Question
THE PROVIDED FILES ARE BELOW: Customer.cc file: #include using namespace std; #include Customer.h ---------------------------------------------------------------------------------------- Customer.h file: #ifndef CUSTOMER_H #define CUSTOMER_H #include #include Vehicle.h #include VehicleArray.h
THE PROVIDED FILES ARE BELOW:
Customer.cc file:
#include
using namespace std;
#include "Customer.h"
----------------------------------------------------------------------------------------
Customer.h file:
#ifndef CUSTOMER_H
#define CUSTOMER_H
#include
#include "Vehicle.h"
#include "VehicleArray.h"
using namespace std;
class Customer {
public:
Customer(string="", string="", string="", string="");
int getId();
string getFname();
string getLname();
string getAddress();
string getPhoneNumber();
int getNumVehicles();
VehicleArray& getVehicles();
int addVehicle(Vehicle*);
private:
static int nextId;
int id;
string firstName;
string lastName;
string address;
string phoneNumber;
VehicleArray vehicles;
};
#endif
----------------------------------------------------------------------------------------
CustomerArray.cc file:
#include "CustomerArray.h"
#include "Customer.h"
#include "defs.h"
----------------------------------------------------------------------------------------
CustomerArray.h file:
#ifndef CUSTOMERARRAY_H
#define CUSTOMERARRAY_H
#include "Customer.h"
class CustomerArray
{
public:
CustomerArray();
~CustomerArray();
int add(Customer*);
Customer* get(int);
int getSize();
private:
Customer* elements[MAX_CUSTOMERS];
int size;
};
#endif
----------------------------------------------------------------------------------------
defs.h file:
#ifndef DEFS_H
#define DEFS_H
#define MAX_VEHICLES 4
#define MAX_CUSTOMERS 6
#define C_OK 0
#define C_NOK -1
#endif
----------------------------------------------------------------------------------------
main.cc file:
#include "ShopController.h"
int main(int argc, char* argv[])
{
ShopController control;
control.launch();
return 0;
}
----------------------------------------------------------------------------------------
shop.cc file:
#include "Shop.h"
#include "defs.h"
int Shop::addCustomer(Customer* c) { return customers.add(c); }
Customer& Shop::getCustomer(int i) { return *(customers.get(i)); }
CustomerArray& Shop::getCustomers() { return customers; }
-------------------------------------------------------------------------------------------
shop.h file:
#ifndef SHOP_H
#define SHOP_H
#include "Customer.h"
#include "CustomerArray.h"
class Shop{
public:
int addCustomer(Customer*);
Customer& getCustomer(int);
CustomerArray& getCustomers();
private:
CustomerArray customers;
};
#endif
-------------------------------------------------------------------------------------------
ShopController.cc file:
#include "ShopController.h"
ShopController::ShopController() {
initCustomers();
}
void ShopController::launch() {
int choice;
while (1) {
choice = -1;
view.mainMenu(choice);
if (choice == 1) {
view.printCustomers(mechanicShop.getCustomers());
view.pause();
} /*else if (choice == 2) {
} else if (choice == 3) {
} else if (choice == 4) {
} ... */
else {
break;
}
}
}
void ShopController::initCustomers() {
//add data fill here
}
-------------------------------------------------------------------------------------------
ShopController.h file:
#ifndef SHOPCONTROLLER_H
#define SHOPCONTROLLER_H
#include "View.h"
#include "Shop.h"
class ShopController {
public:
ShopController();
void launch();
private:
Shop mechanicShop;
View view;
void initCustomers();
};
#endif
-------------------------------------------------------------------------------------------
Vehicle.cc file:
#include "Vehicle.h"
-------------------------------------------------------------------------------------------
Vehicle.h file:
#ifndef VEHICLE_H
#define VEHICLE_H
#include
using namespace std;
class Vehicle {
public:
Vehicle(string, string, string, int, int);
string getMake();
string getModel();
string getColour();
int getYear();
int getMilage();
private:
string make;
string model;
string colour;
int year;
int mileage;
};
#endif
-------------------------------------------------------------------------------------------
VehicleArray.cc file:
#include "VehicleArray.h"
#include "Vehicle.h"
#include "defs.h"
-------------------------------------------------------------------------------------------
VehicleArray.h file:
#ifndef VEHICLEARRAY_H
#define VEHICLEARRAY_H
#include "defs.h"
#include "Vehicle.h"
class VehicleArray
{
public:
VehicleArray();
~VehicleArray();
int add(Vehicle*);
Vehicle* get(int);
int getSize();
private:
Vehicle* elements[MAX_VEHICLES];
int size;
};
#endif
-------------------------------------------------------------------------------------------
View.cc file:
#include
#include
#include
using namespace std;
#include "View.h"
#include "CustomerArray.h"
#include "Customer.h"
#include "VehicleArray.h"
#include "Vehicle.h"
void View::mainMenu(int& choice) {
string str;
choice = -1;
cout
cout
cout
cout
while (choice 1) {
cout
choice = readInt();
}
if (choice == 0) { cout
}
void View::printCustomers(CustomerArray& arr) {
cout
for (int i = 0; i
Customer* cust = arr.get(i);
ostringstream name;
name getFname() getLname();
cout getId()
getAddress()
getPhoneNumber()
if (cust->getNumVehicles() > 0) {
cout getNumVehicles()
}
VehicleArray& varr = cust->getVehicles();
for (int j = 0; j
Vehicle* v = varr.get(j);
ostringstream make_model;
make_model getMake() getModel();
cout getColour()
getYear()
getMilage()
}
cout
}
}
void View::pause() {
string str;
cout
getline(cin, str);
}
int View::readInt() {
string str;
int num;
getline(cin, str);
stringstream ss(str);
ss >> num;
return num;
}
-------------------------------------------------------------------------------------------
