Question
The purpose of this assignment is to implement a simple typing lesson game. The computer will generate a random string of 5 characters in length
The purpose of this assignment is to implement a simple typing lesson game. The computer will generate a random string of 5 characters in length and you need to reproduce it within 10 seconds. You will lose points significantly if you fail to produce the same string within the set time interval.
You have 2000 points in the beginning of the game. Given 5 randomly generated letters being mixed with lower and upper cases, you need to type them correctly within 10 seconds (10000 msec). You will get 500 points every time you produce the matching string within the interval. If you type a correct response, but you take longer than the time limit, you will lose an amount equal to the time overage in millisecond, e.g., your typing ended in 10350 msec you would lose 350 points.
If you misspell, you will be penalized by the total offset of mistaken letters. You will lose this offset from your points. The total offset is computed by accumulating the absolute distance between two characters in the same position, one from the generated string and another from the input. For instance, the offset of "Game" and "Mag" is 81. The shorter string is padded with space(s). Therefore, |G - M| = 6, |a - a| = 0, |m - g| = 6, |e - (space)| = 69. In other words, the total offset is the sum of the ASCII code differences between the ith character in the generated string and the ith character of user input.
You should print the offset if it is different from 0. Do not print the offset if the user had correct input, regardless if the time limit was exceeded.
If you misspell and overtime, you will be penalized the double score of the offset, plus the time overage. The game ends if you reach score 5000 or 0.
An alphabetic character (a letter) is generated 80% of the time. But the generated string may contain wild letters. For each character position in the generated string there is a 20% probability of getting a wild card character. There are two types of wild card characters, shown to the user as [0-9] and [%-!]. [0-9] is used to represent any single digit, and [%-!] is used to represent any non-alphanumeric character. That 20% probability should be divided in two for the two categories of wild card characters,
In other words:
The computer will generate a string of 5 characters. 20% of the time a wild card character will be generated. Half of that time it should be a NON Alphanumeric character. In the prompt to the user this is shown as [%-!]. If the user enters ANY non-alphanumeric character it is considered a match. For this situation, you should add a "*" to your growing string.
The other half of time the wildcard character should be a single digit. This is shown to the user as [0-9] For this situation, you should add a "0" to your growing string.
As for alphabetic characters (not wild), half of the time it should be an UPPER case letter and half of the time it should be a lower case letter
When the following is printed by the program: BIy[0-9][%-!] the user should try to match the 5 character string having the following specification:
The user is to try to type:
the first character is B
the second character is I the third character is y
the fourth character is any single digit
the fifth character is any NON alphanumeric
Typing error for positions with wild card characters is treated according to the following: If [0-9] appears in the output, and the user does not type a digit, the offset for that position should be the absolute difference between the character typed and 0.
If [%-!] appears in the output, and the user does not type a non-alphanumeric character, the offset for that position should be the absolute difference between the character typed and *. For instance, the offset of Tw[0-9]v[%-!] and Twxv2 is 80. From the ASCII chart we have the following decimal codes: 0 has a code of 48 * has a code of 42 x has a code of 120 2 has a code of 50 We get 80 because of the mismatch at 3rd and 5th character. The absolute difference for the 3rd character is abs(120 48) == 72 and for the 5th character is abs(50 42) == 8. Therefore the offset is 72+8 resulting in 80.
There were a few ways to approximate elapsed time. The new C++ 2011 standard has a good way to do it by including
to realize it.
What follows is a sample game session: Your current points 2000, just type -> N[%-!]sVc: N.sVc 6818 milliseconds, you made it within the interval of 10000... Your current points 2500, just type -> U[0-9][0-9]C[%-!]: u00C. 8406 milliseconds, you made it within the interval of 10000... String offset is 32, your total penalty is 32... Your current points 2468, just type -> e[%-!]vNT: e.uNS 9829 milliseconds, you made it within the interval of 10000... String offset is 2, your total penalty is 2... Your current points 2466, just type -> tXr[0-9]x: tXr0x 8920 milliseconds, you made it within the interval of 10000... Your current points 2966, just type -> BIy[0-9][%-!]: BIy0! 10153 milliseconds, you *failed* it within the interval of 10000... Your current points 2813, just type -> rHcmK: rHcmK 12103 milliseconds, you *failed* it within the interval of 10000... Your current points 710, just type -> kO[0-9]ed: kO9ed 14727 milliseconds, you *failed* it within the interval of 10000... Bye...
Use isdigit() and isalnum() utility functions wisely.
Since we have started discussing functions, I would like to see you implement and use some functions.Including one that generates the string.
A possible prototype: string generate(const int length); // generating a random string
A function to compute the sum of absolute value of differences of i-th byte in generated and userInput. Returning a 0, would indicate a match: int offset(const string generated, const string userInput);
Note:Soon we will talk more about functions, and a better implementation would be: int offset(const string& generated, const string& userInput);
Those &'s after the data-type will be explained soon.
Hints: It might be helpful to think of maintaining three views: 1. The one output to the user that could contain upper and lower case letters, [0-9] to represent any digit and/or[%-!] to represent a non-alphanumeric character. 2. The randomly generated string containing characters that could include upper and lower letters, 0 to represent any digit and * to represent a non-alphanumeric character. 3. The string entered by the user with each round.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
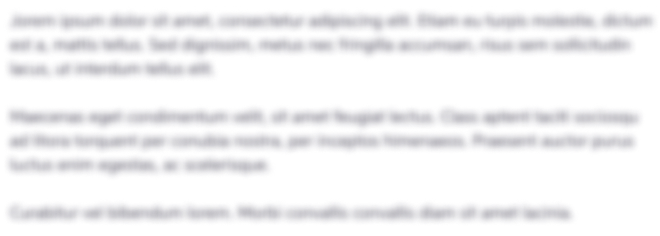
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started