Question
The purpose of this assignment is to exercise operator overloading: To do this assignment, you must extend the code you have developed for PA2 to
The purpose of this assignment is to exercise operator overloading:
To do this assignment, you must extend the code you have developed for PA2 to implement the following:
Add two new member functions non-member operator overloading and member operator overloading for Rectangle class only+ : (union of two rectangles) for two specified rectangle objects, take a union of both rectangles and return the area of the union of two rectangles.
If there is an intersection between two rectangles, it returns the sum of the areas of both rectangles subtracted by the intersected area.
If there is no intersection between two rectangles, it simply return the sum of the areas of both rectangles.
* : (intersection of two rectangles) for two specified rectangle objects, take an intersection of both rectangles and return the area of the intersection of two rectangles.
If there is an intersection between two rectangles, it returns the intersection of the areas of two rectangles.
If there is no intersection between two rectangles, it simply return 0.
Implement both non-member operator overloading and member operator overloading for the following operators for all the classes whenever applicable
<< : a.k.a. insertion operator to draw the shape of each concrete class of Shape class. Please note that you should implement both non-member and member operator.
Add a main driver to test:4 intersection test cases:
Intersected and member operator overloading
Not ntersected and member operator overloading
Intersected and non-member operator overloading
Not ntersected and non-member operator overloading
4 union test cases:
Intersected and member operator overloading
Not ntersected and member operator overloading
Intersected and non-member operator overloading
Not ntersected and non-member operator overloading
Drawing two house pictures from PA2 using "<<" operator, one with member operator overloading and the other with non-member operator overloading
Deliverable:
A new .h and .cpp files for each shape class (total at least 9 files, 4 .h + 4 .cpp + main driver) in a zip file and those 9 files in plain old text file format (Total 10 source files)
A makefile (Must, no cmake please!!)
Output in a text file format (no .jpg picture will be acceptable) - please use UNIX file redirection to draw them into a file.
HERE IS THE CODE FROM PA2:
Circle.cpp
#include
#include "circle.h"
#include "shapes.h"
#include
using namespace std;
Circle::Circle()
{
radius=0;
}
void Circle:: setRadius(int r) {radius=r;}
void Circle:: draw() {
int circle_radius = 3; //
for (int i = 0; i <= 2 * circle_radius; i++) {
for (int j = 0; j <= 2 * circle_radius; j++) {
float distance_to_centre = sqrt(
(i - circle_radius) * (i - circle_radius) + (j - circle_radius) * (j - circle_radius));
if (distance_to_centre > circle_radius - 0.5 && distance_to_centre < circle_radius + 0.5) {
cout << "*";
} else {
cout << " ";
}
}
cout << endl;
}
}
double Circle:: computeArea(){
double area =(3.14159*(radius*(radius)));
}
Circle.h
#ifndef OOHW2_CIRCLE_H
#define OOHW2_CIRCLE_H
#include
#include "shapes.h"
class Circle: public shapes {
public:
Circle();
void setRadius(int r);
void computeArea();
void draw();
private:
int radius;
};
#endif //OOHW2_CIRCLE_H
point.cpp
#include
#include "Point.h"
using namespace std;
Point::Point()
{
x=0;
y=0;
}
Point.h
#ifndef POINT_H
#define POINT_H
#include
class Point {
public:
Point();// default constructor
int x,y;
};
#endif
rectangle.cpp
#include
#include "rectangle.h"
#include "shapes.h"
using namespace std;
Rectangle::Rectangle() {
width=0;
height=0;
}
void Rectangle:: setHeight(int h) {height=h;}
void Rectangle :: setBase(int b) {width=b;}
void Rectangle :: draw() {