View.h file:
#ifndef VIEW_H
#define VIEW_H
#include "CustomerArray.h"
class View {
public:
void mainMenu(int&);
void printCustomers(CustomerArray&);
void pause();
private:
int readInt();
};
#endif
-------------------------------------------------------------------------------------------
The makefile provided:
OBJ = main.o ShopController.o View.o Shop.o CustomerArray.o VehicleArray.o Customer.o Vehicle.o
mechanicshop: $(OBJ)
g++ -o mechanicshop $(OBJ)
main.o: main.cc
g++ -c main.cc
ShopController.o: ShopController.cc ShopController.h Shop.h View.h
g++ -c ShopController.cc
View.o: View.cc View.h
g++ -c View.cc
Shop.o: Shop.cc Shop.h CustomerArray.h
g++ -c Shop.cc
CustomerArray.o: CustomerArray.cc CustomerArray.h Customer.h defs.h
g++ -c CustomerArray.cc
VehicleArray.o: VehicleArray.cc VehicleArray.h Vehicle.h defs.h
g++ -c VehicleArray.cc
Customer.o: Customer.cc Customer.h
g++ -c Customer.cc
Vehicle.o: Vehicle.cc Vehicle.h
g++ -c Vehicle.cc
clean:
rm -f $(OBJ) mechanicshop
-------------------------------------------------------------------------------------------
Task 1: UML Diagram You will first study the provided code to get an understanding of how all of the classes work together. Based solely on what is provided, draw a UML diagram for this program. Refer to the lecture notes for exactly what is expected to be included and omitted on a UML diagram. This diagram (a scan of a hand drawn diagram is acceptable) will be a part of your assignment submission. Task 2: Finish the Program Your program must use the provided skeleton code without making any changes to the existing code, data structures or function prototypes. You will, however, add your own code to it. Below are the required changes you must make to for your program. 1. Customer and Vehicle classes You are to implement all of the member functions declared in the respective header files for these classes. Some notes on what is expected for the Vehicle class: The constructor simply sets all of the data members to the parameters passed in. The getter functions are simple, one line getters. Some notes on what is expected for the Customer class: The static member nextld is used to give all of the Customer objects unique IDs. Initialize it to 1000 The constructor sets the id member to the current value of the static member nextid, increments nextld and then simply sets all of other data members to the parameters passed in. The getter functions are simple, one line getters. The addVehicle function simply called the add function in the VehicleArray class (described below). It returns what this add function returns. 2. CustomerArray and VehicleArray classes These classes are collection classes that will store multiple Customer and Vehicle objects, respectively. Implementing this in a separate class will allow us to modify the data structure used at a later date without having to make changes to other classes (as we will do on a future assignment). These classes are very similar. Some notes on what is expected for both classes: The constructor simply initializes the size data member properly. The destructor must iterate over the stored objects (which are to be dynamically allocated, discussed below). freeing the allocated memory for each one The getSize function is a simple, one line getter Task 1: UML Diagram You will first study the provided code to get an understanding of how all of the classes work together. Based solely on what is provided, draw a UML diagram for this program. Refer to the lecture notes for exactly what is expected to be included and omitted on a UML diagram. This diagram (a scan of a hand drawn diagram is acceptable) will be a part of your assignment submission. Task 2: Finish the Program Your program must use the provided skeleton code without making any changes to the existing code, data structures or function prototypes. You will, however, add your own code to it. Below are the required changes you must make to for your program. 1. Customer and Vehicle classes You are to implement all of the member functions declared in the respective header files for these classes. Some notes on what is expected for the Vehicle class: The constructor simply sets all of the data members to the parameters passed in. The getter functions are simple, one line getters. Some notes on what is expected for the Customer class: The static member nextld is used to give all of the Customer objects unique IDs. Initialize it to 1000 The constructor sets the id member to the current value of the static member nextid, increments nextld and then simply sets all of other data members to the parameters passed in. The getter functions are simple, one line getters. The addVehicle function simply called the add function in the VehicleArray class (described below). It returns what this add function returns. 2. CustomerArray and VehicleArray classes These classes are collection classes that will store multiple Customer and Vehicle objects, respectively. Implementing this in a separate class will allow us to modify the data structure used at a later date without having to make changes to other classes (as we will do on a future assignment). These classes are very similar. Some notes on what is expected for both classes: The constructor simply initializes the size data member properly. The destructor must iterate over the stored objects (which are to be dynamically allocated, discussed below). freeing the allocated memory for each one The getSize function is a simple, one line getterStep by Step Solution
There are 3 Steps involved in it
Step: 1
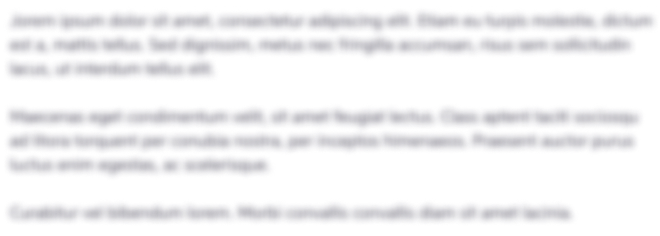
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started