Question
The purpose of the assignment is to finish two Java classes that implement the behavior of 1) a squirrel that is trying to evade terriers,
The purpose of the assignment is to finish two Java classes that implement the behavior of 1) a squirrel that is trying to evade terriers, and 2) a terrier that feels compelled to chase squirrels. The game is played on a board, with some number of terriers and squirrels whose placement is determined by a file. The board is a two-dimensional array, with 'S' for Squirrel, 'D' for Terrier, 'T' for Tree, 'F' for Fence, and '-' for empty squares with Grass.
The game engine reads the file specified on the command line, builds a user interface. Approximately every couple of seconds it asks each squirrel and terrier which way they want to move. You are going to code the behavior of both animals.
Terrier behavior is defined in Terrier.java. Terriers always try to chase after the nearest squirrel and eat it. Terriers must avoid running into other terriers or going off the board, and they cannot climb trees or pass through Fences.
Squirrel behavior is defined in Squirrel.java. Squirrels look for the closest terrier and always move in the opposite direction. Squirrel must avoid running into other squirrels or terriers, but they can pass through fences or climb trees. If they make it to a Tree, they are safe and disappear from the board.
The game continues until all squirrels are safe or eaten, or for 30 iterations, whichever comes first. None of the supplied fields require that many iterations. The game engine reports all movements and significant events.
Write the findClosest method to find which Terrier is the closest. To do so you must scan the board from top to bottom and left to right, computing the Euclidean distance to each Terrier that you find. The Euclidean distance calculation is shown here.
Store the row and column of the closest terrier so that you can return it when getClosestRow and getClosestCol are called.
If more than one Terriers are at an equal distance, store the row and column for the first Terrier you find.
Copy the current position to the previous position.
Here is the code I have so far:
import java.io.File;
import java.io.FileNotFoundException;
import java.lang.Math;
import java.util.Scanner;
public class Squirrel implements AnimalInterface {
//
// DO NOT MODIFY BELOW
//
private int currentRow;
private int currentCol;
private int previousRow = -1;
private int previousCol = -1;
private int closestRow;
private int closestCol;
private char[][] field;
// Initializes position and field
public Squirrel(int row, int col, char[][] field){
this.currentRow = row;
this.currentCol = col;
this.field = field;
}
// Getters
public int getCurrentRow(){ return currentRow; }
public int getCurrentCol(){ return currentCol; }
public int getPreviousRow(){ return previousRow; }
public int getPreviousCol(){ return previousCol; }
public int getClosestRow(){ return closestRow; }
public int getClosestCol(){ return closestCol; }
//
// DO NOT MODIFY ABOVE
//
// Find closest terrier
public void findClosest(){
int rows = 0; int col = 0;
rows = field[0][0]; col = field[1][0];
// TO DO: Replace with code to find closest
closestRow = -1;
closestCol = -1;
}
// Move squirrel according to the rules
public void moveAnimal() {
eMove move;
// Store previous position
previousRow = currentRow;
previousCol = currentCol;
// TO DO: replace with code to select move (Step 1)
move = eMove.RIGHT;
// TO DO: replace with code to adjust move (Step 2)
move = move;
// TO DO: replace with code to make move (Step 3)
currentCol++;
}
//
// Private Methods, if you need them
//
}
Terrier.java code:
import java.lang.Math;
public class Terrier implements AnimalInterface {
//
// DO NOT MODIFY BELOW
//
private int currentRow;
private int currentCol;
private int previousRow = -1;
private int previousCol = -1;
private int closestRow;
private int closestCol;
private char[][] field;
// Initializes position and field
public Terrier(int row, int col, char[][] field){
this.currentRow = row;
this.currentCol = col;
this.field = field;
}
// Getters
public int getCurrentRow(){ return currentRow; }
public int getCurrentCol(){ return currentCol; }
public int getPreviousRow(){ return previousRow; }
public int getPreviousCol(){ return previousCol; }
public int getClosestRow(){ return closestRow; }
public int getClosestCol(){ return closestCol; }
//
// DO NOT MODIFY ABOVE
//
// Find closest squirrel
public void findClosest(){
// TO DO: Replace with code to find closest
closestRow = -1;
closestCol = -1;
}
// Move terrier according to the rules
public void moveAnimal() {
eMove move;
// Store previous position
previousRow = currentRow;
previousCol = currentCol;
// TO DO: replace with code to select move (Step 1)
move = eMove.LEFT;
// TO DO: replace with code to adjust move (Step 2)
move = move;
// TO DO: replace with code to make move (Step 3)
currentCol--;
}
//
// Private Methods, if you need them
//
}
Step by Step Solution
3.42 Rating (158 Votes )
There are 3 Steps involved in it
Step: 1
For Squirrel Terrier same logic code applies Here I am finding the distance of the current location ...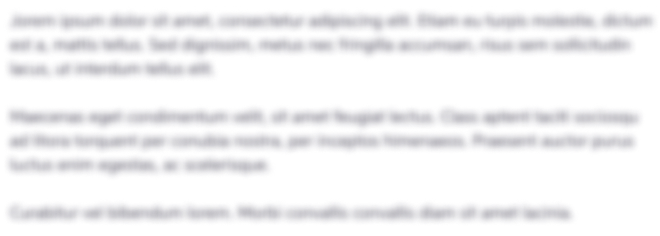
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started