Question
The program below is supposed to ask the user to enter a dollar amount and then to enter with which bills and coins they pay
The program below is supposed to ask the user to enter a dollar amount and then to enter with which bills and coins they pay for the amount. The program then computes the amount to be given as cash back and the bills and coins to be used for that cash back amount.
Complete the program at the places indicated with "TODO".
The only things you need to modify in the template program you are given are concerned with computations. You must not add any print statements nor add any additional Scanner input statements.
The program handles the amounts in cents and not in full dollars. A $1 bill is hence worth 100 cents; a $20 bill is worth 2000 cents.
Suppose you want to compute how many $20 bills to use for a cash-back amount of 4442 cents. That's 2 $20 bills with a remainder of 442 cents, to be returned with other bills and coins, for instance 4 $1 bills, 1 quarter, 1 dime, 1 nickel and 2 pennies.
import java.util.Scanner;
public class Cash {
public static void main(String [] args) { Scanner scnr = new Scanner(System.in); double amountInDollars; int amountInCents; int num20DollarBills; int num10DollarBills; int num5DollarBills; int num1DollarBills; int numQuarters; int numDimes; int numNickels; int numPennies; int amountGivenInCents; int amountReturnInCents; int num20DollarBillsReturn; int num10DollarBillsReturn; int num5DollarBillsReturn; int num1DollarBillsReturn; int numQuartersReturn; int numDimesReturn; int numNickelsReturn; int numPenniesReturn; int amountCashBack;
/* Ask the user to enter an amount in dollars and cents */ System.out.println("Please enter an amount in dollars and cents."); amountInDollars = scnr.nextDouble();
/* Convert dollar amount into amount in cents */ // TODO /* Ask the user to enter the number of bills they want to use */ System.out.println("How many $20 bills do you use to pay the amount?"); num20DollarBills = scnr.nextInt(); System.out.println("How many $10 bills do you use to pay the amount?"); num10DollarBills = scnr.nextInt(); System.out.println("How many $5 bills do you use to pay the amount?"); num5DollarBills = scnr.nextInt(); System.out.println("How many $1 bills do you use to pay the amount?"); num1DollarBills = scnr.nextInt(); System.out.println("How many quarters do you use to pay the amount?"); numQuarters = scnr.nextInt(); System.out.println("How many dimes do you use to pay the amount?"); numDimes = scnr.nextInt(); System.out.println("How many nickels do you use to pay the amount?"); numNickels = scnr.nextInt(); System.out.println("How many pennies bills do you use to pay the amount?"); numPennies = scnr.nextInt();
/* Compute money given */ // TODO
/* Compute amount to return */ amountReturnInCents = amountGivenInCents - amountInCents;
/* Compute number of bills and coins */ amountCashBack = amountReturnInCents; // TODO
/* Display information */ System.out.println("You must pay $" + amountInDollars); System.out.println("You gave $" + (((double) amountGivenInCents) / 100.0)); System.out.println("There is $" + (((double) amountReturnInCents) / 100.0) + " cash back."); System.out.println("You are given " + num20DollarBillsReturn + " $20 bill(s), " + num10DollarBillsReturn + " $10 bill(s), " + num5DollarBillsReturn + " $5 bill(s), " + num1DollarBillsReturn + " $1 bill(s), " + numQuartersReturn + " Quarter(s), " + numDimesReturn + " Dime(s), " + numNickelsReturn + " Nickel(s) and " + numPenniesReturn + " Penny/ies."); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
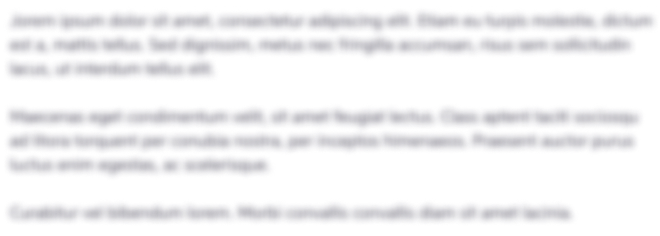
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started