Question
The purpose of this assignment is to build a new php web application form that will connect to the MySQL database hosted on the cloud
The purpose of this assignment is to build a new php web application form that will connect to the MySQL database hosted on the cloud system. This form will do many of the basic web application form activities such as insert and read data from a database. A web page does not always have to perform database activity. If you need a web page the can send out a message and that is it's only purpose then you have met your purpose. In this course, we will build more than just a simple web page with a message.
NOTE - Each of the following lab objectives has individual lab appendix references.
Create a new Word lab document for this week's lab objectives then take screenshots of all your work and paste into the document for submission.
Lab Objectives Checklist
Create a new web page file.
Write basic HTML5 tags to build the web page.
Build an HTML5 web page form.
Get all of the connection string details from the database service.
Write php code to build the connection to the database service.
Write php code to insert new records into the database.
Write php code to get records and display back into the web page.
Write CSS3 to format the web page.
Submit the snapshots of your work to the course Dropbox for grading.
Objective #1 Create a New PhP Web File
In this task your will begin by making a simple file on your desktop. Then rename the file to "index.php", which can get a little tricky if your operating system does not show file extensions. You might need to go into your Control Panel and select the File Explorer Options icon then change the folder options to show file extensions. Look at the View tab and the screenshot below and make sure you have the same options enabled. Once you are able to see the file type, make certain the file name is correct such as "index.php".
For help with the following lab objectives refer to Lab Appendix D.
Image #1 Control Panel File Explorer Options View of Files and Folders
Next, add the HTML5 code below and make the file compatible with web hosting servers so browsers can view the page then save the file.
Objective #2 Build a PhP Web Form
The purpose of this objective is to build a web page with HTML and then start adding php. Once the "index.php" file is created on your desktop then open it in Notepad.exe or you can use any editor that works best for you. Enhance the HTML code and build a simple web form within the
html tags.For help with the following lab objectives refer to Lab Appendix D.
Rasmussen Registration Form
Fill in your name and telephone, then click Submit to register.
Objective #3 Build a PhP Connection String
In this objective, you will create a new connection string to the cloud MySQL database service and there are several pieces of information that you will need to get from the database service. Open the "index.php" file on your desktop into Notepad.exe or you can use any editor that works best for you.
Go to the student cloud portal and select the MySQL database service and get the database service URL which is the $host variable value. In the database service properties get the database user identification, which is the $user variable value. The database service password was created while building the database service and if you do not remember this password, it will need reset then assign a copy in of the password to the $pwd variable. Finally, copy and paste the php connection string code below into the php tags within your file.
For help with the following lab objective refer to Lab Appendix E.
Objective #4 Write PhP and Process Database Activity
In this objective, you will continue building on the php code so the application will connect to the database service and do some basic database activity like running sql commands. Open the "index.php" file on your desktop into Notepad.exe or you can use any editor that works best for you.
There are three blocks of php code the application to the database service. The connection will only occur when the application is hosted on a php server. If you try to view the web page in a browser, expect to see many errors. Copy and paste the php "Connect to Database" code below into the php tags within your file but stay below the connection string. You want the connection string to information populated first before the application tries to connect to the database.
For help with the following lab objective refer to Lab Appendix F.
// Connect to Database. try { $conn = new PDO( "mysql:host=$host;dbname=$db", $user, $pwd); //$conn->setAttribute( PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION ); } catch(Exception $e){ die(var_dump($e)); }
Copy and paste the PHP syntax to insert a new record
//Following the database connection code, add code for inserting registration information into the database. if(!empty($_POST)) { try { $first_name = $_POST['first_name']; $last_name = $_POST['last_name']; $telephone = $_POST['telephone']; // Insert data $sql_insert = "INSERT INTO tblCDA3315C(FIRST_NAME, LAST_NAME, TELEPHONE) VALUES (?,?,?)"; $stmt = $conn->prepare($sql_insert); $stmt->bindValue(1, $first_name); $stmt->bindValue(2, $last_name); $stmt->bindValue(3, $telephone); $stmt->execute(); } catch(Exception $e) { die(var_dump($e)); } echo "
You're Registered!
