STUDENT_NAME finished registering and entered the laptop return line.
TIME: STUDENT_NAME made an error and must redo the registration.
TIME: STUDENT_NAME successfully registered and returned laptop # SERIAL.
Where TIME is the time an action occurred, STUDENT_NAME is the name of the student (first name, followed by a space, and then followed by the last name), and SERIAL is the serial number of the laptop the student is using.
The time should be printed out in the following format:
(H)H:MM PM
The first one (or possibly two) digits represent the hour. The hour must not be printed as 0 if it is between noon and 1:00, so youll need to check for this. This is followed by a colon and then the next two digits represent the minute. If the minute value is less than 10, youll need to add a leading 0, so that it prints as 3:05, not 3:5. (It probably makes sense to have a function that takes in as input the number of minutes after 12:00 PM and, in turn, prints out the corresponding time in this format.)
Follow each day's output with one blank line. Then you will print the day's statistics as follows:
*** Day X: UCF Daily Registration Report ***:
The Registrar received Y registrations as follows:
Where X is the day number (of the simulation), and Y is total number of students that came that day. This is followed by an alphabetical (last name, and then first) printout of all registrations that occurred during the given day:
LASTNAME, FIRSTNAME, ID # STUDENTID
Time Registered: (H)H:MM PM Classes:
| CLASSID | DAYS | TIME | ...
LASTNAME, FIRSTNAME, ID # STUDENTID
Time Registered: (H)H:MM PM Classes:
| CLASSID | DAYS | TIME | ...
Where LASTNAME, FIRSTNAME, and STUDENTID are the respective student data. This is followed by the time that the student completed Registration (with the LRM) and left the Registrar. Finally, this will be followed by the class data (CLASSID, DAYS, and TIME) of the classes the student registered for, printed in the order they were originally read in from the file. The print literal used to print the formatted list of registered classes is: "\t| %-8s | %-5s | %-19s | "
Step by Step Solution
There are 3 Steps involved in it
Step: 1
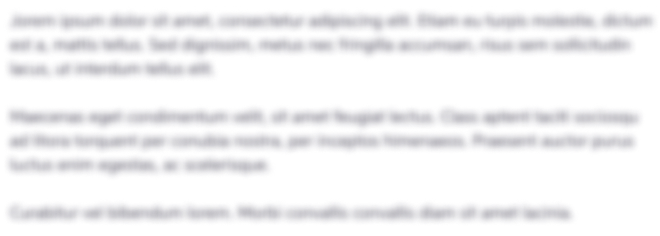
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started