Question
The program generates a sorted list of numbers and calls the binary search function to check if a given value appears in the list Your
The program generates a sorted list of numbers and calls the binary search function to check if a given value appears in the list
Your task is to implement the binary search algorithm in the BinSearch.h file, so that the program produces the expected output. If the number we are looking for appears in the list, then return the position in the list where you found it. If it does not, then return -1.
to test code some expected inputs : 27; should output 26, -1, -1
FASTSEARCHING.CPP FILE:
#include
using namespace std;
int main() { long size; cin >> size; long* numbers = new long[size];
// This list is sorted for (long i = 0; i < size; i++) { numbers[i] = i; } cout << binSearch(numbers, size, size-1) << endl; cout << binSearch(numbers, size, size+5) << endl; cout << binSearch(numbers, size, -17) << endl; return 0; }
Binsearch.h file here :
#ifndef BinSearch_h #define BinSearch_h
long binSearch(long* list, long n, long val){ // Provide your code here }
#endif
Step by Step Solution
There are 3 Steps involved in it
Step: 1
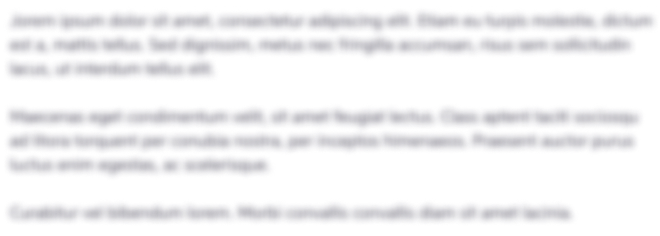
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started