Question
The programing language is Java and mention how to use it on blueJ These are the project codes, Codes for class MailItem /** * A
The programing language is Java and mention how to use it on blueJ
These are the project codes,
Codes for class MailItem
/** * A class to model a simple mail item. The item has sender and recipient * addresses and a message string. * * @author David J. Barnes and Michael Klling * @version 2016.02.29 */ public class MailItem { // The sender of the item. private String from; // The intended recipient. private String to; // The text of the message. private String message;
/** * Create a mail item from sender to the given recipient, * containing the given message. * @param from The sender of this item. * @param to The intended recipient of this item. * @param message The text of the message to be sent. */ public MailItem(String from, String to, String message) { this.from = from; this.to = to; this.message = message; }
/** * @return The sender of this message. */ public String getFrom() { return from; }
/** * @return The intended recipient of this message. */ public String getTo() { return to; }
/** * @return The text of the message. */ public String getMessage() { return message; }
/** * Print this mail message to the text terminal. */ public void print() { System.out.println("From: " + from); System.out.println("To: " + to); System.out.println("Message: " + message); } }
Codes for class MailClient
/** * A class to model a simple email client. The client is run by a * particular user, and sends and retrieves mail via a particular server. * * @author David J. Barnes and Michael Klling * @version 2016.02.29 */ public class MailClient { // The server used for sending and receiving. private MailServer server; // The user running this client. private String user;
/** * Create a mail client run by user and attached to the given server. */ public MailClient(MailServer server, String user) { this.server = server; this.user = user; }
/** * Return the next mail item (if any) for this user. */ public MailItem getNextMailItem() { return server.getNextMailItem(user); }
/** * Print the next mail item (if any) for this user to the text * terminal. */ public void printNextMailItem() { MailItem item = server.getNextMailItem(user); if(item == null) { System.out.println("No new mail."); } else { item.print(); } }
/** * Send the given message to the given recipient via * the attached mail server. * @param to The intended recipient. * @param message The text of the message to be sent. */ public void sendMailItem(String to, String message) { MailItem item = new MailItem(user, to, message); server.post(item); } }
Codes for class MailServer
import java.util.ArrayList; import java.util.List; import java.util.Iterator;
/** * A simple model of a mail server. The server is able to receive * mail items for storage, and deliver them to clients on demand. * * @author David J. Barnes and Michael Klling * @version 2016.02.29 */ public class MailServer { // Storage for the arbitrary number of mail items to be stored // on the server. private List
/** * Construct a mail server. */ public MailServer() { items = new ArrayList(); }
/** * Return how many mail items are waiting for a user. * @param who The user to check for. * @return How many items are waiting. */ public int howManyMailItems(String who) { int count = 0; for(MailItem item : items) { if(item.getTo().equals(who)) { count++; } } return count; }
/** * Return the next mail item for a user or null if there * are none. * @param who The user requesting their next item. * @return The user's next item. */ public MailItem getNextMailItem(String who) { Iterator
/** * Add the given mail item to the message list. * @param item The mail item to be stored on the server. */ public void post(MailItem item) { items.add(item); } }
These are the three class and below is the question
Step by Step Solution
There are 3 Steps involved in it
Step: 1
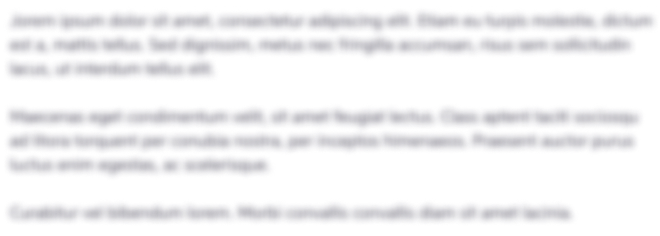
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started