for (int i = 0; i < width; i++) {
cout << "* ";
}
cout << endl;
for (int j = 0; j < height; j++)
{
cout<<"* ";
for(int k=0;k<(width+(width-3));k++)
{
cout << " ";
}
cout <<"* ";
cout < } for(int i=0;i { cout<<"* "; } cout< } void Rectangle:: computeArea() { double area = height * width; } Rectangle.h #ifndef RECTANGLE_H #define RECTANGLE_H #include #include "shapes.h" class Rectangle: public shapes { public: Rectangle(); //default constructor void setHeight(int height); void setBase(int base); double computeArea(); void draw(); private: int width; int height; }; #endif shapes.cpp #include "shapes.h" #include using namespace std; shapes::shapes() { area=0.0; circumference=0.0; } //coordinates int shapes::getX() {return Point::x;} int shapes::getY() {return Point::y;} void shapes::setX(int newx) {Point::x=newx;} void shapes::setY(int newy) {Point::y=newy;} void shapes::draw(){} //area double shapes::getArea() {return area;} void shapes:: setArea(double a) {area=a;} //circumference double shapes:: getCircumference() {return circumference;} void shapes:: setCircumference(double c) {circumference=c;} //methods void shapes:: computeArea() {} void shapes:: computeCircumference() {} void shapes:: setLineType(char lineType) {lineTY=lineType;} void shapes::moveBy(int space) {} void shapes:: draw(int length) {} void shapes:: draw(int width, int height) {} shapes.h #ifndef SHAPES_H #define SHAPES_H #include #include "Point.h" class shapes : public Point{ public: //default constructor shapes(); //Methods virtual double computeArea(); virtual double computeCircumference(); virtual void moveBy(int space); virtual void draw(); virtual void draw (int length); virtual void draw(int width, int height); //setter and getters void setX(int newx); void setY(int newy); void setArea(double a); void setCircumference(double c); void setLineType(char lineType); double getArea(); double getCircumference(); int getX(); int getY(); private: char lineTY; double area; double circumference; }; #endif square.cpp #include "square.h" #include "rectangle.h" #include using namespace std; Square::Square() { } void Square::setSide(int side) { setBase(side); setHeight(side); } square.h #ifndef SQUARE_H #define SQUARE_H #include #include "rectangle.h" class Square: public Rectangle{ public: Square(); void setSide(int side); }; #endif triangle.cpp #include "triangle.h" #include "shapes.h" #include using namespace std; Triangle::Triangle() { base = 0; height = 0; } void Triangle :: setHeight(int h) {height=h;} void Triangle :: setBase(int b) {base=b;} void Triangle:: draw() { int spaces=base/2; cout << " "; for(int i=0;i { cout<< " "; } cout<<"*"< cout<< " "; for(int i=0;i<(spaces/2)+1;i++) { cout << " "; } cout<<"*"; for(int i=0;i<(spaces/2)+1;i++) { cout << " "; } cout<<"*"< cout << " "; for(int i=0;i<(spaces/2)+1;i++) { cout << " "; } cout<<"*"; for(int i=0;i<(spaces/2)+(spaces/2)+1;i++) { cout<<" "; } cout<<"*"< cout<< " "; cout << "*"; for(int i=0;i { cout << "*"; } } double Triangle :: computeArea() { double area= (base*height)/2.0; } triangle.h #ifndef OOHW2_TRIANGLE_H #define OOHW2_TRIANGLE_H #include "shapes.h" #include class Triangle: public shapes { public: Triangle();//default constructor void setHeight(int h); void setBase(int b); void draw(); void computeArea(); private: int base; int height; }; #endif //OOHW2_TRIANGLE_H ABOVE IS MY CODE FROM THE LAST PA2!!! PLEASE MAKE SURE ITS IN C++, YOU READ THE PROBLEM, AND KNOW WHAT TO DELIVER!! PLEASE I DON'T KNOW WHERE TO START WITH THIS PROBLEM!!! PLEASE ANSWER IT ASAP!! AND YOU CAN CHANGE MY CODE FROM MY PA2 IN ORDER TO MAKE THE PROGRAM FUNCTION!! WHOEVER ANSWERS THIS THANK YOU SOOO MUCH!!!
Step by Step Solution
There are 3 Steps involved in it
Step: 1
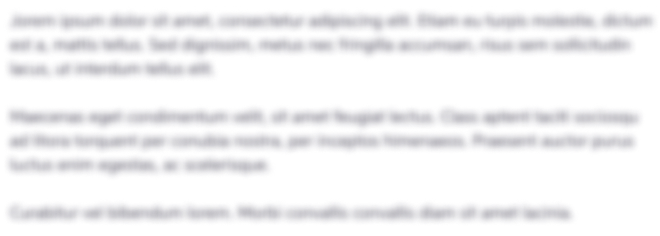
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started