"; }
//Finally, following the code above, add code for retrieving data from the database. $sql_select = "SELECT * FROM tblCDA3315C "; $stmt = $conn->query($sql_select); $registrants = $stmt->fetchAll(); if(count($registrants) > 0) { echo "
People Who Are Registered:
"; echo "FIRST_NAME | "; echo "LAST_NAME | "; echo "TELEPHONE |
---|---|---|
".$registrant['FIRST_NAME']." | "; echo "".$registrant['LAST_NAME']." | "; echo "".$registrant['TELEPHONE']." |
No one is currently registered.
"; }Copy and paste the PHP syntax to retrieve data from the database service
Preview the sample code in the Google Chrome browser
At this point, you should expect several errors since the browser is trying to connect to the database service
This is normal and for now, try to ignore the errors
Objective #5 Format the HTML5 with CSS3
Cascaded Style Sheet (CSS) is a language that works with HTML tags to display the elements on the screen. A developer can use CSS in many ways to visually enhance the HTML elements while giving the user a seamless experience. Work through each step below to build and integrate CSS into a php web site file.
For help with the following lab objective refer to Lab Appendix G.
.wrapper { overflow: hidden; }
Write a new [[Script]] tag in the index.php file
Inside the [[head]] tag create a new open/closing set of script tags (e.g. [[style type="text/css"]] [[/script]])
Expand the script tags so there are empty lines between them
Copy and paste the CSS wrapper class syntax between the script tags
Add a new wrapper
This tag will wrap around all of the other
Copy and paste the CSS syntax to format support for different device screen sizes
Make sure this CSS syntax is between the [[script]] tags
/*PHONE CSS */ @media screen and (min-width: 10px) and (max-width: 550px), (min-device-width: 10px) and (max-device-width: 550px) { #Div1, #Div2, #Div3, #tblGrid { border:2px solid black; width:100%; float:left; font-size: 2em; } #first_name, #last_name, #telephone { width: 100%; border: 2px solid black; } #submit1 { background-color: #ffff80; } }
/*TABLET CSS */ @media screen and (min-width: 551px) and (max-width: 900px), (min-device-width: 551px) and (max-device-width: 900px) { #Div1, #Div2, #Div3 { border:2px solid black; width:100%; float:left; font-size: 4em; } #first_name, #last_name, #telephone { width: 100%; border: 2px solid black; } #submit1 { background-color: #ccb3ff; } }
/*FULL SCREEN CSS */ @media screen and (min-width: 901px), (min-device-width: 901px){
body { background-color: #fff; border-top: solid 10px #000; color: #333; font-size: .85em; margin: 20; padding: 20; font-family: "Segoe UI", Verdana, Helvetica, Sans-Serif; } h1, h2, h3,{ color: #000; margin-bottom: 0; padding-bottom: 0; } h1 { font-size: 2em; } h2 { font-size: 1.75em; } h3 { font-size: 1.2em; } table { margin-top: 0.75em; boarder: 2px; } th { font-size: 1.2em; text-align: left; border: none; padding-left: 0; } td { padding: 0.25em 2em 0.25em 0em; border: 0 none; } #Div2, #Div3 { border:2px solid black; float:left; font-size: 4em; } #Div1 { border:2px solid black; float:left; font-size: 4em; width:100%; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
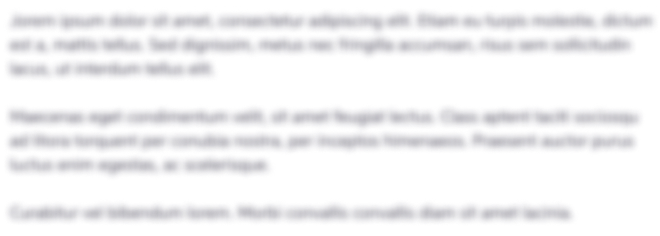
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started